How to Log SQL Queries in Laravel
Hello Artisans, in this tutorial we will discuss how to Log SQL queries in Laravel. SQL log is the most common way to debug any application. So, let’s get dive into the topic.
Note: Tested on Laravel 8.65.
Table of Contents
Using Default Log fi
Laravel default log file will be stored in storage/logs. To log queries in this file we have to add the following code snippet in AppServiceProvider.php file in the boot() method. So paste the below code
app/Providers/AppServiceProvider.php
<?php
namespace App\Providers;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\Log;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
//
}
public function boot()
{
DB::listen(function($query) {
Log::info(
$query->sql,
$query->bindings
);
});
}
}
Now go to storage/logs and open laravel.log file, you will see like about below output
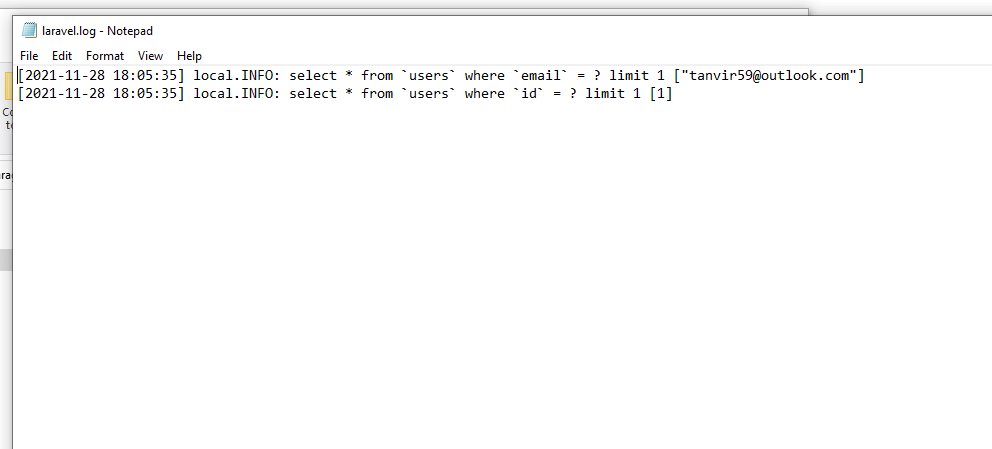
Using Default Log fi
Another option is to log into a separate query.log file. So, replace your AppServiceProvider.php file with the below code:
app/Providers/AppServiceProvider.php
<?php
namespace App\Providers;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\File;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
//
}
public function boot()
{
DB::listen(function($query) {
File::append(
storage_path('/logs/query.log'),
'[' . date('Y-m-d H:i:s') . ']' . PHP_EOL . $query->sql . ' [' . implode(', ', $query->bindings) . ']' . PHP_EOL . PHP_EOL
);
});
}
}
Now go to storage/logs and open query.log file, you will see like about below output
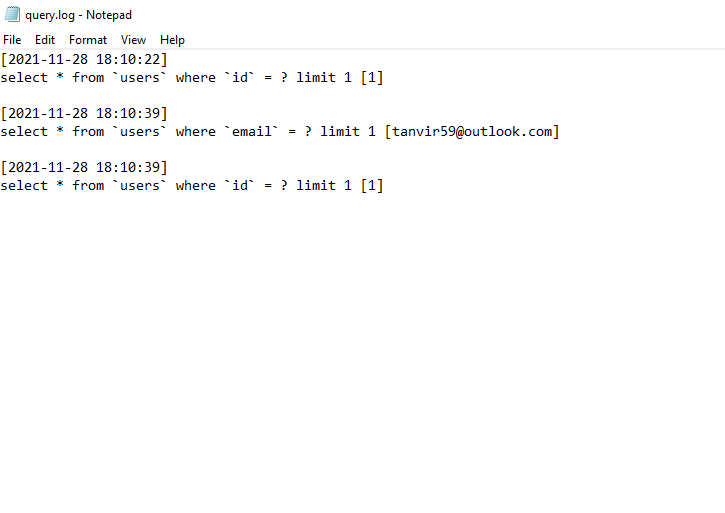
That’s all for today. Thanks for reading.