Laravel 10 Create Zip File and Download
Hello Artisan, today I'll show you How to Create Zip File and Download in our Laravel application. This article provides a comprehensive guide on creating a zip file in Laravel 10. If you're looking for a practical example of how to create and download a zip file in Laravel 10 from a specific folder, you've come to the right place. Let's demonstrate how to achieve this.
A zip file is like a digital container that helps make big data smaller and more organized. It compresses files and folders into a single archive, making them take up less space without losing any content. This way, you can easily share or store them, especially when dealing with large files over the internet.
In this post, I'll demonstrate two methods to generate a zip file from a folder in Laravel. The first approach utilizes the ZipArchive class, while the second one employs the "stechstudio/laravel-zipstream" package. We'll begin by creating a folder called "myFiles" and then proceed with creating a zip file from the contents of that folder. Now, let's explore both examples step by step.
Example 1:
Example 2:
- Install Laravel
- Install stechstudio/laravel-zipstream Package
- Create Controller
- Create Route
- Run Laravel App
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project laravel/laravel Zip-File
Let's create ZipController by following command:
php artisan make:controller ZipController
Here we will add one new method for route. __invoke() will generate new zip file and download as response, so let's add bellow
<?php
namespace App\Http\Controllers;
use File;
use ZipArchive;
class ZipController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function __invoke()
{
$zip = new ZipArchive;
$fileName = 'myNewFile.zip';
if ($zip->open(public_path($fileName), ZipArchive::CREATE) === TRUE)
{
$files = File::files(public_path('myFiles'));
foreach ($files as $key => $value) {
$relativeNameInZipFile = basename($value);
$zip->addFile($value, $relativeNameInZipFile);
}
$zip->close();
}
return response()->download(public_path($fileName));
}
}
In this is step we need to create route for create and download zip file. so open your "routes/web.php" file and add following route.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ZipController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('download-zip', ZipController::class);
Ok now you can run project and open that route like.
Make sure you have a folder named "myFiles" in the public directory. Add some PDF files to that folder, and the script will create a zip file containing those files
To run the Laravel app, simply type the following command and press Enter:
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
http://localhost:8000/download-zip
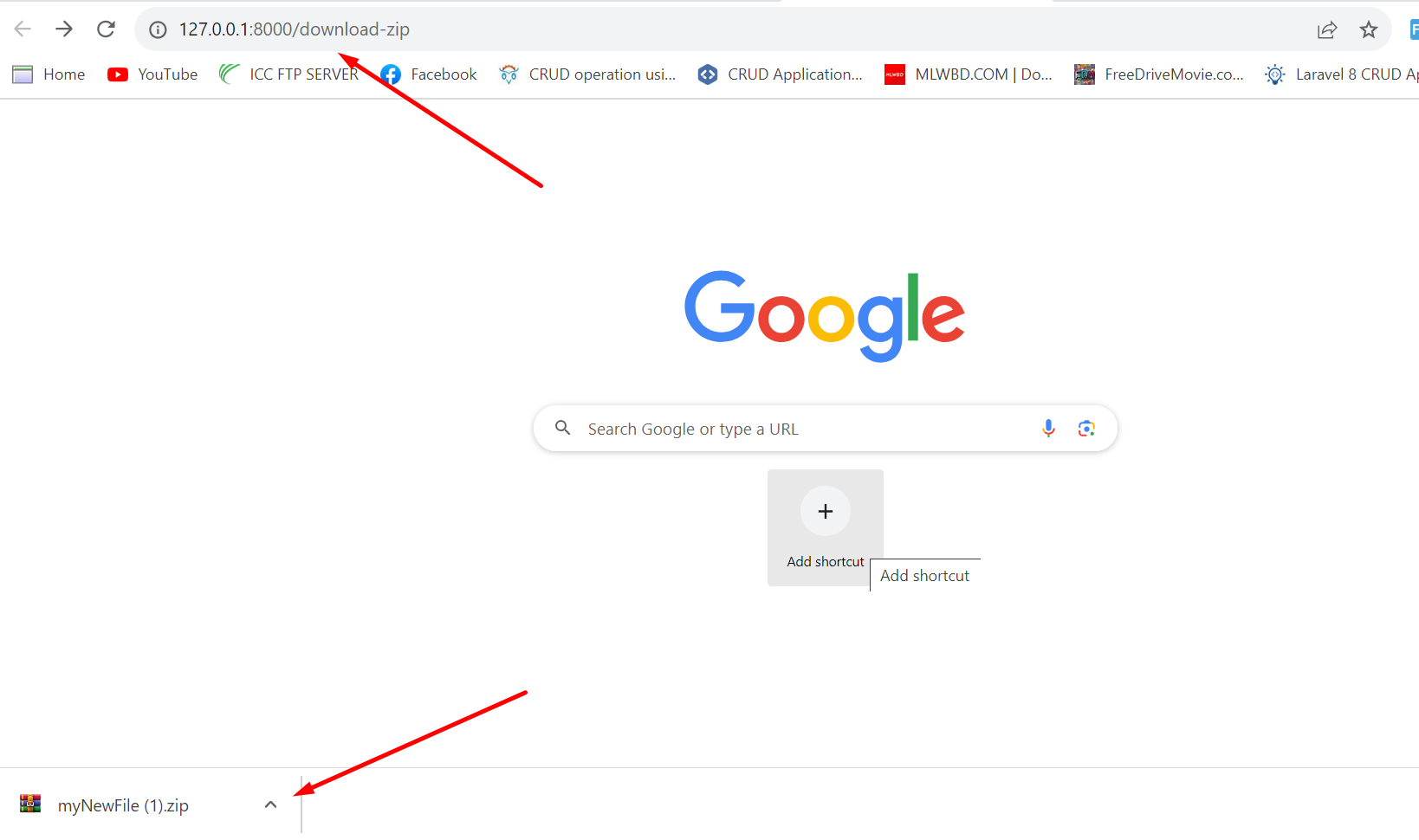
Example 2:
Let's see this example using stechstudio/laravel-zipstream composer package.
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project laravel/laravel Zip-File
In this step, we will install stechstudio/laravel-zipstream composer package using the following command:
composer require stechstudio/laravel-zipstream
Let's create ZipController by following command:
php artisan make:controller ZipController
Here we will add one new method for route. __invoke() will generate new zip file and download as response, so let's add bellow
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use File;
use Zip;
class ZipController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function __invoke()
{
return Zip::create('zipFileName.zip', File::files(public_path('myFiles')));
}
}
In this is step we need to create route for create and download zip file. so open your "routes/web.php" file and add following route.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ZipController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('download-zip', ZipController::class);
Ok now you can run project and open that route like.
Make sure you have a folder named "myFiles" in the public directory. Add some PDF files to that folder, and the script will create a zip file containing those files
To run the Laravel app, simply type the following command and press Enter:
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
http://localhost:8000/download-zip
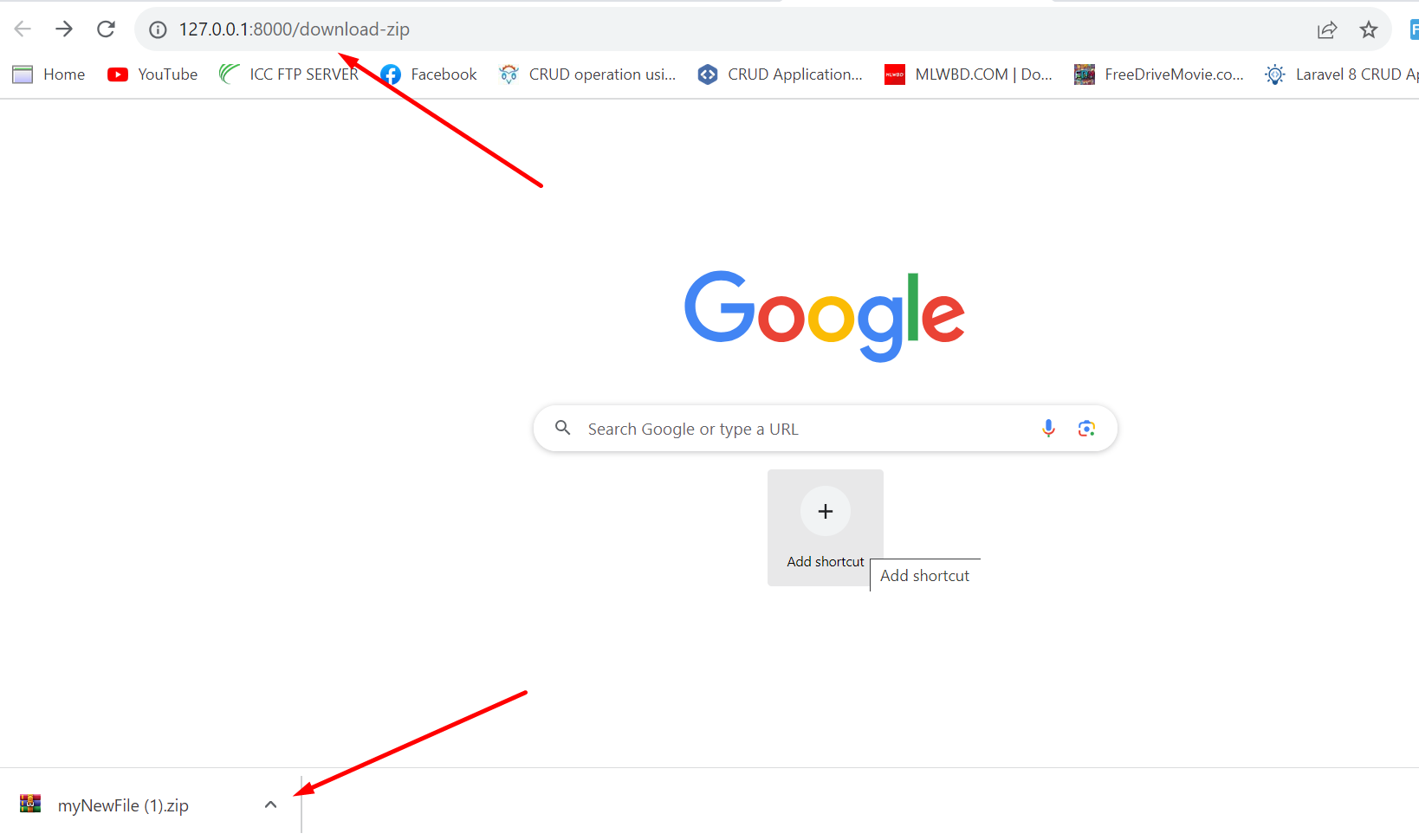
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂