Laravel Restrict User Access from Specific IP Address
Hello Artisans, today I'll show you how to restrict list of IP address form accessing your laravel application. For achieving our expected result, we just need to complete 2 steps. So, let's see how we can easily integrate an IP address blocker in our laravel application.
Note: Tested on Laravel 9.19
First of all, we'll create a middleware so that we can define some IP's which we want to block and based on that we can restrict user or can give them access to our application. So, fire the below commands in the terminal to create a middleware.
php artisan make:middleware RestrictIpMiddleware
It'll create a middleware under app\Http\Middleware called RestrictIpMiddleware.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Mail;
class SenMailController extends Controller
{
public $block_ips = ['blacklist-ip-1', 'blacklist-ip-2', '127.0.0.1'];
public function handle(Request $request, Closure $next)
{
if (in_array($request->ip(), $this->block_ips)) {
return abort(403);
}
return $next($request);
}
}
Now we need to register the middleware. Open kernel.php update the $routeMiddlleware array as following
protected $routeMiddleware = [
.....
'blockIp' => RestrictIpMiddleware::class
];
And our middleware is successfully registered. Now, if any user wants to access in our application it'll check, if the current IP is blacklisted or not.
Now we'll create routes so that we can access in our view file. So, put the below route in web.php file.
<?php
use Illuminate\Support\Facades\Route;
Route::middleware('blockIp')->get('/', function () {
return view('welcome');
});
And finally we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well, we can find a below output.
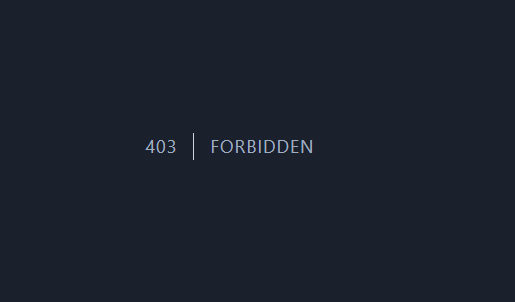
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐