Laravel Remove Quotes/Any Character from String
Hello Artisans, today I'll show you how to remove quotes from string in your laravel application. From todays tutorial we cannot only remove quotes from string but also can remove any characters we want. So, let's get started and see how we can easily remove quotes/ special character from any string in our laravel application.
Note: Tested on Laravel 9.19
First of all, create a controller so that we can remove quotes (or any string we want) from the string . So, fire the below commands in the terminal.
php artisan make:controller HomeController
It'll create a controller under app\Http\Controllers called HomeController.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function removeWords()
{
$str = 'Welcome to "shouts.dev"';
dd(str_replace('"', '', $str));
}
}
Now we'll create routes so that we can see the output of our string after removing the quotes. So, put the below route in web.php file.
<?php
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('welcome');
});
Route::get('remove/words', [\App\Http\Controllers\HomeController::class,'removeWords']);
And finally we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well (hope so) you'll find a below output
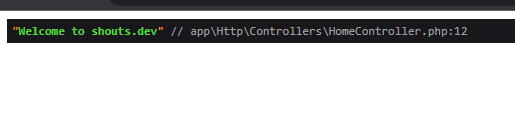
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐