How to Generate XML Sitemap Without Using Package
In this article, I’m going to share how to create an XML sitemap manually. Let’s assume that we have a model named Article for table articles. We’re going to generate sitemap for the articles table. Let’s get started:
Table of Contents
Create a Controller
I’m creating a controller called SitemapController
. Run this command to create the controller:
php artisan make:controller SitemapController
After creating the controller, open it and paste the below code:
<?php
namespace App\Http\Controllers;
use App\Article;
use Illuminate\Http\Request;
class SitemapController extends Controller
{
public function articles()
{
$articles = Article::all();
return response()->view('sitemap-articles', compact('articles'))->header('Content-Type', 'text/xml');
}
}
Create a View
Go to resources>views folder and make a view file named sitemap-articles.blade.php. Then open the file & paste this code:
<?php echo '<?xml version="1.0" encoding="UTF-8"?>'; ?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
@foreach ($articles as $article)
<url>
<loc> {{ url('') . '/' . $article->slug }}</loc>
<lastmod>{{ $article->created_at->tz('UTC')->toAtomString() }}</lastmod>
<changefreq>weekly</changefreq>
<priority>0.5</priority>
</url>
@endforeach
</urlset>
Make Route & Test
Open web.php from the routes folder and make a route like:
Route::get('/sitemap-articles.xml', 'SitemapController@articles');
Now run the project and visit http://example.com/sitemap-articles.xml
. You’ll see the output like:
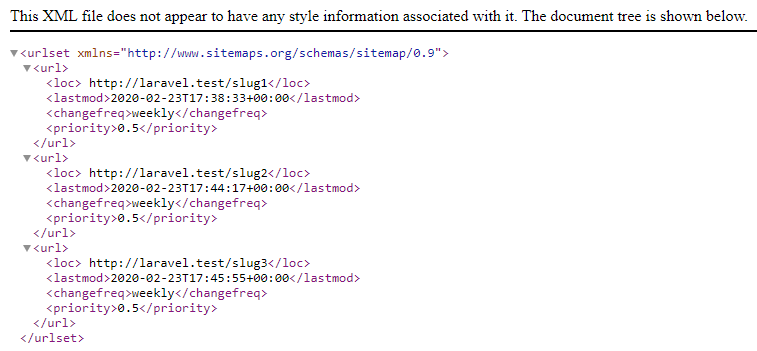
That’s all. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.