How to Use Custom Pagination in Laravel 10
Hello Artisan, today I'll show you how to use custom pagination in our Laravel application. As we all know that laravel provide the pagination by default. And to meet our design we need to customize the pagination design. So, today in this tutorial today we'll see how custom pagination in our application to meet our requirements.
First of all, we'll create some dummy data, so that we can test our pagination seamlessly. And for that we'll use Tinker. And if you're new on tinker, I'd recommend you go through this article. Now fire the below commands in the terminal.
php artisan migrate
php artisan tinker
App\Models\User::factory()->count(30)->create()
This will migrate all the migrations and then create 30 users to the “users” table.
At first we'll create a controller by firing the below command
php artisan make:controller PaginationController
It'll create a controller under app/Http/Controllers named PaginationController, open the file and put index() method in your controller. Look at the below source code.
<?php
namespace App\Http\Controllers;
use App\Models\User;
class PaginationController extends Controller
{
public function index()
{
$data = [
'users' => User::paginate(10)
];
return view('users',$data);
}
}
Put the below route in the web.php file.
Route::get('users', [App\Http\Controllers\PaginationController::class, 'index']);
Now we need to setup the blade file, so that we can user your custom pagination file. So, let's create a blade file called custom.blade.php and put the below source code.
@if ($paginator->hasPages())
<nav aria-label="Page navigation example">
<ul class="pagination justify-content-end">
@if ($paginator->onFirstPage())
<li class="page-item disabled">
<a class="page-link" href="#" tabindex="-1">Previous</a>
</li>
@else
<li class="page-item"><a class="page-link" href="{{ $paginator->previousPageUrl() }}">Previous</a></li>
@endif
@foreach ($elements as $element)
@if (is_string($element))
<li class="page-item disabled">{{ $element }}</li>
@endif
@if (is_array($element))
@foreach ($element as $page => $url)
@if ($page == $paginator->currentPage())
<li class="page-item active">
<a class="page-link">{{ $page }}</a>
</li>
@else
<li class="page-item">
<a class="page-link" href="{{ $url }}">{{ $page }}</a>
</li>
@endif
@endforeach
@endif
@endforeach
@if ($paginator->hasMorePages())
<li class="page-item">
<a class="page-link" href="{{ $paginator->nextPageUrl() }}" rel="next">Next</a>
</li>
@else
<li class="page-item disabled">
<a class="page-link" href="#">Next</a>
</li>
@endif
</ul>
@endif
Now we 'll show all of users that generate via tinker in step1 using our custom pagination that we create above.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css"
integrity="sha384-B0vP5xmATw1+K9KRQjQERJvTumQW0nPEzvF6L/Z6nronJ3oUOFUFpCjEUQouq2+l" crossorigin="anonymous">
<style>
.page-item.active .page-link{
z-index: 3;
color: #fff !important ;
background-color: #000000 !important;
border-color: #000000 !important;
border-radius: 50%;
padding: 6px 12px;
}
.page-link{
z-index: 3;
color: #000000 !important;
background-color: #fff;
border-color: #000000;
border-radius: 50%;
padding: 6px 12px !important;
}
.page-item:first-child .page-link{
border-radius: 10% !important;
}
.page-item:last-child .page-link{
border-radius: 10% !important;
}
.pagination li{
padding: 3px;
}
.disabled .page-link{
color: #212529 !important;
opacity: 0.5 !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<div class="card">
<div class="card-header">
<h4>Laravel 10 Custom Pagination Example Tutorial - <span class="text-dark">shouts.dev</span></h4>
</div>
<div class="card-footer">
<table class="table table-bordered">
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
@forelse($users as $key => $user)
<tr>
<td>{{ $users->firstItem() + $key }}</td>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
</tr>
@empty
<tr>
<td colspan="3">There Are no Data</td>
</tr>
@endforelse
</tbody>
</table>
<div class="col-md-12">
{{ $users->links('vendor.pagination.custom')}}
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Now it's the time to check the output. Go to localhost:8000/users, and you'll find the below output.
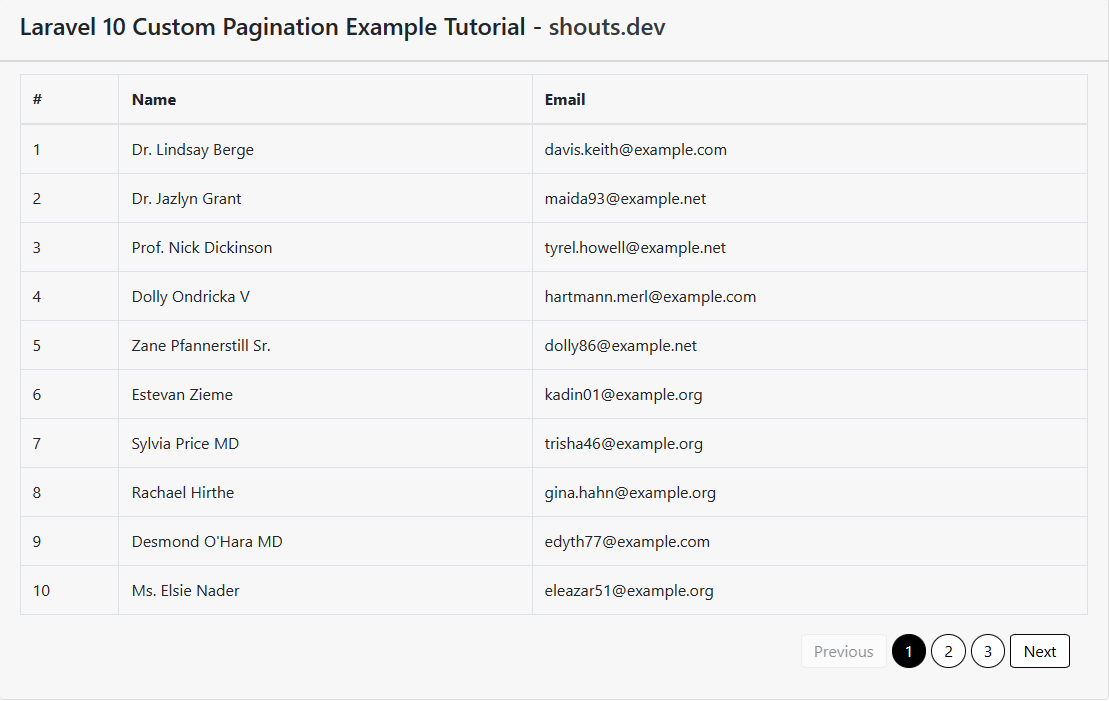
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂