Laravel Auto Complete Search Using Typeahead
hi artisans, today I’m going to show you how to make an auto-complete search using typeahead.js. Typeahead.js is a flexible JavaScript library that provides a strong foundation for building robust typeahead. I’m testing on Laravel 8.9.0. Let’s get started:
Table of Contents
- Install Laravel and Basic Configurations
- Create Dummy Data
- Create Controller
- Create View File
- Define Routes
- Output
Install Laravel and Basic Configurations
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Create Dummy Data
Navigate to database/seeders directory and open DatabaseSeeder.php file. Let’s import 50 dummy user data:
<?php
namespace Database\Seeders;
use App\Models\User;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
// create 50 fake users
User::factory(50)->create();
}
}
Now migrate the migration with seed:
php artisan migrate:fresh --seed
Create Controller
We need to a controller to show search page and process search results.
php artisan make:controller AutoCompleteSearchController
Now open the controller and paste this code:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class AutoCompleteSearchController extends Controller
{
// index page
public function index()
{
return view('auto_complete_search');
}
// return search results
public function query(Request $request)
{
$input = $request->all();
$data = User::select("name")
->where("name", "LIKE", "%{$input['query']}%")
->get();
return response()->json($data);
}
}
Create View File
We need to create a blade file named auto_complete_search.blade.php inside resources/views folder.
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel Auto Complete Search Using Typeahead - MyNotePaper.com</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<div class="container" style="max-width: 750px; margin-top: 50px;">
<div class="text-center">
<h5 class="text-center">Laravel + Typeahead Auto Complete Search</h5>
<input type="text" class="form-control typeahead" placeholder="Start typing something to search...">
</div>
</div>
</body>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-3-typeahead/4.0.2/bootstrap3-typeahead.min.js"></script>
<script type="text/javascript">
var path = "{{ route('autocomplete.search.query') }}";
$('input.typeahead').typeahead({
source: function (query, process) {
return $.get(path, {query: query}, function (data) {
return process(data);
});
}
});
</script>
</html>
Define Routes
This is the last step. Let’s register two routes in routes/web.php:
use App\Http\Controllers\AutoCompleteSearchController;
Route::get('/auto-complete-search', [AutoCompleteSearchController::class, 'index'])->name('autocomplete.search.index');
Route::get('/auto-complete-search-query', [AutoCompleteSearchController::class, 'query'])->name('autocomplete.search.query');
Output
We’re done. Run the project and visit /auto-complete-search
route to see the output:
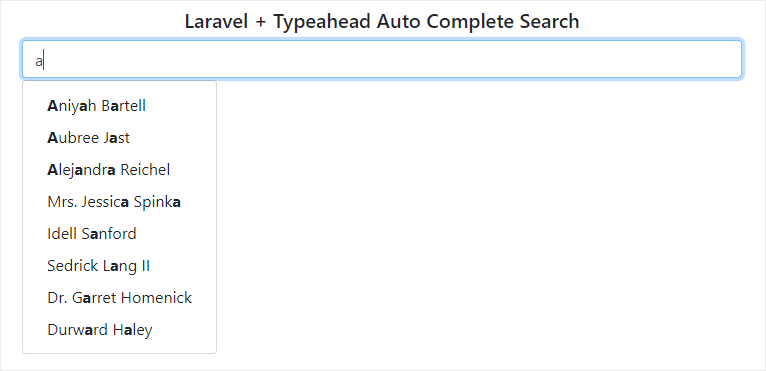
That’s it. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.