Laravel 10 - Convert CSV file to JSON/Array
Hello Artisan, today I'll show you how to convert a CSV file into JSON. JSON is the globally used format which we can use in every language. So, let's start how we can easily convert an CSV file into JSON.
Note: Tested on Laravel 10.0
First, we'll create a controller called ConverterController.php where we'll write our logic to convert the uploaded CSV file into . So, fire the below command in terminal to generate controller first.
php artisan make:controller ConverterController
It'll create a controller called ConverterController under App/Http/Controllers, now what we'll do is to add logic to our controller. See the below source code.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class ConverterController extends Controller
{
public function csvToJson(Request $request)
{
try {
$filePath = $request->file('csv_file');
$keys = $newArray = $data = [];
if (($handle = fopen($filePath, 'r')) !== FALSE) {
$i = 0;
$delimiter = ',';
while (($lineArray = fgetcsv($handle, 4000, $delimiter, '"')) !== FALSE) {
for ($j = 0; $j < count($lineArray); $j ) {
$data[$i][$j] = $lineArray[$j];
}
$i ;
}
fclose($handle);
}
$count = count($data) - 1;
$labels = array_shift($data);
foreach ($labels as $label) {
$keys[] = $label;
}
$keys[] = 'id';
for ($i = 0; $i < $count; $i ) {
$data[$i][] = $i;
}
for ($j = 0; $j < $count; $j ) {
$d = array_combine($keys, $data[$j]);
$newArray[$j] = $d;
}
dd(json_encode($newArray));
} catch (\Exception $e) {
dd($e);
}
}
}
Now we'll add routes which will let us show the form where user can upload the csv file. So, let's add the below route to our web.php.
Route::post('json-converter', [\App\Http\Controllers\ConverterController::class , 'csvToJson'])->name('json.converter');
Now we'll build a form where user can upload the CSV file. For that we'll use welcome.blade.php which comes with laravel by default. So, let's see the below source code.
<!DOCTYPE html>
<html>
<head>
<title>Laravel 10 Sweet Alert Confirm Delete Example - shouts.dev</title>
<meta name="csrf-token" content="{{ csrf_token() }}">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link href="https://cdnjs.cloudflare.com/ajax/libs/limonte-sweetalert2/5.0.7/sweetalert2.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<form action="{{ route('json.converter') }}" method="post" enctype="multipart/form-data">@csrf
<div class="row">
<div class="col-lg-12">
<div class="form-group">
<label for="csv_file">CSV File</label>
<input type="file" name="csv_file" id="csv_file" class="form-control">
</div>
</div>
<div class="col-lg-12">
<button type="submit" class="btn btn-primary">Save</button>
</div>
</div>
</form>
</div>
</body>
</html>
And finally, we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well (hope so) we can see the below output.
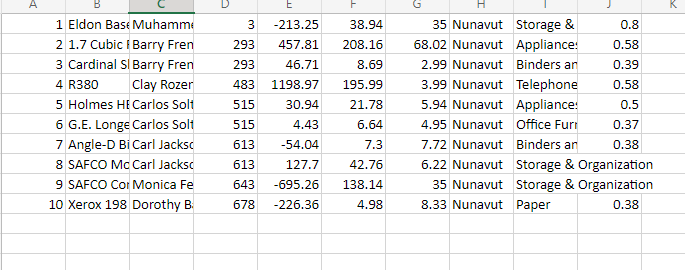
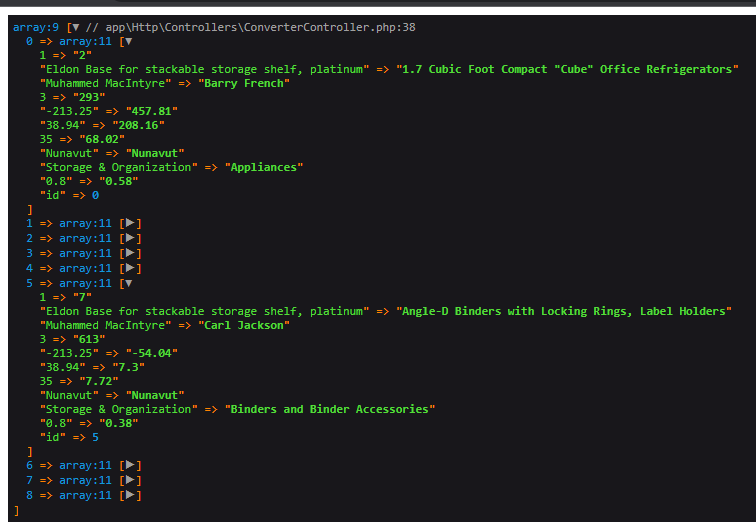
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐