Laravel Resource Route and Controller
Hello Artisans, today I'll tell you about one of the best feature of laravel is resource route and controller. How you can easily perform you r CRUD operation using resource route and controller. Laravel resource route and controller is one of the most efficient way to keep neat and clean your web.php file. Let's see how we can use Laravel resource route and controller.
Note: Tested on Laravel 9.19
At first we'll see some traditional way to declare routes.
<?php
use Illuminate\Support\Facades\Route;
Route::controller(\App\Http\Controllers\ArticleController::class)->group(function(){
Route::get('articles', 'index')->name('articles.index');
Route::post('articles', 'store')->name('articles.store');
Route::get('articles/create', 'create')->name('articles.create');
Route::get('articles/{item}', 'show')->name('articles.show');
Route::put('articles/{item}', 'update')->name('articles.update');
Route::delete('articles/{item}', 'destroy')->name('articles.destroy');
Route::get('articles/{item}/edit', 'edit')->name('articles.edit');
});
It'll produce the below result
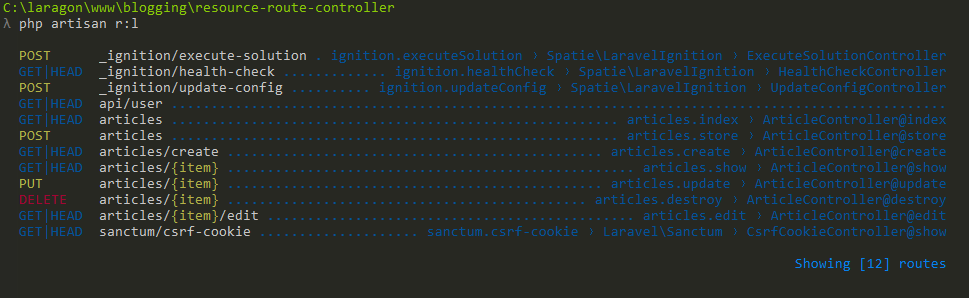
Here what we'll do is, we'll define 7 routes one by one. Which is kind of boring and time killing as well. Do you know we can achieve the same result just in one single line? Yes, we can easily achieve the above result using resource route. Let's see the below code snippet.
<?php
use Illuminate\Support\Facades\Route;
Route::resource('articles',\App\Http\Controllers\ArticleController::class);
And it'll produce the same result as previous one.
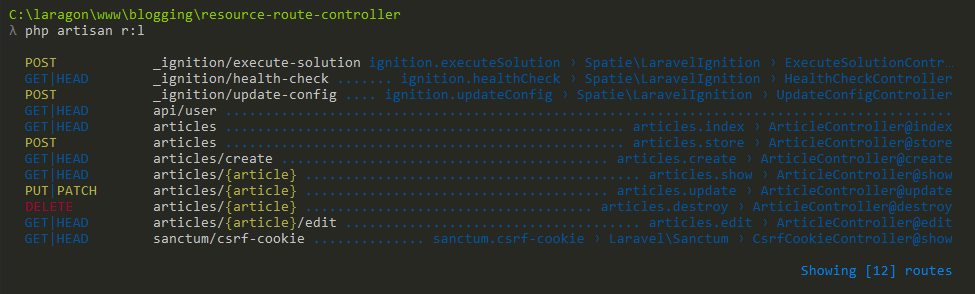
In this step we'll talk about the Resource controller. If we want to perform a CRUD operation for an specific model, just fire the below command in terminal.
php artisan make:model Article -cr
And it'll create a model called Article.php and a resource controller called ArticleController corresponding to to the model. Let's look at the below controller.
<?php
namespace App\Http\Controllers;
use App\Models\Article;
use Illuminate\Http\Request;
class ArticleController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param \App\Models\Article $article
* @return \Illuminate\Http\Response
*/
public function show(Article $article)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Models\Article $article
* @return \Illuminate\Http\Response
*/
public function edit(Article $article)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Article $article
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Article $article)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Article $article
* @return \Illuminate\Http\Response
*/
public function destroy(Article $article)
{
//
}
}
So, the last say is to perform a quick CRUD operation, resource route and controller is one of the best effective method.
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐