Laravel Integrate Place Autocomplete using Google Map API
Hello Artisans, today I'll show you how to add google autocomplete in your laravel application. Google autocomplete nowadays quite necessary for a website. Suppose you have a restaurant, and the user doesn't know where the restaurant is located. But if there is google autocomplete, then the user can search for your restaurant So, let's see how we can easily integrate place autocomplete in google map in our laravel application.
Note: Tested on Laravel 9.19
Now, we'll add new variable in .env file to set google map api key which we'll use later in blade file. See below source code
GOOGLE_MAP_KEY=google_api_key
Now we'll update our default blade file called welcome.blade.php which is come by default. Now open the file and replace with below codes.
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Google Autocomplete in Laravel - shouts.dev</title>
</head>
<body>
<div class="container mt-5">
<h2>Google Autocomplete Address in Laravel - shouts.dev</h2>
<div class="form-group">
<label>Location/City/Address</label>
<input type="text" name="autocomplete" id="autocomplete" class="form-control" placeholder="Choose Location">
</div>
</div>
<script>
function initMap() {
const input = document.getElementById("autocomplete");
const options = {
fields: ["formatted_address", "geometry", "name"],
strictBounds: false,
types: ["establishment"],
};
const autocomplete = new google.maps.places.Autocomplete(input, options);
autocomplete.addListener("place_changed", () => {
const place = autocomplete.getPlace();
if (!place.geometry || !place.geometry.location) {
// User entered the name of a Place that was not suggested and
// pressed the Enter key, or the Place Details request failed.
window.alert("No details available for input: '" place.name "'");
return false;
}
});
}
window.initMap = initMap;
</script>
<script type="text/javascript"
src="https://maps.google.com/maps/api/js?key={{ env('GOOGLE_MAP_KEY') }}&callback=initMap&libraries=places&v=weekly" ></script>
</body>
</html>
And finally, we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well (hope so) we can see the below output.
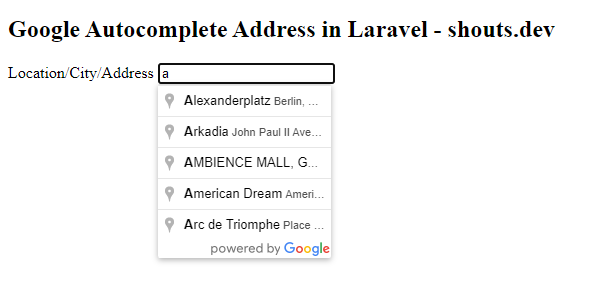
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐