How to Show Data in Modal using Ajax in Laravel 10
Hello Artisan, today I'll show you how to display data in modal popup laravel application.In this tutorial, you'll learn how to display data in a Bootstrap modal popup using Laravel. I'll guide you through a simple step-by-step process, explaining how to use Laravel to show data in the modal using AJAX.
In this example, we'll create a list of users with a "Show" button. When the button is clicked, we'll open a modal and retrieve data using AJAX to display it. You can fetch data using jQuery AJAX in Laravel versions 6, 7, 8, 9, and 10.
Let's follow the steps:
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project laravel/laravel AjaxModal
Feel free to ignore this step if you've already created the Laravel app.
Here are the commands for creating dummy records on the users table and importing them:
Create Dummy Records:
php artisan tinker
User::factory()->count(20)->create()
At this stage, we need to develop a new controller called UserController. This controller will have two methods: index() and show(). The index method will be responsible for returning users with filtering capabilities.
Let's update following code to your controller file:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index()
{
$users = User::paginate(20);
return view('user.index', compact('users'));
}
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$user = User::find($id);
return response()->json($user);
}
}
To create a route for listing users, add the following line to your “routes/web.php” file
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\UserController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('users', [UserController::class, 'index']);
Route::get('users/{id}', [UserController::class, 'show'])->name('users.show');
In the last step, create index.blade.php in “resources/views/user” and design the layout for user-related content.
<!DOCTYPE html>
<html>
<head>
<title>Laravel Ajax Data Fetch Example</title>
<meta name="csrf-token" content="{{ csrf_token() }}">
<link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<div class="container">
<h1>Laravel Ajax Data Fetch Example</h1>
<table class="table table-bordered data-table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach($users as $user)
<tr>
<td>{{ $user->id }}</td>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
<td>
<a
href="javascript:void(0)"
id="show-user"
data-url="{{ route('users.show', $user->id) }}"
class="btn btn-info"
>Show</a>
</td>
</tr>
@endforeach
</tbody>
</table>
{{ $users->links() }}
</div>
<!-- Modal -->
<div class="modal fade" id="userShowModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Show User</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<p><strong>ID:</strong> <span id="user-id"></span></p>
<p><strong>Name:</strong> <span id="user-name"></span></p>
<p><strong>Email:</strong> <span id="user-email"></span></p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
</body>
<script type="text/javascript">
$(document).ready(function () {
/* When click show user */
$('body').on('click', '#show-user', function () {
var userURL = $(this).data('url');
$.get(userURL, function (data) {
$('#userShowModal').modal('show');
$('#user-id').text(data.id);
$('#user-name').text(data.name);
$('#user-email').text(data.email);
})
});
});
</script>
</html>
Simply type the command below and press enter to run the Laravel app
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
http://localhost:8000/users
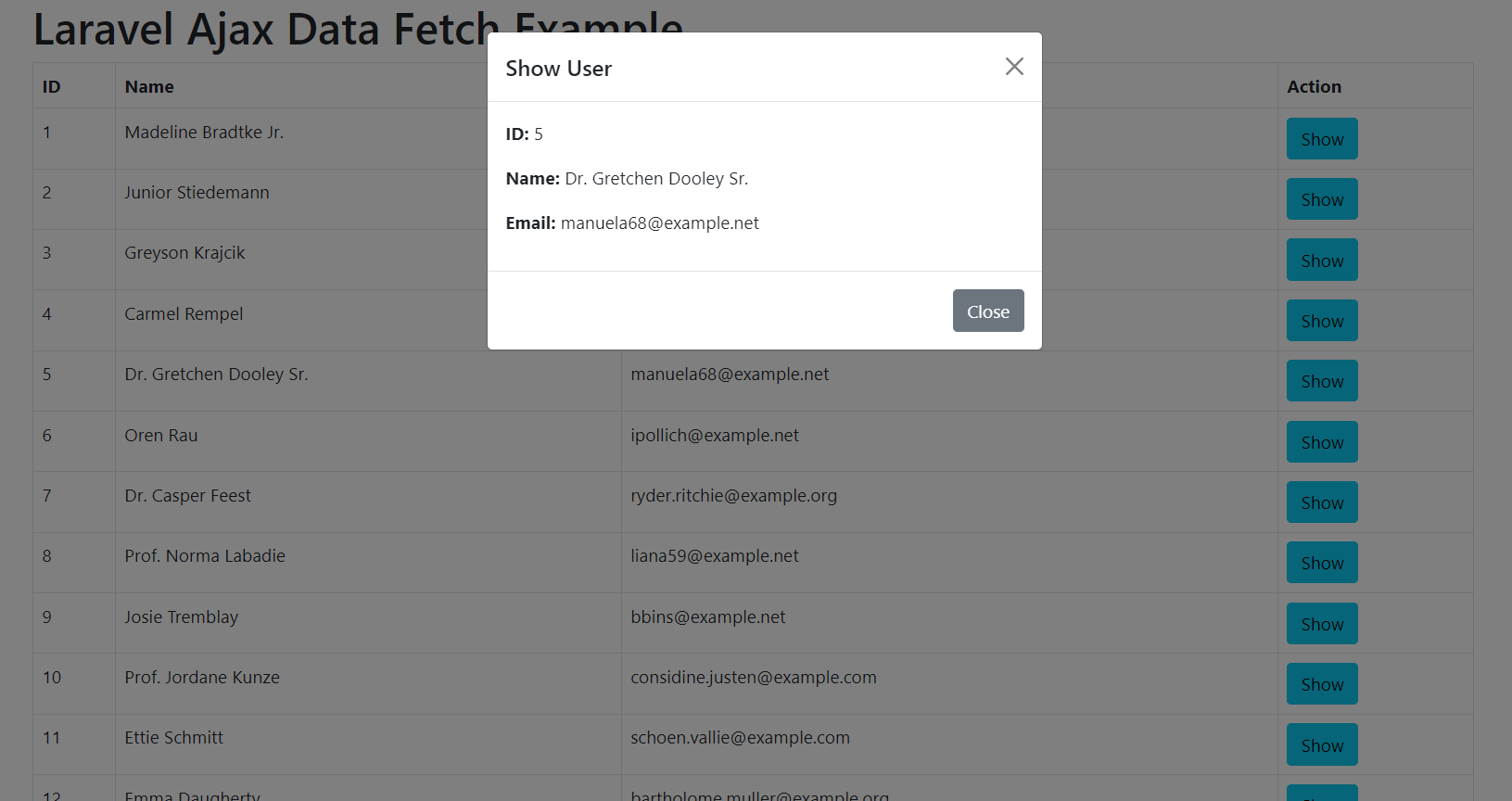
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂