Laravel Integrate Google ReCaptcha v2 in Laravel Application
Hello Artisans, today I'll show you how to integrate google ReCaptcha into our Laravel Application. Google Recaptcha is a handy tool to prevent robots or who kept busy on your site by requesting the resource again and again until the server crash or doesn't respond anymore. So, let's see how we can easily integrate google ReCaptcha into our Laravel Application.
Note: Tested on Laravel 9.19.
Table of Contents
Create and Setup Controller
In the very first step, we'll create a controller and also we'll write our necessary codes/logics.
Now fire the below command in terminal
php artisan make:controller RecaptchaController
It'll create a file under app\Http\Controllers called RecaptchaController.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class RecaptchaController extends Controller
{
public function index()
{
return view('recaptcha');
}
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'email' => 'required|email',
'phone' => 'required',
'stack' => 'required',
'g-token' => 'required',
],
[
'g-token.required' => 'Please verify that you are not a robot.'
]);
dd('Successfully validated');
}
}
Create and Setup Route
Let's put the below route in web.php
Route::resource('recaptcha', RecaptchaController::class)->only([
'index', 'store'
]);
Create and Setup View File
Now we we'll create a blade file where we can setup our view where we can see our form with google recaptcha protected. So let's create a view file called recaptcha.blade.php under resources/views. Now we need to setup so that user have to check google recaptcha. But hang on there. Before setup we need to enable google recaptcha API keys from the below link reCAPTCHA (google.com). Here you'll get the below form
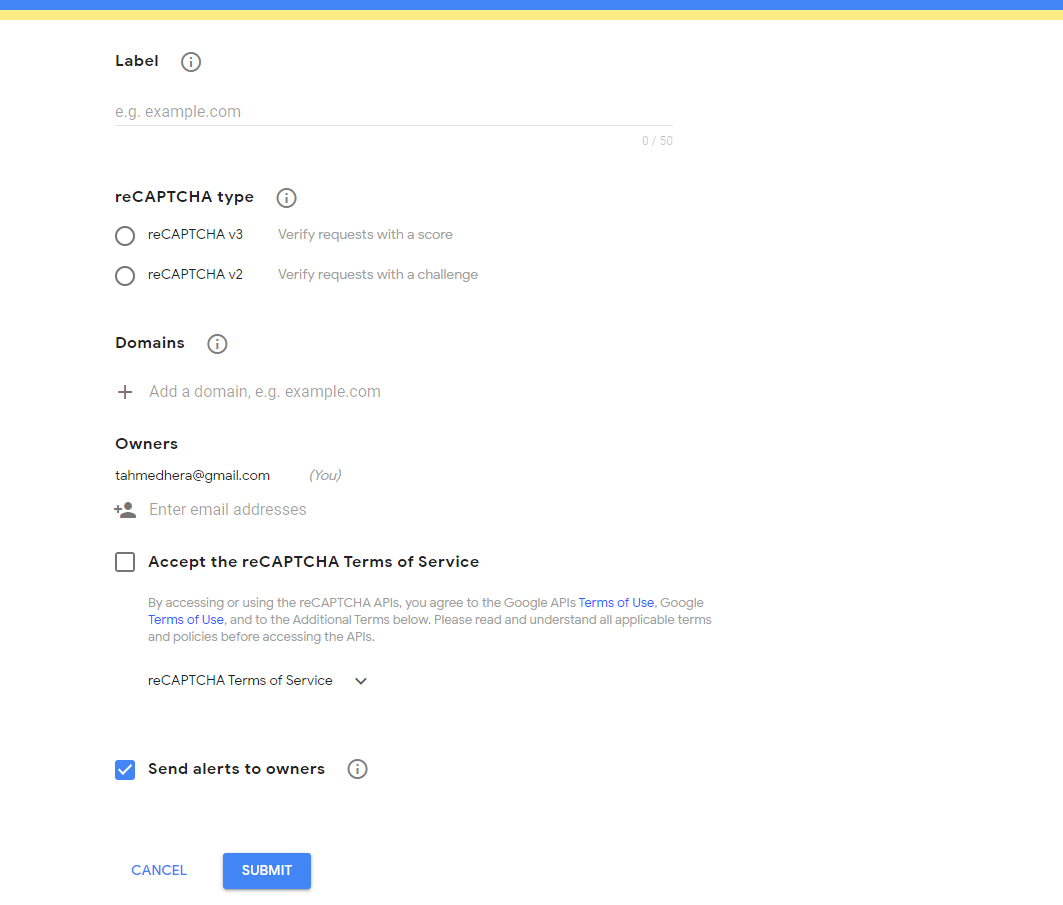
Now fill up the form like in the below picture.
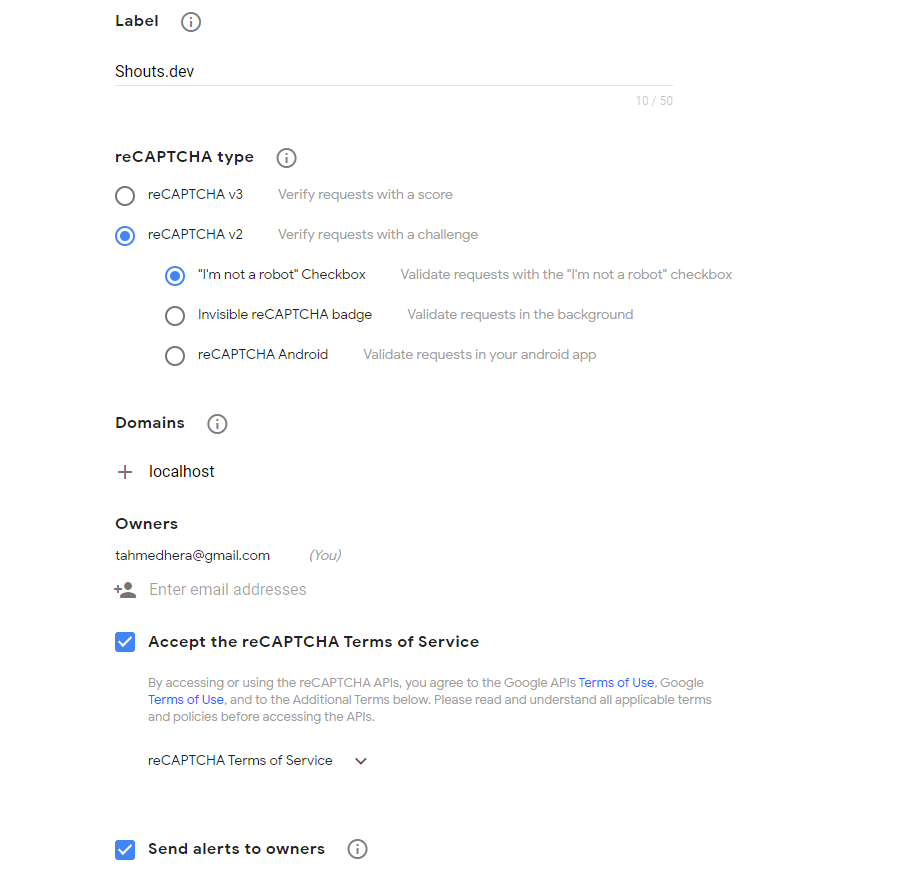
And click "Submit". After clicking to the submit you'll be redirected to next page. Where you'll get your API keys. Save them for now.
Now back to our application. Open the recaptcha.blade.php that we'll create earlier. Open the file paste the following code.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Google Recaptcha - shouts.dev</title>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<script src="https://www.google.com/recaptcha/api.js"></script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h4>Google Recaptcha Form - shouts.dev</h4>
<form action="{{ route('recaptcha.store') }}" method="post">@csrf
<div class="form-group">
<label for="name">Name</label>
<input type="text" name="name" id="name" class="form-control">
<span class="text-danger">{{ $errors->first('name') }}</span>
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="email" name="email" id="email" class="form-control">
<span class="text-danger">{{ $errors->first('name') }}</span>
</div>
<div class="form-group">
<label for="phone">Phone</label>
<input type="text" name="phone" id="phone" class="form-control">
<span class="text-danger">{{ $errors->first('name') }}</span>
</div>
<div class="form-group">
<label for="phone">Phone</label>
<select class="form-control" id="sel1" name="stack">
<option value="">Select One</option>
<option value="php">PHP</option>
<option value="python">Python</option>
<option value="js">Javascript</option>
<option value="laravel">Laravel</option>
</select>
<span class="text-danger">{{ $errors->first('stack') }}</span>
</div>
<div class="form-group">
<label for="address">Address</label>
<input type="text" name="address" id="address" class="form-control">
</div>
<input type="hidden" name="g-token" id="recaptchaToken">
<div class="g-recaptcha"
data-sitekey="6LdgeOIUAAAAABcXEV3jTS1lxrE0LsdrHaL7WwlO"
data-callback='onSubmit'
data-action='submit'>Submit</div>
<span class="text-danger">{{ $errors->first('g-token') }}</span>
<div class="form-group">
<button type="submit" class="btn btn-success">Submit</button>
</div>
</form>
</div>
</div>
</div>
<script>
function onSubmit(token) {
document.getElementById("recaptchaToken").value = token;
}
</script>
</body>
</html>
And that's it. Now modify a little bit with your site key. In the data-sitekey attribute replace it with your google recaptcha api key which you got it after submitting the google recaptcha form.
<div class="g-recaptcha"
data-sitekey="your_site_ket"
data-callback='onSubmit'
data-action='submit'>Submit</div>
Output
And finally we're ready with our setup. It's time to check our output. Now go to http://localhost:8000/recaptcha, If everything goes well (hope so) you can find a below output.
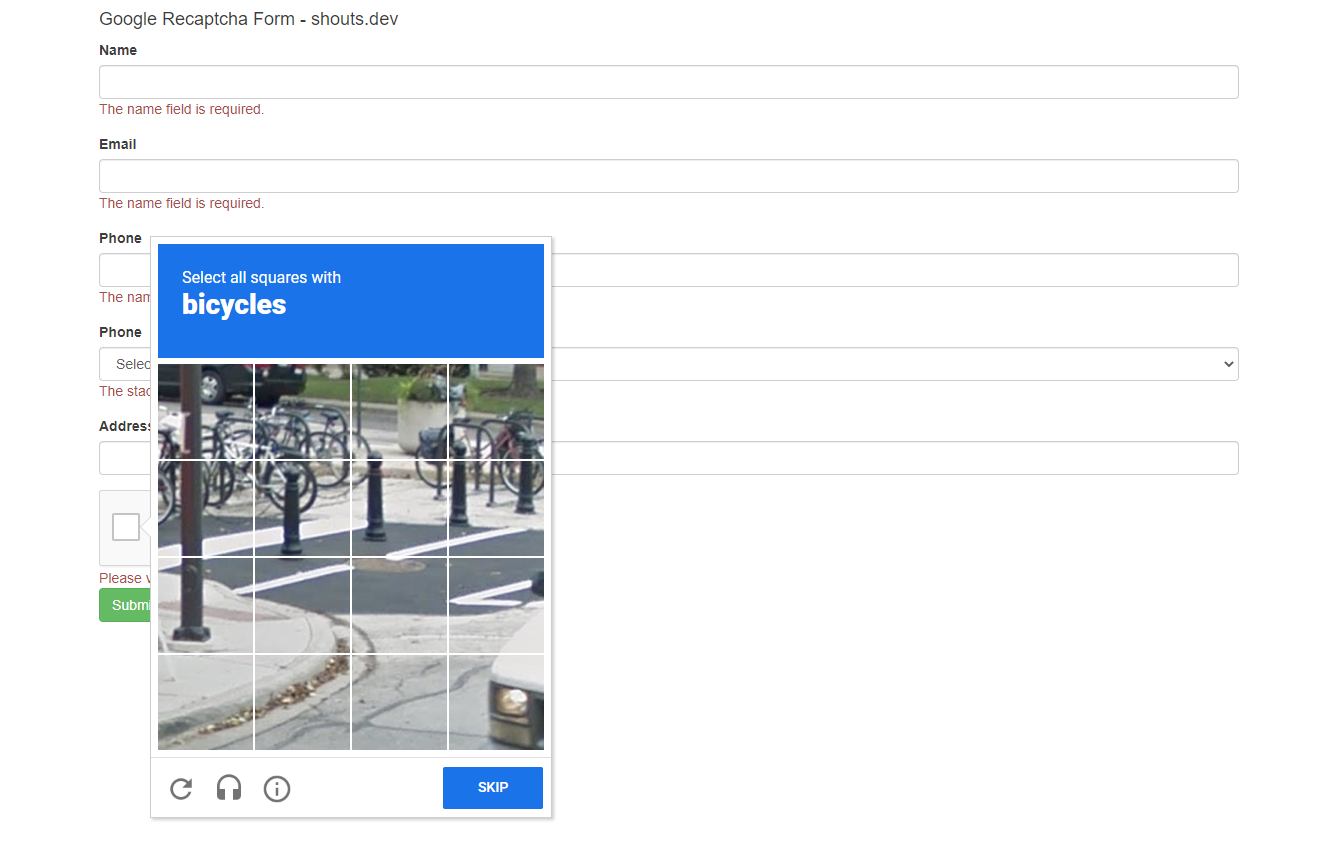
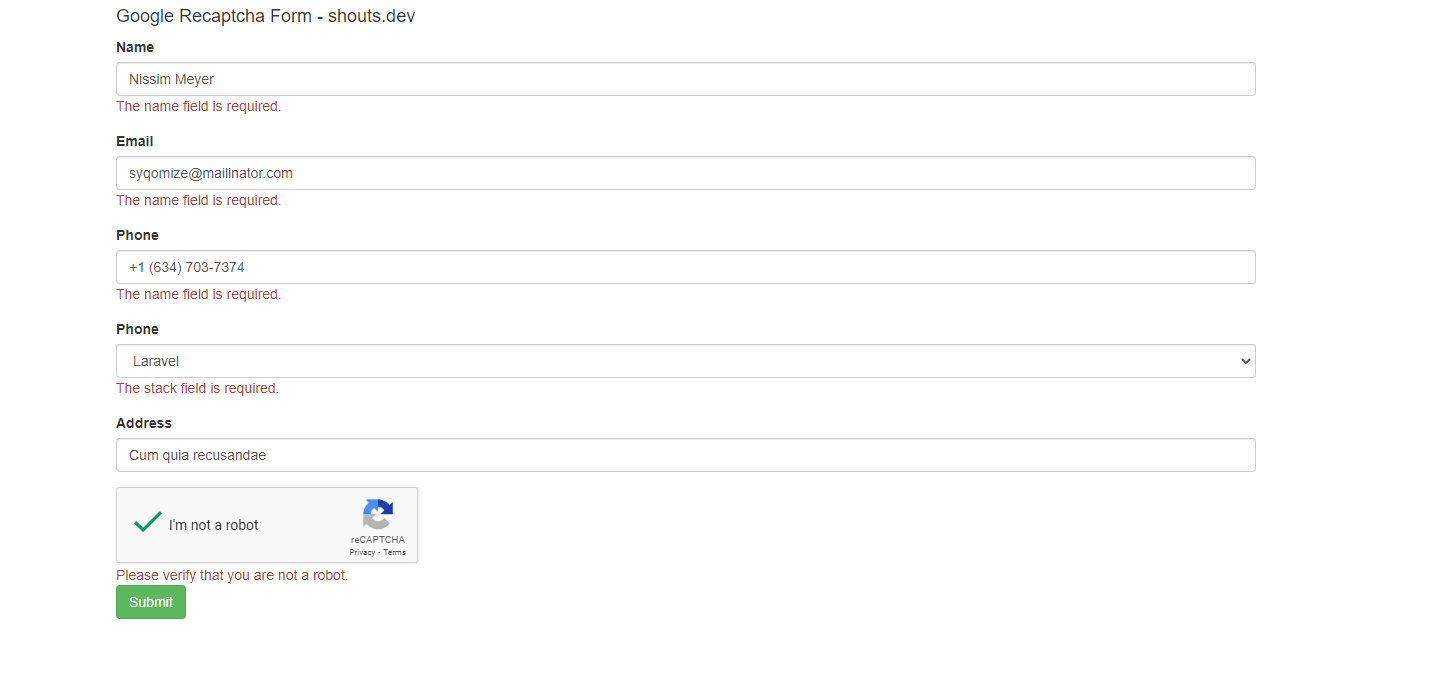
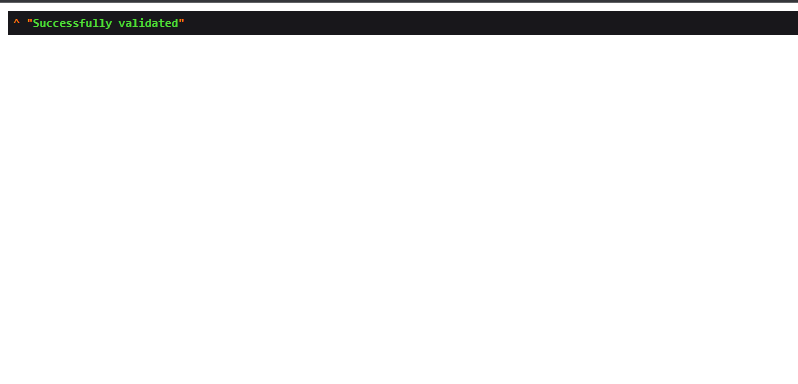
That's it for today. Hope you'll enjoy this tutorial. Catch me in the comment section if anything goes wrong. You can also download this tutorial from GitHub. Thanks for reading.