VueJs How to Download File using Axios
Hello devs, today I'll show you how to download files using axios in VueJS application. Axios is one of the most popular way to fetch API's form our server end. Fore more info you can see here. And today we'll use that one to download our files from backend. So, let's see how we can easily implement in our VueJs application.
Note: Tested on VueJS 2.6.14 and Axios 0.19.0.
We'll use the below source code to download our file.
<!DOCTYPE html>
<html>
<head>
<title>How to Download File using Axios Vue JS? - ItSolutionStuff.com</title>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.19.0/axios.min.js" integrity="sha256-S1J4GVHHDMiirir9qsXWc8ZWw74PHHafpsHp5PXtjTs=" crossorigin="anonymous"></script>
</head>
<body>
<div id="app">
<button @click="onClick()">DownLoad</button>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
methods: {
onClick() {
axios({
url: 'http://localhost:63342/pdf_download/my.pdf',
method: 'GET',
responseType: 'blob',
}).then((response) => {
var fileURL = window.URL.createObjectURL(new Blob([response.data]));
var fileLink = document.createElement('a');
fileLink.href = fileURL;
fileLink.setAttribute('download', 'file.pdf');
document.body.appendChild(fileLink);
fileLink.click();
});
}
}
})
</script>
</body>
</html>
Here what we do is, at first we'll call axios using get request and we'll also specify the responseType to blob as we want to download files. And if everything goes well it'll produce the below output.
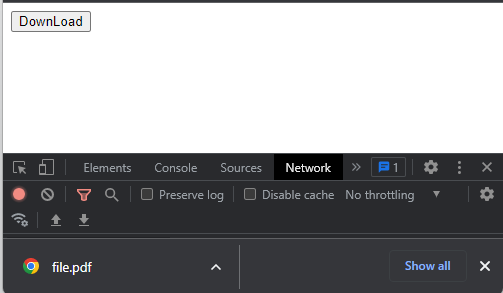
That's it for today. I hope you've enjoyed this tutorial. If you found any difficulties o questions, catch me in comment sections. Thanks for reading. ๐