Laravel 10 How to Call External API in Laravel
Hello Artisan, today I'll show you how to call external API to connect other web application thorough our Laravel application. For that we're going to use Laravel HTTP Client. Of course there's lot of client available like Guzzle, PHP HTTP Client, even you can make call with PHP CURL etc. But HTTP client is well featured and easy to use according to the Guzzle or curl and it's provided by Laravel default. So, no more talk let's see how we can easily connect other application with our Laravel application.
So at first, we'll create a controller by firing the below command.
php artisan make:controller PostController
It'll create a controller under app/Http/Controllers called PostController, open the file and below code in your controller. Look at the below source code.
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Crypt;
use Illuminate\Support\Facades\Http;
class PostController extends Controller
{
public function index()
{
$response = Http::get('https://jsonplaceholder.typicode.com/posts');
$jsonData = $response->json();
dd($jsonData);
}
public function store()
{
$response = Http::post('https://jsonplaceholder.typicode.com/posts', [
'title' => 'This is test from shouts.dev as title',
'body' => 'This is test from shouts.dev as body',
]);
$jsonData = $response->json();
dd($jsonData);
}
}
Put the below route in the web.php file.
<?php
use App\Http\Controllers\PostController;
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return view('welcome');
});
Route::get('posts', [PostController::class,'index']);
Route::get('store/posts', [PostController::class,'store']);
Now it's the time to check the output. Go to localhost:8000/posts, and you'll find the below output.
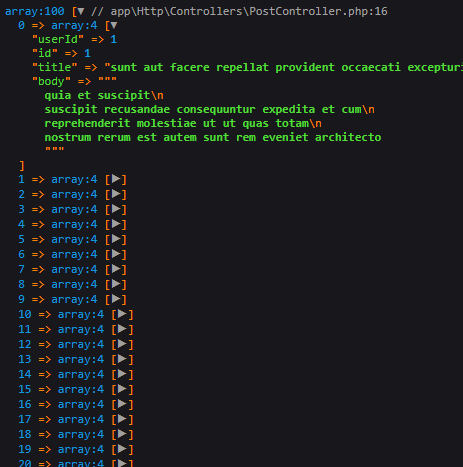
Go to localhost:8000/store/posts, and you'll find the below output
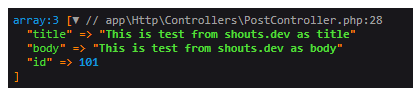
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. ๐