How to Force User to Use Strong Password in Laravel
Hello artisans, today I’ll show you how to prevent users from using weak/regular password like “123456”, “abcd”, “qwerty” etc. As Laravel default validation rule doesn’t provide this one so we will use “unicodeveloper/laravel-password” package, which was very helpful and easy to integrate. So, no more talking and get dive into the topic.
Note: Tested on Laravel 8.54.
Table of Contents
Install Laravel and Basic Config
Each Laravel project needs this thing. For that you can see the very beautiful article on this topic from here: Install Laravel and Basic Configurations.
Install “unicodeveloper/laravel-password” package
To get the latest version of Laravel Password, simply run the following command in your terminal
composer require unicodeveloper/laravel-password
Run your migrations.
php artisan migrate
Use the validation rule “dumbpwd” in your RegisterController “validator()” function and you are done.
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Providers\RouteServiceProvider;
use App\Models\User;
use Illuminate\Foundation\Auth\RegistersUsers;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
class RegisterController extends Controller
{
use RegistersUsers;
protected $redirectTo = RouteServiceProvider::HOME;
public function __construct()
{
$this->middleware('guest');
}
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['required', 'string', 'min:8', 'confirmed','dumbpwd'],
]);
}
protected function create(array $data)
{
return User::create([
'name' => $data['name'],
'email' => $data['email'],
'password' => Hash::make($data['password']),
]);
}
}
By default, the error message is This password is just too common. Please try another!
.You can customize the error message by opening resources/lang/en/validation.php
and adding to the array like so:
'dumbpwd' => 'You are using a dumb password'
You can get more info from this GitHub repository.
Output
Finally run your project and see the output below
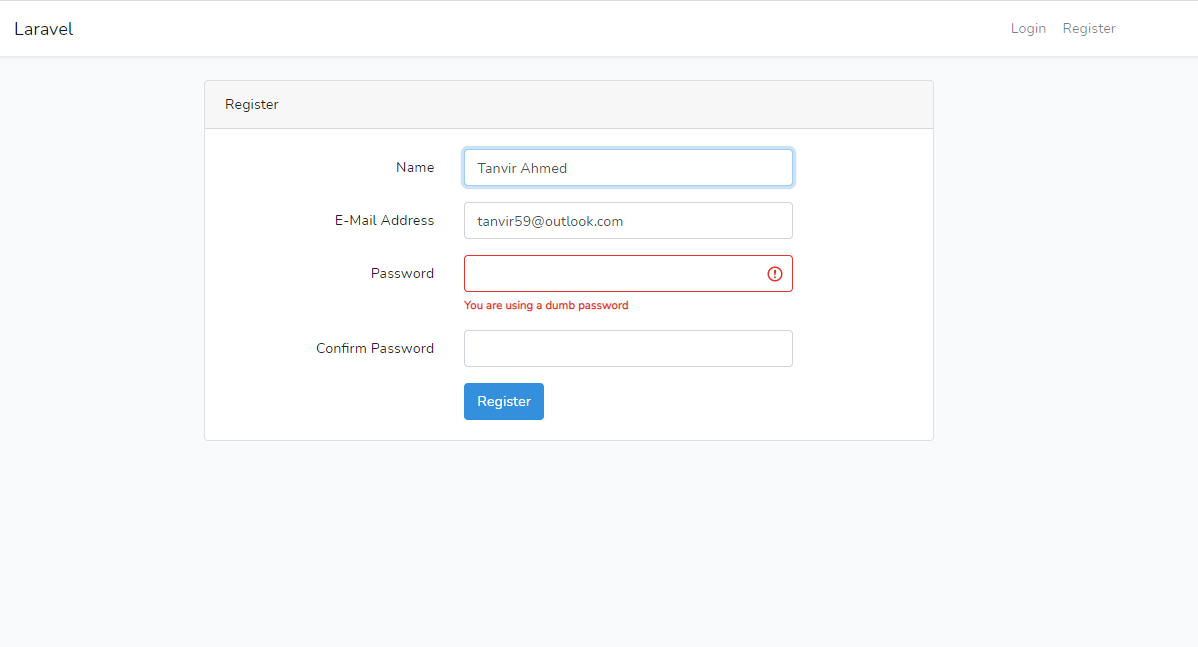
That’s all. You can download this project from GitHub. Thanks for reading.