Laravel 10 Toastr Notification Without any Package
Hello Artisan, today I'll show you how to use Toastr notification in our Laravel application without the use of any package. Most of the time what we do is use some packages of Laravel and use the CDN version of jquery library as well. But here we don't going to use any Laravel package. So, no more talk let's see how we can easily add toastr notification in our application.
So at first, we'll create a controller by firing the below command.
php artisan make:controller ToastrController
It'll create a controller under app/Http/Controllers, open the file, and put toastr() method in your controller. Look at the below source code.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class ToastrController extends Controller
{
public function toastr(): \Illuminate\Contracts\View\View|\Illuminate\Foundation\Application|\Illuminate\Contracts\View\Factory|\Illuminate\Contracts\Foundation\Application
{
session()->flash('success', 'This is a success message!');
session()->flash('info', 'This is an info message!');
session()->flash('warning', 'This is a warning message!');
session()->flash('error', 'This is an error message!');
return view('toastr');
}
}
Now we need to set up the blade file, so that we can show the result in a nice way.
<!DOCTYPE html>
<html>
<head>
<title>How To Notification Message PopUp Using Toastr Js Plugin Laravel 10 Example - shouts.dev</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" type="text/css"
href="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.js"></script>
</head>
<body>
<div class="container mt-5">
<div class="row">
<div class="col-md-6 mx-auto">
<div class="card">
<div class="card-header">
<h5>Dashboard</h5>
</div>
<div class="card-body">
<h5>Welcome to the shouts.dev</h5>
</div>
</div>
</div>
</div>
</div>
<script>
// success message popup notification
@if(session()->has('success'))
toastr.success("{{ session()->get('success') }}");
@endif
// info message popup notification
@if(session()->has('info'))
toastr.info("{{ session()->get('info') }}");
@endif
// warning message popup notification
@if(session()->has('warning'))
toastr.warning("{{ session()->get('warning') }}");
@endif
// error message popup notification
@if(session()->has('error'))
toastr.error("{{ session()->get('error') }}");
@endif
</script>
</body>
</html>
Put the below route in the web.php file.
Route::get('/toastr', [App\Http\Controllers\ToastrController::class, 'toastr'])->name('toastr');
Now it's time to check the output. Go to localhost:8000/toastr, and you'll find the below output.
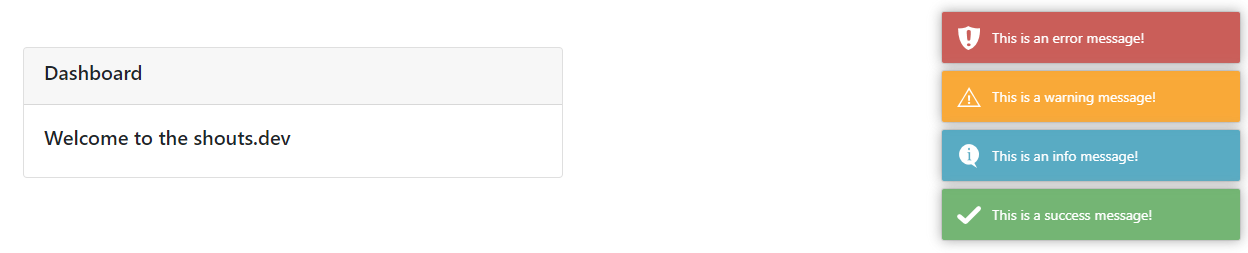
That's it for today. I hope it'll be helpful in upcoming projects. You can also download this source code from GitHub. Thanks for reading. ๐