File Validation using Pure JavaScript
Hi devs, I'll show you file size validation using pure JavaScript today. We often used it to validate our server-side code. That's good but not better. For seamless user experience, we should validate in real-time. So, let's see how we can easily integrate this using vanilla/pure JS.
File Size Validation
So, at first we'll see the below source code.
<!DOCTYPE html>
<html>
<head>
<title>File size validation using Javascript - shouts.dev</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row justify-content-center mt-5">
<div class="col-md-8">
<form enctype="multipart-formdata">
<div class="form-group">
<label for="image">Image</label>
<input type="file" class="form-control" name="file" id="image" onchange="fileSizeVaklidation()">
</div>
</form>
</div>
</div>
</div>
<script>
function fileSizeValidation() {
var image = event.target.files[0];
if (image) {
var size = parseFloat(image.size / (1024 * 1024)).toFixed(2);
if(size > 2) {
alert('Please select image size less than 2 MB');
}else{
alert('success');
}
} else {
alert("This browser does not support HTML5.");
}
}
</script>
</body>
</html>
Here what we do is, firstly we ran a onchange() event, so that when a user uploads a file we can execute our function called fileSizeValidation(). Where we give a condition like if a image size is below 2MB then it'll popup an alert saying "success" as below output we can see.
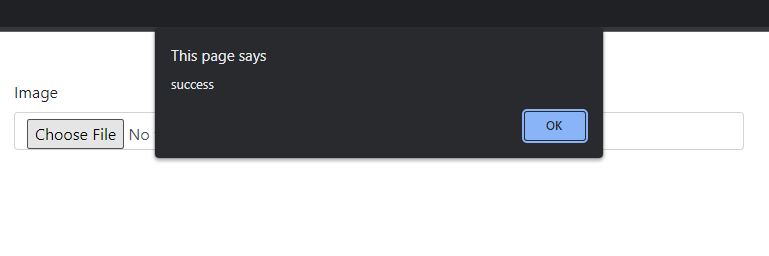
But if a file size above 2MB then the below screen will be appears.
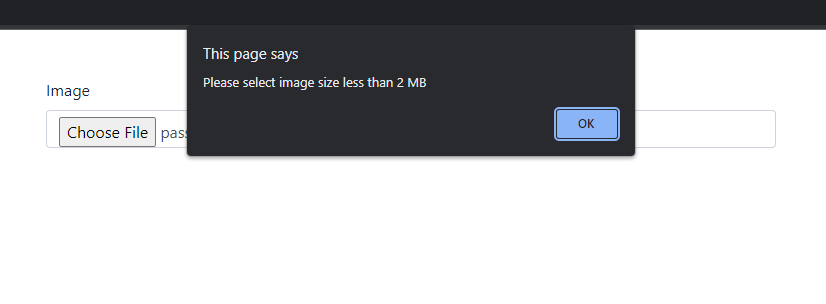
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐