Laravel Create Paginated Data from Array
Hello Artisans, today I'll show you how to paginate data from array in your laravel application. Sometimes it's quite necessary to show the paginated data through arrays of data. So, let's get started and see how we can easily integrate pagination in our laravel application using arrays of data.
Note: Tested on Laravel 9.19
First of all, create a controller so that we can write our logics or query to show the result. So, fire the below commands in the terminal.
php artisan make:controller PaginateController
It'll create a controller under app\Http\Controllers called PaginationController.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use Illuminate\Pagination\LengthAwarePaginator;
use Illuminate\Pagination\Paginator;
use Illuminate\Support\Collection;
class PaginateController extends Controller
{
public function index(): \Illuminate\Contracts\View\Factory|\Illuminate\Contracts\View\View|\Illuminate\Contracts\Foundation\Application
{
$myArray = [
['id' => 1, 'title' => 'Laravel'],
['id' => 2, 'title' => 'Symfony'],
['id' => 3, 'title' => 'CodeIgniter'],
['id' => 4, 'title' => 'CakePHP'],
['id' => 5, 'title' => 'Yii'],
['id' => 6, 'title' => 'Zend'],
['id' => 7, 'title' => 'Slim'],
['id' => 8, 'title' => 'Phalcon'],
['id' => 9, 'title' => 'FuelPHP'],
['id' => 10, 'title' => 'Kohana'],
['id' => 11, 'title' => 'Fat-Free Framework']
];
$stacks = $this->paginate($myArray);
return view('welcome', compact('stacks'));
}
public function paginate($items, $perPage = 5, $page = null, $options = [])
{
$page = $page ?: (Paginator::resolveCurrentPage() ?: 1);
$items = $items instanceof Collection ? $items : Collection::make($items);
return new LengthAwarePaginator($items->forPage($page, $perPage), $items->count(), $perPage, $page, $options);
}
}
Now we'll create routes so that we can access in our view file. So, put the below route in web.php file.
<?php
use Illuminate\Support\Facades\Route;
Route::get('/', [\App\Http\Controllers\PaginateController::class,'index'])->name('paginate');
Now we'll modify the default blade file which comes with the laravel named welcome.blade.php. Just replace with below codes.
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Pagination with Array - shouts.dev</title>
<!-- Fonts -->
<link href="https://fonts.bunny.net/css2?family=Nunito:wght@400;600;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<style>
body {
font-family: 'Nunito', sans-serif;
}
</style>
</head>
<body class="antialiased">
<div class="container mt-5">
<h4 class="text-center mb-3">Popular PHP Frameworks</h4>
<table class="table table-bordered">
<tr>
<th>Id</th>
<th>Title</th>
</tr>
@foreach($stacks as $stack)
<tr>
<td>{{ $stack['id'] }}</td>
<td>{{ $stack['title'] }}</td>
</tr>
@endforeach
</table>
<div class="text-center">
{{ $stacks->links('vendor.pagination.bootstrap-4') }}
</div>
</div>
</body>
</html>
And finally we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well you'll find a below output
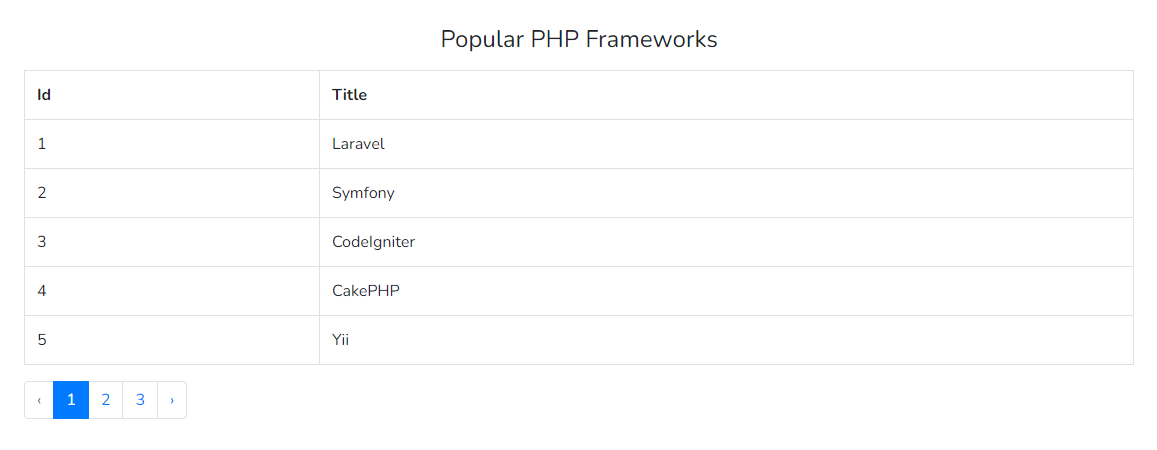
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐