VueJS How to Create Custom Directives
Hello devs, today I'll show you how to create custom directives using VueJS. Custom directives are used to encapsulate common DOM manipulations, and they can be applied to any element in your Vue.js templates. So, let's see how we can easily create custom directives in a just few steps.
Here we'll use the VUE@3 of the CDN version. So, first of all, let's look at the below code to ready our Vue app.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Creating a Custom Directives with VueJS - shouts.dev</title>
</head>
<body>
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
<div id="app">
</div>
<script>
const app = Vue.createApp({
data() {
return {
message: 'Hello Vue!'
}
}
})
app.mount('#app')
</script>
</body>
</html>
Then we'll add some custom directives to our app. So we'll add some html content on to the body part and add some directives into our script part. So, our final modified file will look like this below:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Creating a Custom Directives with VueJS - shouts.dev</title>
</head>
<body>
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
<div id="app">
<div v-my-directive="'Hello world'"></div>
<div v-highlight="'yellow'">Highlight me!</div>
<input type="text" v-focus>
</div>
<script>
const app = Vue.createApp({
data() {
return {
message: 'Hello Vue!'
}
}
})
app.directive('my-directive', {
beforeMount(el, binding) {
el.innerHTML = 'My directive says: ' + binding.value
}
})
app.directive('highlight', {
beforeMount(el, binding) {
el.style.backgroundColor = binding.value;
}
})
app.directive('focus', {
mounted(el) {
el.focus()
}
})
app.mount('#app')
</script>
</body>
</html>
Which can produce the below result
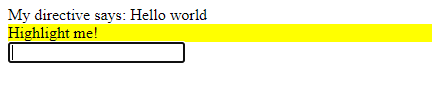
So, here we can see that, at first we'll create directive called my-directive and in the html we'll take a div called where we used as v-my-directive, which acts like an normal html attribute. Even we can use any complex calculation as like as any helper function. Suppose, your sites in BDT currency and want to convert your currency whenever the user want to change the currency to USD. Then we can build a below directive
<div v-usd-converter="150"></div>
app.directive('usd-converter', {
beforeMount(el, binding) {
el.innerHTML = binding.value + 'BDT in USD is: ' + parseFloat(binding.value * 106)
}
})
by which we can get the below result
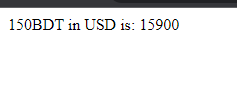
So, directives are a quick and easy solution for reusing the same function again and again in a concise way. And you can find out more directive hooks here.
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐