Implementing a Drag-and-Drop Feature with JavaScript
Hello dev's, today I'll show you how to implement a drag-and-drop feature using JS, without using any library like jQuery or Dropzone which will allows users to click and drag items on a web page or an specific container. So, let's see how we can easily implementing a drag-and-drop feature.
Here we we'll write some CSS code and JS code to manipulate a html content. So, look at the below source code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Implementing a drag-and-drop feature with JavaScript - shouts.dev</title>
<style>
#drag-item {
width: 100px;
height: 50px;
background-color: #007bff;
color: #fff;
text-align: center;
line-height: 50px;
cursor: move;
}
#drop-zone {
width: 200px;
height: 200px;
border: 2px dashed #ccc;
margin-top: 20px;
}
</style>
</head>
<body>
<div id="drag-item" draggable="true">
Drag me!
</div>
<div id="drop-zone">
Drop here!
</div>
<script>
const dragItem = document.getElementById("drag-item");
const dropZone = document.getElementById("drop-zone");
// Add event listeners for the drag and drop events
dragItem.addEventListener("dragstart", handleDragStart);
dropZone.addEventListener("dragover", handleDragOver);
dropZone.addEventListener("drop", handleDrop);
// Define the dragstart event handler
function handleDragStart(event) {
// Set the data to be transferred
event.dataTransfer.setData("text/plain", event.target.id);
}
// Define the dragover event handler
function handleDragOver(event) {
// Prevent the default action
event.preventDefault();
}
// Define the drop event handler
function handleDrop(event) {
// Prevent the default action
event.preventDefault();
// Get the data from the transfer
const data = event.dataTransfer.getData("text/plain");
// Move the dragged item to the drop zone
const draggedItem = document.getElementById(data);
event.target.appendChild(draggedItem);
}
</script>
</body>
</html>
Here, we're adding event listeners to the drag item and drop zone elements. When the user drags the drag item, we're setting the data to be transferred using the setData() method. When the user drops the drag item on the drop zone, we're getting the data from the transfer using the getData() method and moving the dragged item to the drop zone using the appendChild() method.
So if we open our html file in our browser then we can find the below output.
In initial condition
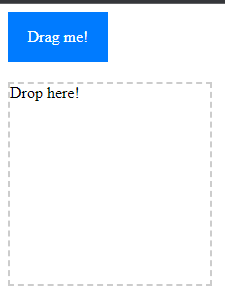
After being dragged
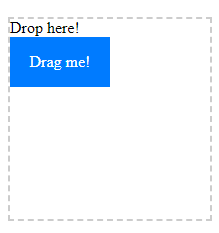
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐