JavaScript - Get Length of Array/Object/Arrays of Object
Hello dev's, Toady I'll show you how to count the length of an array or object or arrays of object. this is one of the main fundamental things we need to know when we are working of arrays and objects. Especially while we are working on a framework (vue js, react js) it's quite necessary to count the arrays of objects. So, let's see how we can easily count our object/array in a pure javascript way.
Count array or object
First we'll see the below code snippet
<html>
<head>
<title>Count array or object in javascript - shouts.dev</title>
</head>
<body>
<script>
var array = [1, 2, 3];
console.log("length of given array is = "+array.length);
let object = {
id : 1,
name : "Tanvir Ahmed",
email : "[email protected]",
phone : "+8801631102838",
website : "shouts.dev"
};
console.log('length of given object is = '+Object.keys(object).length);
let array_of_objects = [
{
id : 1,
name : "Tanvir Ahmed",
email : "[email protected]",
phone : "+8801631102838",
website : "shouts.dev"
},
{
id : 2,
name : "Tanvir Ahmed Hera",
email : "[email protected]",
phone : "+8801631102838",
website : "shouts.dev"
},
{
id : 3,
name : "Tanvir Ahmed Hera",
email : "[email protected]",
phone : "+8801631102838",
website : "shouts.dev"
}
];
console.log('length of given Array of objects is = '+array_of_objects.length);
</script>
</body>
</html>
It'll produce the below output.
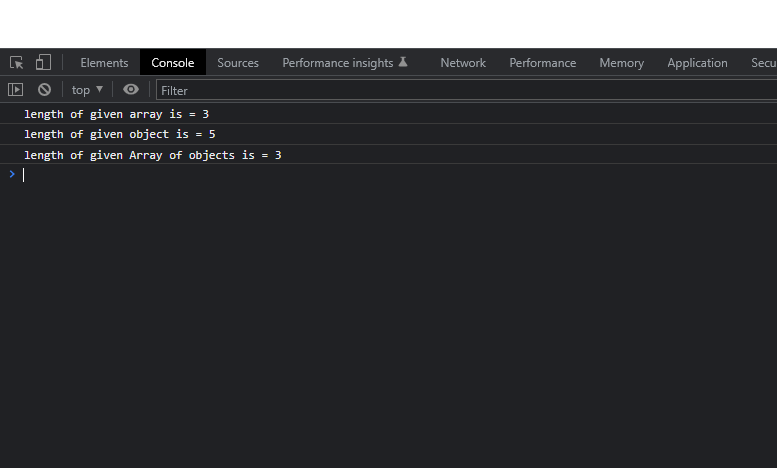
That's it for today. Hope you've enjoyed this tutorial. If you've any questions or face any difficulty, catch me in the comment section. Thanks for reading. ๐