JavaScript Create Dropdown using JS & CSS
Hello dev's, today I'll show you how to create a dropdown using JS & CSS only, without using any framework like bootstrap. So, let's see how we can easily create a dropdown using JS & CSS only.
Here we we'll write some CSS code and JS code to manipulate a html content. So, look at the below source code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Creating Dropdown with JS and CSS - shouts.dev</title>
<style>
.dropdown {
position: relative;
display: inline-block;
}
.dropdown-toggle {
background-color: #f1f1f1;
color: black;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
}
.dropdown-menu {
position: absolute;
top: 100%;
left: 0;
display: none;
z-index: 1;
}
.dropdown-option {
background-color: #f1f1f1;
color: black;
padding: 10px;
font-size: 16px;
cursor: pointer;
}
.dropdown-option:hover {
background-color: #ddd;
}
.show {
display: block;
}
</style>
</head>
<body>
<div class="dropdown">
<div class="dropdown-toggle">Select an option</div>
<div class="dropdown-menu">
<div class="dropdown-option">Option 1</div>
<div class="dropdown-option">Option 2</div>
<div class="dropdown-option">Option 3</div>
</div>
</div>
<script>
const dropdownToggle = document.querySelector('.dropdown-toggle');
const dropdownMenu = document.querySelector('.dropdown-menu');
dropdownToggle.addEventListener('click', function() {
dropdownMenu.classList.toggle('show');
});
const dropdownOptions = document.querySelectorAll('.dropdown-option');
dropdownOptions.forEach(function(option) {
option.addEventListener('click', function() {
console.log(`Selected option: ${option.textContent}`);
dropdownMenu.classList.remove('show');
});
});
</script>
</body>
</html>
Here, we have created a div element with the class "dropdown" to serve as the container for our dropdown menu. Within this container, we have added two child elements: a div element with the class "dropdown-toggle" to serve as the button that displays the menu options when clicked, and a div element with the class "dropdown-menu" to serve as the container for our menu options.
We have also added CSS styles to our dropdown menu and menu options to make them look and behave like a typical dropdown menu. The dropdown-menu class has the display property set to "none" by default, and the show class has the display property set to "block". When the dropdown-toggle is clicked, the show class is toggled on and off for the dropdown-menu, making it appear and disappear.
Finally, we have added event listeners to our dropdown-toggle and dropdown-options elements using JavaScript. When the dropdown-toggle is clicked, it toggles the show class for the dropdown-menu. And it mostly appears like a dropdown menu.
So if we open our html file in our browser then we can find the below output.
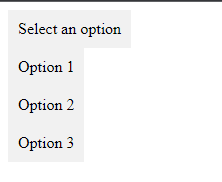
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐