Vue Toasted Notification Tutorial with Example
In this guide, I'm going to show how to make toast notification in VueJS using vue-toasted. Here's the project's output:
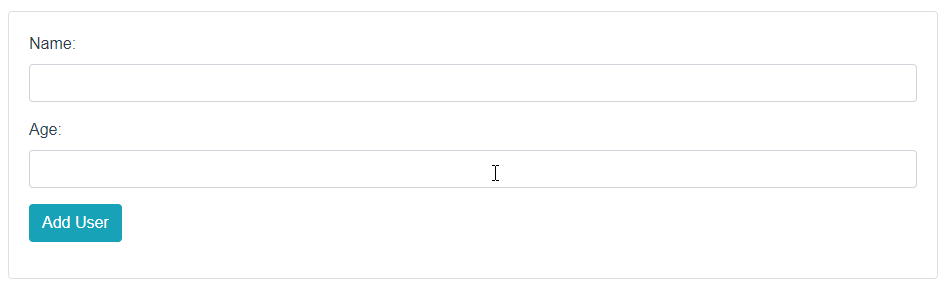
Now let's follow these steps:
Table of Contents
- Install Vue CLI and Create a Project
- Install vue-toasted
- Install Axios
- Install Bootstrap & Create Form
- Run the Project
Step 1 : Install Vue CLI and Create a Project
To install Vue CLI globally run this command:
# using npm:
npm install -g @vue/cli
# using yarn:
yarn global add @vue/cli
After installing Vue CLI, let's create a vue.js project using this command:
vue create vuenotification
Then navigate to your project directory:
cd vuenotification
Step 2 : Install vue-toasted
I'm going to install this package using NPM. Here's the command:
npm install vue-toasted --save
Open main.js from src folder and import vue-toasted like this:
import Toasted from 'vue-toasted'
Vue.use(Toasted, {
duration: 9000,
position: 'top-right',
action : {
text : 'Okay',
onClick : (e, toastObject) => {
toastObject.goAway(0);
}
}
})
To get more vue-toasted attributes, please see their official GitHub page.
Step 3 : Install Axios
Using Axios we will send POST request to the server like CURL. Let's install this:
npm install vue-axios axios --save
Import Axios like vue-toasted:
import VueAxios from 'vue-axios'
import axios from 'axios'
Vue.use(VueAxios, axios)
Step 4 : Install Bootstrap & Create Form
Install Bootstrap using this command:
npm install bootstrap --save
Import Bootstrap like vue-toasted:
// boostrap
import 'bootstrap/dist/css/bootstrap.css')
Let's create a simple form to add user. Go to src/components
folder and make a file called AddUser.vue. Then open the newly created file and paste the below code:
<template>
<div class="container">
<div class="card">
<div class="card-body">
<form @submit.prevent="addUser">
<div class="form-group">
<label>Name:</label>
<input type="text" class="form-control" v-model="user.name"/>
</div>
<div class="form-group">
<label>Age:</label>
<input type="text" class="form-control" v-model="user.age"/>
</div>
<div class="form-group">
<input type="submit" class="btn btn-info" value="Add User"/>
</div>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
user: {}
}
},
methods: {
addUser() {
let uri = 'https://reqres.in/api/users';
this.axios.post(uri, this.user).then((response) => {
console.log(response.data);
this.$toasted.show("User successfully added..!");
});
}
}
}
</script>
In this file, we have created a simple form to add user. and we have sent the form data to the server via POST method using Axios.
I've tested using a dummy API called https://reqres.in
. You can use your own API here.
We need to import AddUser.vue in App.vue file. Open src/App.vue file and import AddUser like this:
<template>
<div id="app">
<AddUser/>
</div>
</template>
<script>
import AddUser from './components/AddUser.vue'
export default {
name: 'app',
components: {
AddUser
}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
color: #2c3e50;
margin-top: 30px;
}
</style>
Step 5 : Run the Project
So, the main.js file looks like:
import Vue from 'vue'
import App from './App.vue'
// boostrap
import 'bootstrap/dist/css/bootstrap.css'
// axios
import VueAxios from 'vue-axios'
import axios from 'axios'
Vue.use(VueAxios, axios)
// vue toasted
import Toasted from 'vue-toasted'
Vue.use(Toasted, {
duration: 9000,
position: 'top-right',
action : {
text : 'Okay',
onClick : (e, toastObject) => {
toastObject.goAway(0);
}
}
})
Vue.config.productionTip = false
new Vue({
render: h => h(App),
}).$mount('#app')
Now run the project using the below command and see the output.
npm run serve
The tutorial is over. You can download this project from GitHub. Thank you.Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.