Laravel 10 Filter Collection Items using where()
Laravel Collection's where() method allows you to easily filter items in a collection by specifying a key-value pair. It checks if the specified key has a value equal to the provided value. You can also use the optional $operator parameter to customize how the comparison is done. The where() method then gives you a new collection containing only the items that meet your criteria.
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project laravel/laravel laravel_filtering
The signature of where()
method in the Illuminate/Support/Collection class looks something like below.
**
* Filter items by the given key value pair.
*
* @param string $key
* @param mixed $operator
* @param mixed $value
* @return static
*/
public function where($key, $operator = null, $value = null)
{
...
}
Let's use the where method to narrow down our collection.
<?php
namespace App\Http\Controllers;
use Illuminate\Foundation\Auth\Access\AuthorizesRequests;
use Illuminate\Foundation\Validation\ValidatesRequests;
use Illuminate\Routing\Controller as BaseController;
class Controller extends BaseController
{
use AuthorizesRequests, ValidatesRequests;
public function index(){
$collection = collect([
[
'name' => 'Luke Thomas',
'job' => 'Marketing Manager',
'salary' => 8000
],
[
'name' => 'Liam Coleman',
'job' => 'Web Manager',
'salary' => 7500
],
[
'name' => 'Jacob Hill',
'job' => 'Web Developer',
'salary' => 6500
],
[
'name' => 'Charles Brown',
'job' => 'Web Developer',
'salary' => 5000
],
[
'name' => 'Richard Wilson',
'job' => 'Web Developer',
'salary' => 5400
],
[
'name' => 'Isaac Kelly',
'job' => 'Graphics Designer',
'salary' => 6000
]
]);
$filteredCollection = $collection->where('salary', '>', 6000);
dd($filteredCollection);
}
}
To find employees with salaries over $6000, we can filter the records using the where() method.
The above statement will return the response to something like below.
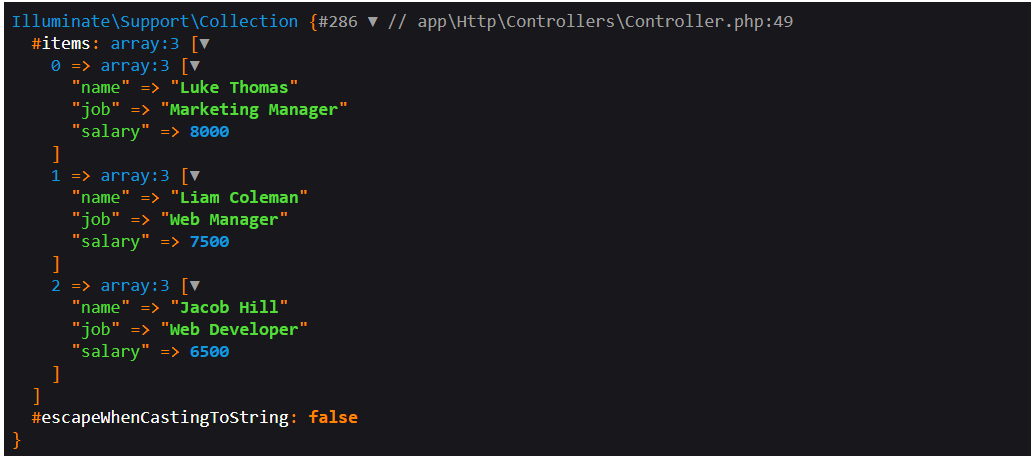
We're going to narrow down our original collection, $collection, to show only the employees who have the job title "web developer."
<?php
namespace App\Http\Controllers;
use Illuminate\Foundation\Auth\Access\AuthorizesRequests;
use Illuminate\Foundation\Validation\ValidatesRequests;
use Illuminate\Routing\Controller as BaseController;
class Controller extends BaseController
{
use AuthorizesRequests, ValidatesRequests;
public function index(){
$collection = collect([
[
'name' => 'Luke Thomas',
'job' => 'Marketing Manager',
'salary' => 8000
],
[
'name' => 'Liam Coleman',
'job' => 'Web Manager',
'salary' => 7500
],
[
'name' => 'Jacob Hill',
'job' => 'Web Developer',
'salary' => 6500
],
[
'name' => 'Charles Brown',
'job' => 'Web Developer',
'salary' => 5000
],
[
'name' => 'Richard Wilson',
'job' => 'Web Developer',
'salary' => 5400
],
[
'name' => 'Isaac Kelly',
'job' => 'Graphics Designer',
'salary' => 6000
]
]);
$filteredCollection = $collection->where('job', 'Web Developer');
dd($filteredCollection);
}
}
You will be presented with something like the below output.
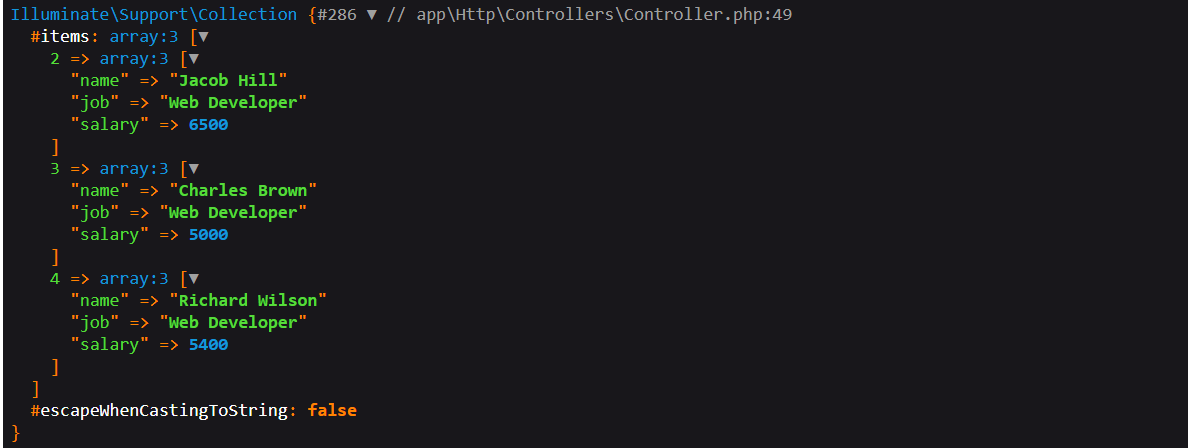
You may have noticed that in the earlier examples, we initially used all three arguments: $key, $operator, and $value. However, as we proceeded, we only provided $key and $value. If we don’t provide any $operator
Laravel will set the operator to =
.
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂