Laravel Generate PDF and Attach to Email
Hello artisans, in this article I’m going to share how to generate PDF and attach it to the email. I’m testing on Laravel 8.9.0. Let’s get started:
Table of Contents
- Install Laravel and Basic Configurations
- Install Package & Config
- Create Controller
- Create PDF View
- Define Routes
Install Laravel and Basic Configurations
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Install Package & Config
At first we need to install barryvdh/laravel-dompdf
package. Run this composer command to install the package:
composer require barryvdh/laravel-dompdf
Now open .env file and set your SMTP credentials:
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=null
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
MAIL_FROM_ADDRESS=null
MAIL_FROM_NAME="${APP_NAME}"
Create Controller
Create a test controller and make a function to send email:
<?php
namespace App\Http\Controllers;
use PDF;
use Mail;
class TestController extends Controller
{
public function sendMailWithPDF()
{
$data["email"] = "[email protected]";
$data["title"] = "Welcome to MyNotePaper";
$data["body"] = "This is the email body.";
$pdf = PDF::loadView('mail', $data);
Mail::send('mail', $data, function ($message) use ($data, $pdf) {
$message->to($data["email"], $data["email"])
->subject($data["title"])
->attachData($pdf->output(), "test.pdf");
});
dd('Email has been sent successfully');
}
}
Create PDF View
Now we’ll create the PDF view which will be attached to the email. Go to resources>views folder and create mail.blade.php
file. Then paste this simple PDF view:
<!DOCTYPE html>
<html>
<head>
<title>MyNotePaper</title>
</head>
<body>
<h3>{{ $title }}</h3>
<p>{{ $body }}</p>
<p>
Regards,<br/>
mynotepaper.com
</p>
</body>
</html>
Define Routes
Open routes/web.php
file and add following route:
use App\Http\Controllers\TestController;
use Illuminate\Support\Facades\Route;
Route::get('send-email', [TestController::class, 'sendMailWithPDF']);
Now run the project:
php artisan serve
Visit the send email route:
http://localhost:8000/send-email
The output:
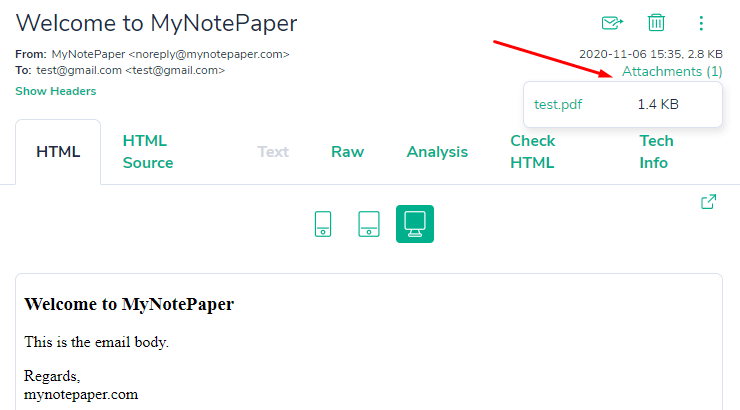
The tutorial is over. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.