Laravel 10 Laravel withSum() with Where Condition Example
Hello Artisan, today I'll show you how to use Laravel withSum() with Where Condition. Welcome to a brief guide on using the withSum() method in Laravel along with conditions. This guide will walk you through a straightforward example of utilizing withSum() within Laravel's Eloquent relationships. Let's get started!
We'll focus on understanding how to employ withSum() alongside a condition. In this demonstration, we'll work with a scenario involving Categories and Products. By establishing relationships and applying a condition, we'll explore how to efficiently utilize withSum().
This approach is applicable across various Laravel versions, including 6, 7, 8, 9, and 10. Now, let's move ahead and delve into the specifics.
Creating the Category and Product Model, you can proceed by executing the following command
php artisan make:Model Category -m
php artisan make:Model Product -m
Let's see example with output:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Category extends Model
{
use HasFactory;
protected $fillable = [
'name', 'is_active'
];
public function products()
{
return $this->hasMany(Product::class);
}
}
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
use HasFactory;
protected $fillable = [
'name','price', 'is_active'
];
}
<?php
namespace App\Http\Controllers;
use App\Models\Category;
use Illuminate\Http\Request;
class ProductsController extends Controller
{
public function index()
{
$categories = Category::select("id", "name")
->withSum('products', 'price')
->get()
->toArray();
dd($categories);
}
}
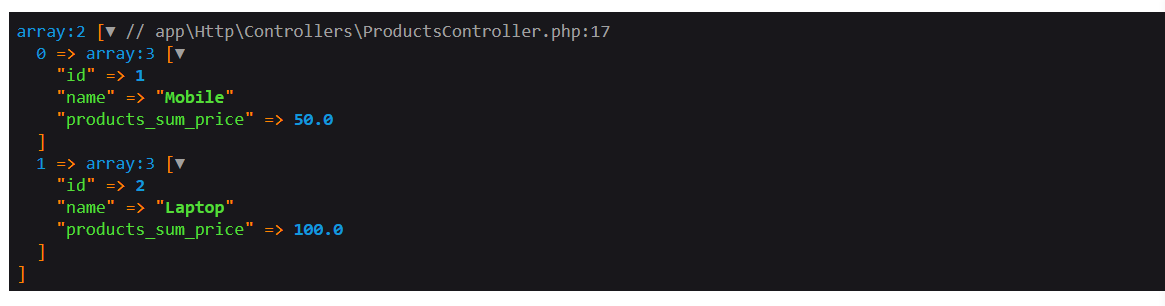
<?php
namespace App\Http\Controllers;
use App\Models\Category;
use Illuminate\Http\Request;
class ProductsController extends Controller
{
public function index()
{
$categories = Category::select("id", "name")
->withSum([
'products' => function ($query) {
$query->where('is_active', '1');
}], 'price')
->get()
->toArray();
dd($categories);
}
}
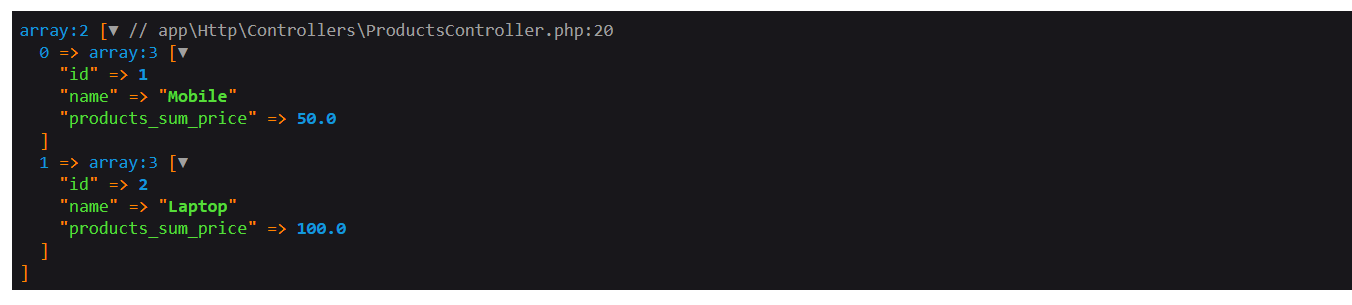
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. ๐