Laravel 6 CRUD (Create Read Update Delete) From Scratch
Today I’m going to show the CRUD operations for Laravel 6. It is a simple Laravel CRUD project for beginners.
Table of Contents
- Install Laravel and Basic Configurations
- Create Table
- Create Controller and Model
- Setup Resource Route
- Create Blade Template and Files
- The Output
Step 1 : Install Laravel and Basic Configurations
Each Laravel project needs this thing. That’s why I have written an article on this topic. Please see this part from here: Install Laravel and Basic Configurations.
Step 2 : Create Table
We are going to add “products” table. Run this command to create the products migration file:
php artisan make:migration create_products_table --create=products
Navigate to database/migrations
and you will see the migration file for creating products table. Open the file and update the up()
function like this:
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('name');
$table->string('price');
$table->text('detail');
$table->timestamps();
});
}
Let’s migrate the migration file using this command:
php artisan migrate
Step 3 : Create Controller and Model
Our table is ready. Now we are going create a controller named ‘ProductController‘ and a model named ‘Product ‘. Run this command to create both the controller and the model:
php artisan make:controller ProductController --resource --model=Product
Open the Product.php file from app
folder. We will add product name, price and details fields in $fillable
array like this:
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
protected $fillable = [
'name', 'price', 'detail'
];
}
In this step, we will write CRUD operations in the ProductController. Open ProductController from app/Http/Controllers
directory. You can see 7 functions which are empty. Remove all codes and paste this code to the ProductController file:
<?php
namespace App\Http\Controllers;
use App\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$products = Product::latest()->paginate(5);
return view('products.index',compact('products'))
->with('i', (request()->input('page', 1) - 1) * 5);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('products.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'price' => 'required',
'detail' => 'required',
]);
Product::create($request->all());
return redirect()->route('products.index')
->with('success','Product created successfully.');
}
/**
* Display the specified resource.
*
* @param \App\Product $product
* @return \Illuminate\Http\Response
*/
public function show(Product $product)
{
return view('products.show',compact('product'));
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Product $product
* @return \Illuminate\Http\Response
*/
public function edit(Product $product)
{
return view('products.edit',compact('product'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Product $product
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Product $product)
{
$request->validate([
'name' => 'required',
'price' => 'required',
'detail' => 'required',
]);
$product->update($request->all());
return redirect()->route('products.index')
->with('success','Product updated successfully');
}
/**
* Remove the specified resource from storage.
*
* @param \App\Product $product
* @return \Illuminate\Http\Response
*/
public function destroy(Product $product)
{
$product->delete();
return redirect()->route('products.index')
->with('success','Product deleted successfully');
}
}
Step 4 : Setup Resource Route
Open web.php
from routes folder. Let’s create a resource route called products for the ProductController.
Route::resource('products','ProductController');
Step 5 : Create Blade Template and Files
Go to resources/views
directory and make a folder named ‘products‘. Now go to the resources/views/products
folder.
We are going to create 5 files here. Just create these files and copy-paste the codes:
<!DOCTYPE html>
<html>
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>Laravel 6 CRUD from Scratch</title>
<!-- Bootstrap CSS -->
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="text-center" style="margin: 50px 0 50px 0;"><a href="{{url("products")}}"><img
src="{{asset("images/logo.png")}}" alt="Logo"></a><br>Laravel 6 CRUD from Scratch
</div>
@yield('content')
</div>
</body>
</html>
@extends('products.layout')
@section('content')
<div class="row" style="margin-bottom: 20px;">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Products</h3>
</div>
<div class="pull-right">
<a class="btn btn-success" href="{{ route('products.create') }}">Add Product</a>
</div>
</div>
</div>
@if ($message = Session::get('success'))
<div class="alert alert-success">
<p>{{ $message }}</p>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
<th>Details</th>
<th width="280px">Actions</th>
</tr>
@foreach ($products as $product)
<tr>
<td>{{ $product->id }}</td>
<td>{{ $product->name }}</td>
<td>{{ $product->price }}</td>
<td>{{ $product->detail }}</td>
<td>
<form action="{{ route('products.destroy',$product->id) }}" method="POST">
<a class="btn btn-info" href="{{ route('products.show',$product->id) }}">Show</a>
<a class="btn btn-primary" href="{{ route('products.edit',$product->id) }}">Edit</a>
@csrf
@method('DELETE')
<button type="submit" class="btn btn-danger">Delete</button>
</form>
</td>
</tr>
@endforeach
</table>
{!! $products->links() !!}
@endsection
@extends('products.layout')
@section('content')
<div class="row" style="margin-bottom: 20px;">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Add Product</h3>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('products.store') }}" method="POST">
@csrf
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Name:</strong>
<input type="text" name="name" class="form-control">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Price:</strong>
<input type="text" name="price" class="form-control">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Detail:</strong>
<textarea class="form-control" style="height:150px" name="detail"></textarea>
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-success">Add Product</button>
</div>
</div>
</form>
@endsection
@extends('products.layout')
@section('content')
<div class="row" style="margin-bottom: 20px;">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Edit Product</h3>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('products.update',$product->id) }}" method="POST">
@csrf
@method('PUT')
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Name:</strong>
<input type="text" name="name" value="{{ $product->name }}" class="form-control">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Price:</strong>
<input type="text" name="price" value="{{ $product->price }}" class="form-control">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Detail:</strong>
<textarea class="form-control" style="height:150px" name="detail">{{ $product->detail }}</textarea>
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-success">Update</button>
</div>
</div>
</form>
@endsection
@extends('products.layout')
@section('content')
<div class="row" style="margin-bottom: 20px;">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>Show Product</h3>
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Name:</strong>
{{ $product->name }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Price:</strong>
{{ $product->price }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Details:</strong>
{{ $product->detail }}
</div>
</div>
</div>
@endsection
Step 6 : The Output
We have completed all the necessary steps. Our Laravel CRUD application is ready. Let’s run your application:
php artisan serve
Now visit your project URL from browser:
http://localhost/laravel6crud:8000/products
Here’s my project’s output:
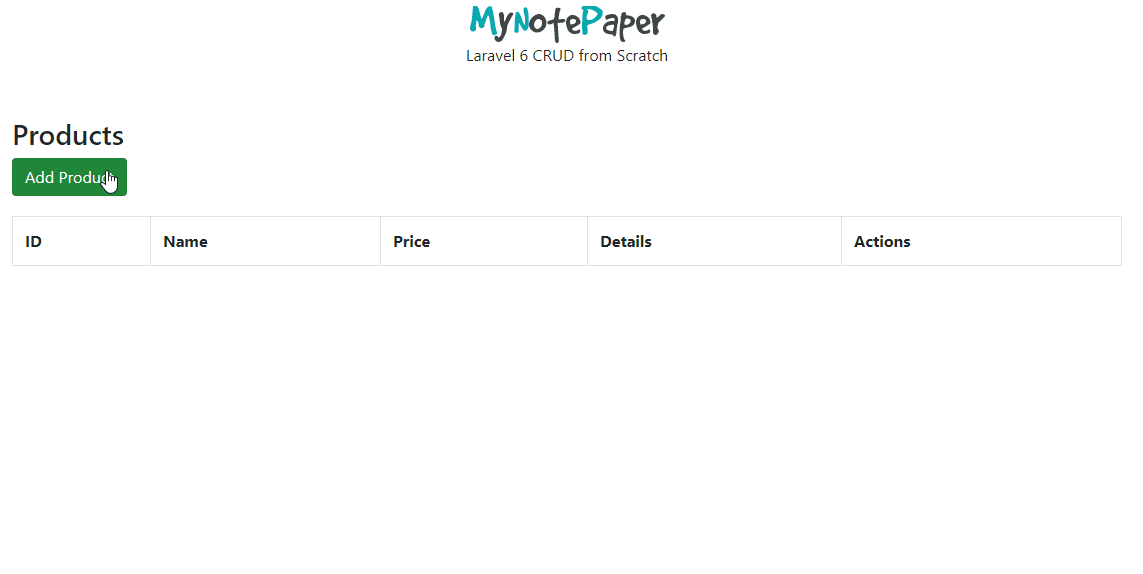
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.