Laravel Livewire CRUD with Example
Hello artisans, In this tutorial, I will show you the CRUD operation using Livewire with Bootstrap modal. Livewire is a full-stack framework for Laravel that makes constructing dynamic interfaces easy, without leaving the comfort of Laravel. So, let’s get dive into the topic.
Note: Tested on Laravel 8.65.
Table of Contents
Install Livewire
In this step, we will simply install the Livewire by firing the below command.
composer require livewire/livewire
Create and Configure Component
Creating a component is very easy. Just fire the below command
php artisan make:livewire user
This will create two files
- app/Http/Livewire/User.php
- resources/views/livewire/user.blade.php
Now we work on the component. So, open User.php and the paste below code into it
<?php
namespace App\Http\Livewire;
use Livewire\Component;
use App\Models\User as UserModel;
class User extends Component
{
public $name, $email, $user_id;
public $updateMode = false;
public function render()
{
$users = UserModel::all();
$data = [
'users' => $users
];
return view('livewire.user',$data);
}
private function resetInputFields()
{
$this->name = '';
$this->email = '';
}
public function store()
{
$this->validate([
'name' => 'required',
'email' => 'required|email',
]);
UserModel::create([
'name' => $this->name,
'email' => $this->email,
]);
session()->flash('message', 'Users Created Successfully.');
$this->resetInputFields();
$this->emit('userStore'); // Close model to using to jquery
}
public function edit($id)
{
$this->updateMode = true;
$user = UserModel::find($id);
$this->user_id = $id;
$this->name = $user->name;
$this->email = $user->email;
}
public function cancel()
{
$this->updateMode = false;
$this->resetInputFields();
}
public function update()
{
$this->validate([
'name' => 'required',
'email' => 'required|email',
]);
if ($this->user_id) {
$user = UserModel::find($this->user_id);
$user->update([
'name' => $this->name,
'email' => $this->email,
]);
$this->updateMode = false;
session()->flash('message', 'Users Updated Successfully.');
$this->resetInputFields();
}
}
public function delete($id)
{
if ($id) {
UserModel::destroy($id);
session()->flash('message', 'Users Deleted Successfully.');
}
}
}
Add Route
Put the below route in your web.php
Route::view('user','livewire.home');
Create and Configure View
At first we need to create the following blade files under resources/livewire
- home.blade.php
- create.blade.php
- update.blade.php
Now open these files and paste the below code to the corresponding files.
user.blade.php
<div>
@include('livewire.create')
@include('livewire.update')
@if (session()->has('message'))
<div class="alert alert-success" style="margin-top:30px;">x
{{ session('message') }}
</div>
@endif
<table class="table table-bordered mt-5">
<thead>
<tr>
<th>No.</th>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach($users as $value)
<tr>
<td>{{ $value->id }}</td>
<td>{{ $value->name }}</td>
<td>{{ $value->email }}</td>
<td>
<button data-toggle="modal" data-target="#updateModal" wire:click="edit({{ $value->id }})" class="btn btn-primary btn-sm">Edit</button>
<button wire:click="delete({{ $value->id }})" class="btn btn-danger btn-sm">Delete</button>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
home.blade.php
<html>
<head>
<title></title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<!-- Popper JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
@livewireStyles
</head>
<body>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">
<h2>Laravel Livewire Crud</h2>
</div>
<div class="card-body">
@if (session()->has('message'))
<div class="alert alert-success">
{{ session('message') }}
</div>
@endif
@livewire('user')
</div>
</div>
</div>
</div>
</div>
@livewireScripts
<script type="text/javascript">
window.livewire.on('userStore', () => {
$('#exampleModal').modal('hide');
});
</script>
</body>
</html>
create.blade.php
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#exampleModal">
Open Form
</button>
<!-- Modal -->
<div wire:ignore.self class="modal fade" id="exampleModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true close-btn">×</span>
</button>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<label for="exampleFormControlInput1">Name</label>
<input type="text" class="form-control" id="exampleFormControlInput1" placeholder="Enter Name" wire:model="name">
@error('name') <span class="text-danger error">{{ $message }}</span>@enderror
</div>
<div class="form-group">
<label for="exampleFormControlInput2">Email address</label>
<input type="email" class="form-control" id="exampleFormControlInput2" wire:model="email" placeholder="Enter Email">
@error('email') <span class="text-danger error">{{ $message }}</span>@enderror
</div>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary close-btn" data-dismiss="modal">Close</button>
<button type="button" wire:click.prevent="store()" class="btn btn-primary close-modal">Save changes</button>
</div>
</div>
</div>
</div>
update.blade.php
<div wire:ignore.self class="modal fade" id="updateModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<input type="hidden" wire:model="user_id">
<label for="exampleFormControlInput1">Name</label>
<input type="text" class="form-control" wire:model="name" id="exampleFormControlInput1" placeholder="Enter Name">
@error('name') <span class="text-danger">{{ $message }}</span>@enderror
</div>
<div class="form-group">
<label for="exampleFormControlInput2">Email address</label>
<input type="email" class="form-control" wire:model="email" id="exampleFormControlInput2" placeholder="Enter Email">
@error('email') <span class="text-danger">{{ $message }}</span>@enderror
</div>
</form>
</div>
<div class="modal-footer">
<button type="button" wire:click.prevent="cancel()" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="button" wire:click.prevent="update()" class="btn btn-primary" data-dismiss="modal">Save changes</button>
</div>
</div>
</div>
</div>
Output
If you go to the http://127.0.0.1:8000/user, you will find the below output
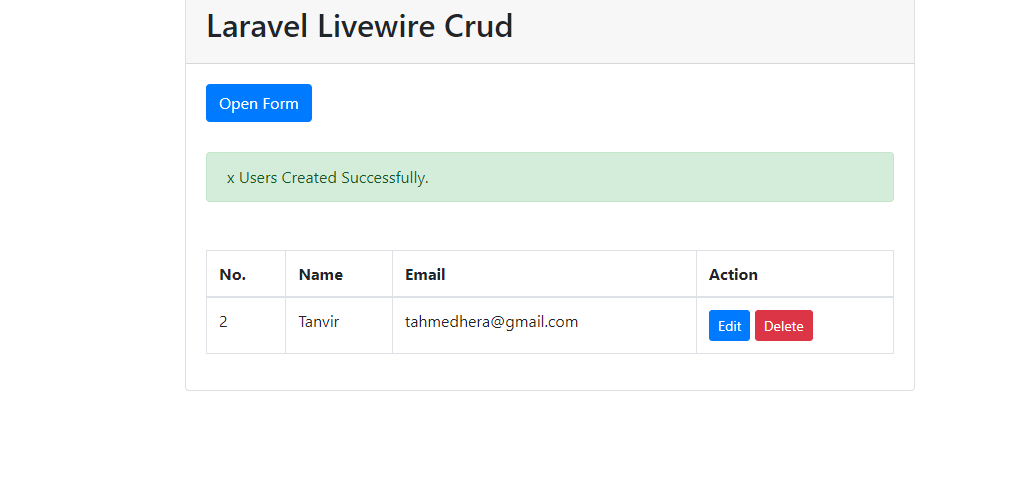
That’s all for today. You can download the project from GitHub also. Thanks for reading.