Laravel 10 How to Generate a Dropdown List of Timezone in Laravel
Hello Artisan, today I'll show you how to create Dropdown List of Timezone in our Laravel application.In this tutorial, we will demonstrate how to create a Laravel seeder to populate a database table with a list of timezones. The seeder will store each timezone's name, offset, and name, offset and diff_from_gtm fields. To achieve this, we will leverage the timezone_identifiers_list() function, which provides a list of all available time zones.
Step-by-step, we'll create a Laravel seeder that runs on database seeding, allowing us to store the timezone data efficiently. By the end of this tutorial, you'll have a select box in your Laravel application containing a comprehensive list of timezones, ready to be used in various parts of your project.
Let's get started and explore how to implement this in a straightforward and easy-to-understand manner.
- Install Laravel App
- Create Migration and Model
- Create Seeder Class
- Create Controller
- Create Route
- Create View File
- Output
Creating the Laravel app is not necessary for this step, but if you haven't done it yet, you can proceed by executing the following command
composer create-project laravel/laravel Timezone
Feel free to ignore this step if you've already created the Laravel app.
Let's create a new Model and migration to add a table called 'timezones' with the required fields. So let's run bellow command
php artisan make:Model Timezone -m
After this command you will find one file in following path "database/migrations". In this file, paste the following code to create the 'timezones' table.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('timezones', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('offset');
$table->string('diff_from_gtm');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('timezones');
}
};
To run this migration, use the following command:
php artisan migrate
Next, After create “timezones” table you should create Timezone model for “timezones table”, so first create file in this path app/Models/Timezone.php and put bellow content in Timezone.php file
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Timezone extends Model
{
use HasFactory;
protected $fillable = [
'name', 'offset', 'diff_from_gtm'
];
}
Next, we'll make a new seeder called “TimezoneSeeder” to store all the “timezones” in it.
php artisan make:seeder TimezoneSeeder
Further, put the below code in database\seeders\TimezoneTableSeeder.php
<?php
namespace Database\Seeders;
use App\Models\Timezone;
use Illuminate\Database\Seeder;
class TimezoneSeeder extends Seeder
{
/**
* Run the database seeds.
*/
public function run(): void
{
$timestamp = time();
foreach (timezone_identifiers_list() as $zone) {
date_default_timezone_set($zone);
$zones['offset'] = date('P', $timestamp);
$zones['diff_from_gtm'] = 'UTC/GMT '.date('P', $timestamp);
Timezone::updateOrCreate(['name' => $zone], $zones);
}
}
}
Now, we will run seeder commands
php artisan db:seed --class=TimezoneSeeder
Here's a simplified version of the code to create the new TimezoneController with the index method for displaying a form
<?php
namespace App\Http\Controllers;
use App\Models\Timezone;
class TimezoneController extends Controller
{
public function index()
{
$timezones = Timezone::Orderby('offset')->get();
return view('timezone.timezonelist', compact('timezones'));
}
}
In the “routes/web.php” file, add a route to display a list of all timezones.
<?php
use App\Http\Controllers\TimezoneController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider and all of them will
| be assigned to the "web" middleware group. Make something great!
|
*/
Route::get('timezone-list', [TimezoneController::class, 'index']);
In Last step, let's create timezonelist.blade.php for display timezone list and put following code
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Laravel Generate Timezone List in Dropdown List </title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.3.0/css/bootstrap.min.css"/>
</head>
<body>
<div class="container mt-4">
<h2>Laravel Generate Timezone List in Dropdown List </h2>
<form>
<div class="mb-3">
<label for="exampleInputEmail1" class="form-label">Select Timezone:</label>
<select class="form-control mb-2" name="timezone_id">
<option value="">Please select...</option>
@foreach($timezones as $timezone)
<option value="{{ $timezone->id }}">{{ $timezone->name }} ({{ $timezone->offset }})</option>
@endforeach
</select>
</div>
</form>
</div>
</body>
</html>
To run the Laravel app, simply type the following command and press Enter:
php artisan serve
Now, Go to your web browser, type the given URL and view the app output:
http://localhost:8000/timezone-list
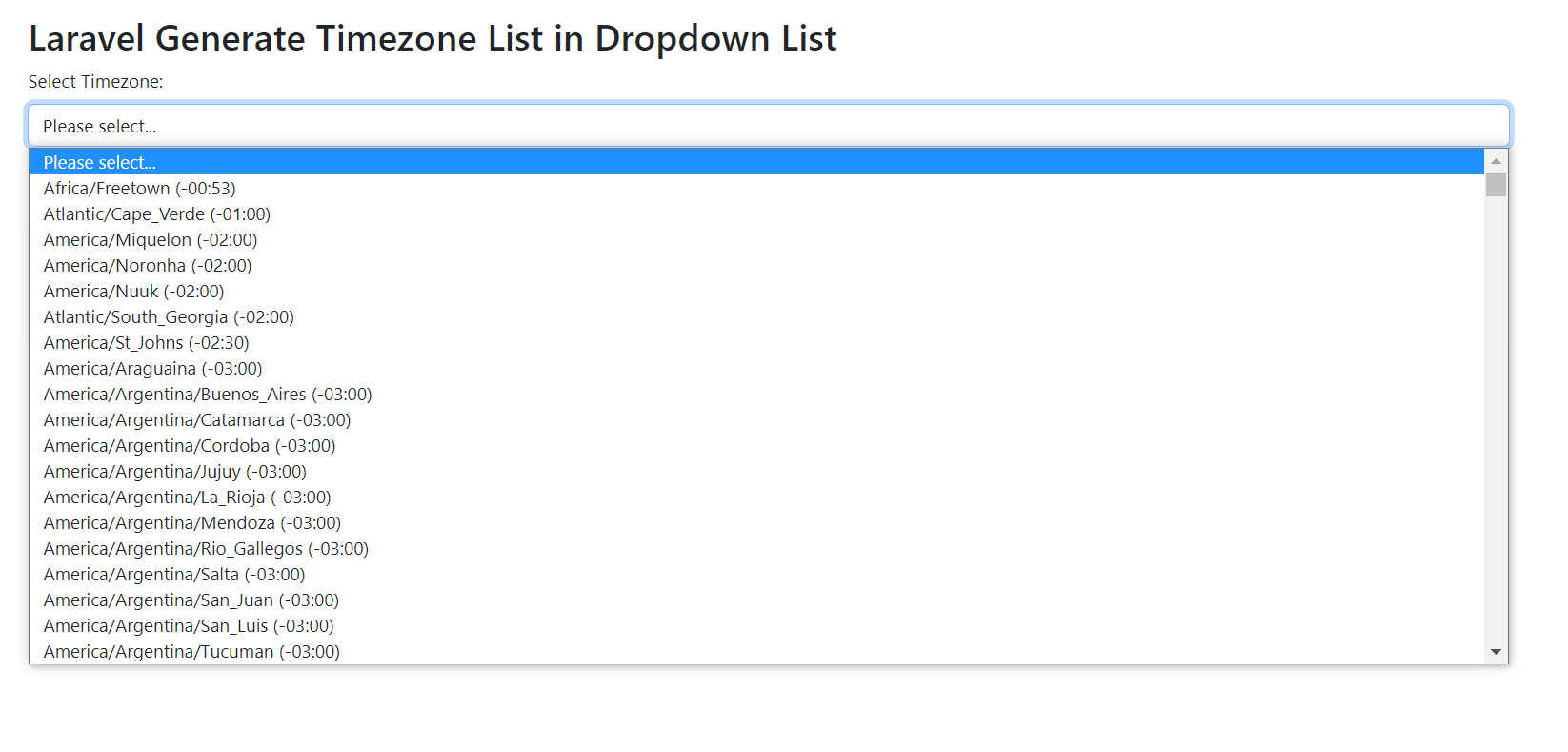
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. 🙂