Create Custom Laravel 404 Error Page
Today I’m going to show how to use custom 404 not found error page in Laravel. We can do it very easily.
Create Error Page
Navigate to resources>views and create a folder named ‘errors‘. Now go to the errors page.
We have to make a file called ‘404.blade.php‘. Let’s create the file in the errors folder.
So, the final path of the file is resources>views/errors/404.blade.php. Now open the 404.blade.php file and paste your custom 404-page template. I’m placing a demo 404 template. You can paste this code to test the demo template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Create Custom Laravel 404 Error Page</title>
<!-- Google font -->
<link href="https://fonts.googleapis.com/css?family=Kanit:200" rel="stylesheet">
<!-- Font Awesome Icon -->
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.8.2/css/all.css">
<!-- Custom stlylesheet -->
<style>
*{-webkit-box-sizing:border-box;box-sizing:border-box}body{padding:0;margin:0}#notfound{position:relative;height:100vh}#notfound .notfound{position:absolute;left:50%;top:50%;-webkit-transform:translate(-50%,-50%);-ms-transform:translate(-50%,-50%);transform:translate(-50%,-50%)}.notfound{max-width:767px;width:100%;line-height:1.4;text-align:center;padding:15px}.notfound .notfound-404{position:relative;height:220px}.notfound .notfound-404 h1{font-family:'Kanit',sans-serif;position:absolute;left:50%;top:50%;-webkit-transform:translate(-50%,-50%);-ms-transform:translate(-50%,-50%);transform:translate(-50%,-50%);font-size:186px;font-weight:200;margin:0;background:linear-gradient(130deg,#ffa34f,#ff6f68);color:transparent;-webkit-background-clip:text;background-clip:text;text-transform:uppercase}.notfound h2{font-family:'Kanit',sans-serif;font-size:33px;font-weight:200;text-transform:uppercase;margin-top:0;margin-bottom:25px;letter-spacing:3px}.notfound p{font-family:'Kanit',sans-serif;font-size:16px;font-weight:200;margin-top:0;margin-bottom:25px}.notfound a{font-family:'Kanit',sans-serif;color:#ff6f68;font-weight:200;text-decoration:none;border-bottom:1px dashed #ff6f68;border-radius:2px}.notfound-social>a{display:inline-block;height:40px;line-height:40px;width:40px;font-size:14px;color:#ff6f68;border:1px solid #efefef;border-radius:50%;margin:3px;-webkit-transition:.2s all;transition:.2s all}.notfound-social>a:hover{color:#fff;background-color:#ff6f68;border-color:#ff6f68}@media only screen and (max-width: 480px){.notfound .notfound-404{position:relative;height:168px}.notfound .notfound-404 h1{font-size:142px}.notfound h2{font-size:22px}}
</style>
</head>
<body>
<div id="notfound">
<div class="notfound">
<div class="notfound-404">
<h1>404</h1>
</div>
<h2>Oops! Nothing was found</h2>
<p>The page you are looking for might have been removed had its name changed or is temporarily unavailable. <a href="https://shouts.dev/">Return to homepage</a></p>
<div class="notfound-social">
<a href="#"><i class="fab fa-facebook"></i></a>
<a href="#"><i class="fab fa-twitter"></i></a>
<a href="#"><i class="fab fa-pinterest"></i></a>
<a href="#"><i class="fab fa-google-plus"></i></a>
</div>
</div>
</div>
</body>
</html>
The Output
Now run the project and visit a wrong page to see the output. Here’s my output:
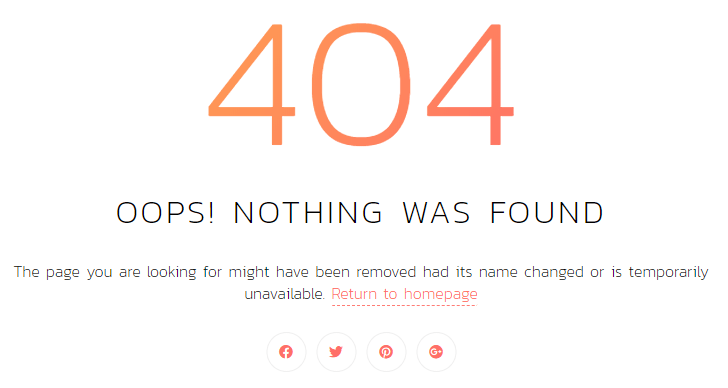
We have successfully created a custom 404 template in Laravel.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.