Vue.js CLI Fetch Data from API with Axios
In this tutorial, we are going to learn how to fetch data from a third-party API using Axios.
Table of Contents
- Create a Vue CLI project
- Install Necessary Dependencies
- Add Bootstrap to Project
- Import Axios and Create Get Method
- The Final App.vue File
- Add ESLint File to Ignore Errors
- Run and Test
Step 1 : Create a Vue CLI Project
Go to your projects directory and create a project by typing this:
vue create vuejs-cli-api-get-request
Go to the folder:
cd vuejs-cli-api-get-request
Step 2 : Install Necessary Dependencies
At first, let’s install Axios by typing this command:
npm install axios --save
For front-end, we are going to install bootstrap, jQuery, and popper.js. We can install these three by one command:
npm install bootstrap jquery popper.js --save
Step 3 : Add Bootstrap to Project
We have already installed Bootstrap in our project. Open main.js from src folder using an editor and add these two lines:
import 'bootstrap'
import 'bootstrap/dist/css/bootstrap.min.css'
So, the main.js looks like:
import Vue from 'vue'
import App from './App.vue'
import 'bootstrap'
import 'bootstrap/dist/css/bootstrap.min.css'
Vue.config.productionTip = false
new Vue({
render: h => h(App),
}).$mount('#app')
Step 4 : Import Axios and Create Get Method
Go to src folder and open App.vue file. We are going to import axios and create a get method. We are going to take dummy data from
https://reqres.in/api/users?page=1.
<script>
import axios from "axios";
export default {
name: 'app',
data () {
return {
loading: false,
all_users: null
}
},
mounted () {
this.loading = true;
axios
.get('https://reqres.in/api/users?page=1')
.then(response => (this.all_users = response.data.data))
.catch(error => console.log(error))
.finally(() => this.loading = false)
}
}
</script>
Step 5 : The Final App.vue File
We have completed our javascript part. Let’s bind to html. So, the final App.vue looks like:
<template>
<div id="app" class="container">
<p v-if="loading">Loading...</p>
<div v-else>
<h3 class="heading">Users</h3>
<table class="table table-bordered">
<thead>
<tr>
<th scope="col">ID</th>
<th scope="col">Avatar</th>
<th scope="col">Name</th>
<th scope="col">Email</th>
</tr>
</thead>
<tbody>
<tr v-for="user in all_users" v-bind:key="user">
<td>{{ user.id }}</td>
<td><img v-bind:src="user.avatar" width="50"/></td>
<td>{{ user.first_name + " " + user.last_name }}</td>
<td>{{ user.email }}</td>
</tr>
</tbody>
</table>
</div>
</div>
</template>
<script>
import axios from "axios";
export default {
name: 'app',
data () {
return {
loading: false,
all_users: null
}
},
mounted () {
this.loading = true;
axios
.get('https://reqres.in/api/users?page=1')
.then(response => (this.all_users = response.data.data))
.catch(error => console.log(error))
.finally(() => this.loading = false)
}
}
</script>
<style>
#app {
text-align: center;
margin-top: 50px;
}
.heading {
margin-bottom: 30px;
}
</style>
Step 6 : Add ESLint File to Ignore Errors
As we aren’t using webpack for this project, we have to do it manually. In the root folder create a file named “.eslintrc.js“. Then open it and paste this:
module.exports = {
parser: 'babel-eslint',
parserOptions: {
sourceType: 'module'
ecmaFeatures: {
experimentalObjectRestSpread: true
}
},
extends: [
'plugin:vue/recommended'
],
rules: {
"comma-dangle": 0,
"no-unused-vars": "warn",
"no-console": 1,
"no-mixed-spaces-and-tabs": 0,
"no-unexpected-multiline": "warn"
}
}
Step 7 : Run and Test
Now let’s run the app:
npm run serve
Visit the project URL http://localhost:8080
and see the output:
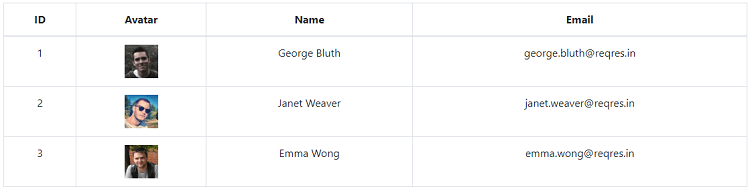
You can download this project from GitHub.
Our project is done.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.