Use Word Captcha Code and Validation in Laravel
Hello Artisans, today I'll show how we can integrate captcha code and validation in our Laravel Application. For that, we will use captcha-com/laravel-captcha. Let's see how we can use it in our application.
Note: Tested on Laravel 9.2.
Table of Contents
- Install and setup captcha-com/laravel-captcha
- Create and Setup Controller
- Define Routes
- Create and Setup blade File
- Output
Install and setup captcha-com/laravel-captcha
Fire the below command in terminal to install laravel captcha package.
composer require mews/captcha
Now we'll register service provider and alias in config/app.php.
'providers' => [
....
Mews\Captcha\CaptchaServiceProvider::class
],
'aliases' => [
....
'Captcha' => Mews\Captcha\Facades\Captcha::class
],
Create and Setup Controller
Now we'll create a controller where we'll write our logic to show the validation messages and for refresh the captcha code. So, fire the below command in the terminal
php artisan make:controller HomeController
Now put the below code in your HomeController.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function myCaptcha()
{
return view('captcha');
}
public function myCaptchaPost(Request $request)
{
$request->validate([
'email' => 'required|email',
'password' => 'required',
'captcha' => 'required|captcha'
],
['captcha.captcha' => 'Invalid captcha code.']);
dd("You are here :) .");
}
public function refreshCaptcha(): \Illuminate\Http\JsonResponse
{
return response()->json(['captcha'=> captcha_img()]);
}
}
Define Routes
Now we will define routes for refresh our captcha code and post a request form. Put these below routes in your web.php.
Route::get('my-captcha', [\App\Http\Controllers\HomeController::class,'myCaptcha'])->name('myCaptcha');
Route::post('my-captcha', [\App\Http\Controllers\HomeController::class,'myCaptchaPost'])->name('myCaptcha.post');
Route::get('refresh_captcha', [\App\Http\Controllers\HomeController::class,'refreshCaptcha'])->name('refresh_captcha');
Create and Setup blade File
Now first we'll create a blade file called captcha.blade.php where we'll put our form and show the validation messages. So, create a file under the resources/views/captcha.blade.php and put the below codes.
<html lang="en">
<head>
<title>How to create captcha code in Laravel 9?</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
<link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet"
integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
</head>
<body>
<div class="container" style="margin-top: 50px">
<div class="col-md-8 col-md-offset-2">
<div class="panel panel-default">
<div class="panel-heading">Login</div>
<div class="panel-body">
<form class="form-horizontal" method="POST" action="{{ route('myCaptcha.post') }}">@csrf
<div class="form-group{{ $errors->has('email') ? ' has-error' : '' }}">
<label for="email" class="col-md-4 control-label">E-Mail Address</label>
<div class="col-md-6">
<input id="email" type="text" class="form-control" name="email" value="{{ old('email') }}">
@if ($errors->has('email'))
<span class="help-block">
<strong>{{ $errors->first('email') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group{{ $errors->has('password') ? ' has-error' : '' }}">
<label for="password" class="col-md-4 control-label">Password</label>
<div class="col-md-6">
<input id="password" type="password" class="form-control" name="password">
@if ($errors->has('password'))
<span class="help-block">
<strong>{{ $errors->first('password') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group{{ $errors->has('captcha') ? ' has-error' : '' }}">
<label for="password" class="col-md-4 control-label">Captcha</label>
<div class="col-md-6">
<div class="captcha">
<span>{!! captcha_img() !!}</span>
<button type="button" class="btn btn-success btn-refresh"><i class="fa fa-refresh"></i>
</button>
</div>
<input id="captcha" type="text" class="form-control" placeholder="Enter Captcha"
name="captcha">
@if ($errors->has('captcha'))
<span class="help-block">
<strong>{{ $errors->first('captcha') }}</strong>
</span>
@endif
</div>
</div>
<div class="form-group">
<div class="col-md-8 col-md-offset-4">
<button type="submit" class="btn btn-primary">
Submit
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</body>
<script type="text/javascript">
$(".btn-refresh").click(function () {
$.ajax({
type: 'GET',
url: '/refresh_captcha',
success: function (data) {
$(".captcha span").html(data.captcha);
}
});
});
</script>
</html>
Output
Now we ready with our setup. It's time to check our output. If everything goes well you'll find a below output
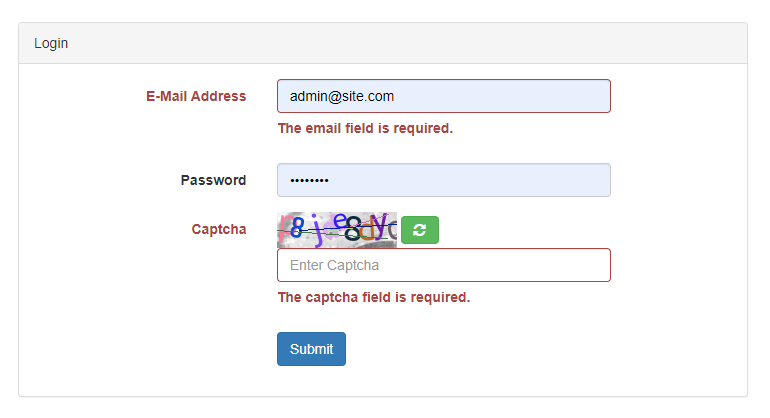
That's it for today. Hope you'll enjoy this tutorial. You can also download this tutorial from GitHub. Thanks for reading. 🙂