Laravel Import Inputted Data on JSON File and Download It
Hello Artisans, today I'll teach you about how you can create an json file from inputted data through <form>. As all of we know that json format is accepted by globally in each of every stack. So, it can be quite helpful to retrieve data in json file. So, let's see how we can accomplish our result with just few steps.
Note: Tested on Laravel 9.19
Frist, we'll create a controller called JsonFileController.php where we'll write our logic to create an json file first then put the request date into json file. So, fire the below command in terminal to generate controller first.
php artisan make:controller JsonFileController
Now we'll do add logic to our controller. See the below source code.
class JsonFileController extends Controller
{
public function downloadJson(Request $request): \Symfony\Component\HttpFoundation\BinaryFileResponse
{
$data = $request->except('_token');
$json = json_encode($data);
$file = Str::uuid().'.json';
$path = public_path($file);
file_put_contents($path, $json);
return response()->download($path);
}
}
Now we'll add routes which will connect our <form> with our controller. So, let's add the below route to our web.php.
Route::post('download-json',[App\Http\Controllers\JsonFileController::class, 'downloadJson'])->name('download.json');
Now we'll build a form which data we want to export into json file. For that we'll use welcome.blade.php which comes with laravel by default. So, let's see the below source code.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Download JSON File</title>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row justify-content-center">
<div class="col-lg-8">
<h1>Import Requested Data into JSON File and Download It</h1>
<form action="{{ route('download.json') }}" method="POST">@csrf
<div class="row">
<div class="col-lg-12 form-group">
<label for="name">Name</label>
<input type="text" name="name" id="name" class="form-control">
</div>
<div class="col-lg-12 form-group">
<label for="email">Email</label>
<input type="email" name="email" id="email" class="form-control">
</div>
<div class="col-lg-12 form-group">
<label for="phone">Phone</label>
<input type="text" name="phone" id="phone" class="form-control">
</div>
<div class="col-lg-12 form-group">
<label for="address">Address</label>
<input type="text" name="address" id="address" class="form-control">
</div>
<div class="col-lg-12">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</form>
</div>
</div>
</div>
<!-- jQuery library -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/jquery.slim.min.js"></script>
<!-- Popper JS -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
And finally, we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000, If everything goes well (hope so) we can see the below output.
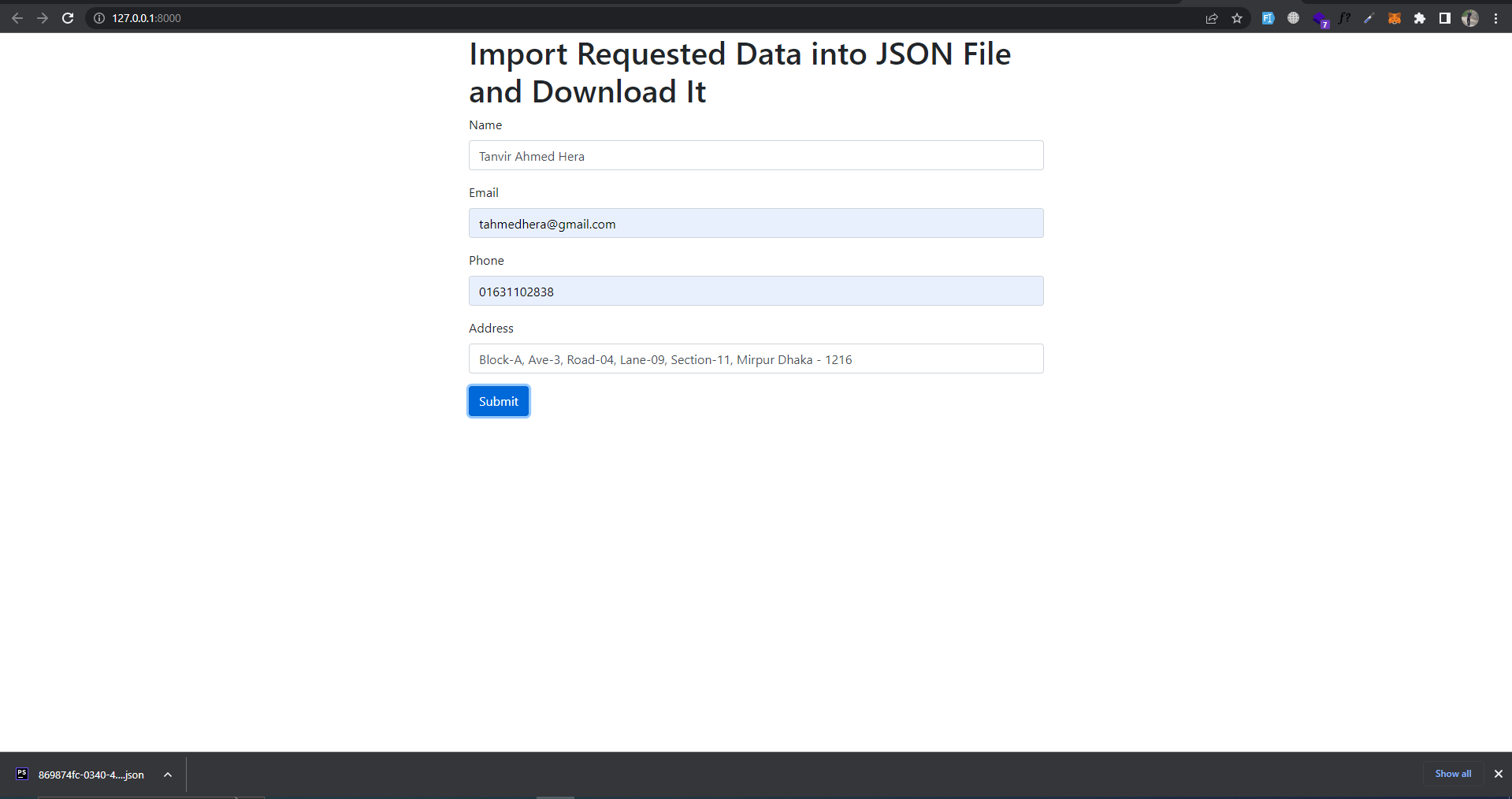
And as all we know that our .json file structure will look like below.
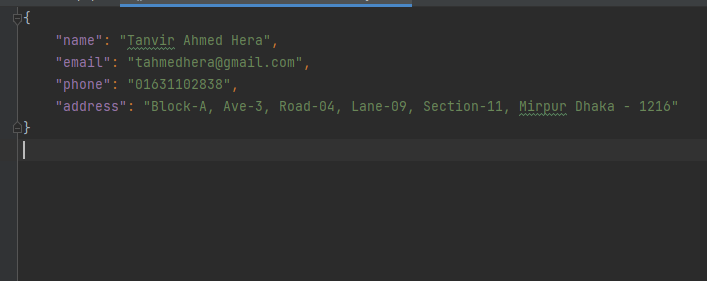
That's it for today. I hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐