How to Get Only Unique Values From Array in JavaScript
Hello dev's, today I'll show you How to Get Only Unique Values From Array in JavaScript. I'll guide you through a simple tutorial on how to extract unique values from a JavaScript array. We'll cover the concept of getting distinct values from an array of objects in JavaScript. By the end, you'll know how to obtain all the unique values effectively in JavaScript.
There are several ways to get only unique values from an array in JavaScript:
1. Using the Set object: The Set object in JavaScript is a tool for holding only unique values of different types. You can change an array into a Set using the spread operator, and then turn it back into an array using the Array.from() method.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to Get Only Unique Values From Array in JavaScript? - shouts.dev</title>
</head>
<body>
</body>
<script type="text/javascript">
const arr = [1, 2, 3, 2, 4, 3];
const uniqueArr = Array.from(new Set(arr));
console.log(uniqueArr); // [1, 2, 3, 4]
</script>
</html>
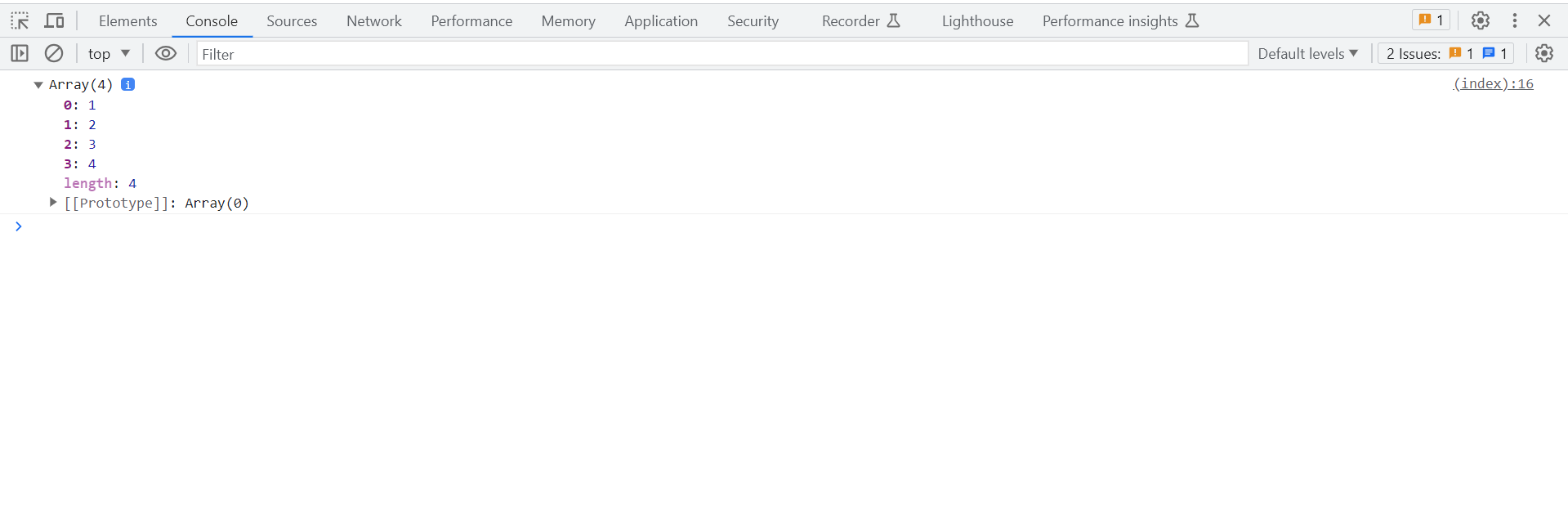
2. Using the filter() method: The Set object in JavaScript is like a special box where you can put things, but each thing has to be unique. If you have a list of things (an array), you can turn it into this special box using the spread operator. And if you want to turn the special box back into a list, you can use the Array.from() trick.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to Get Only Unique Values From Array in JavaScript? - shouts.dev</title>
</head>
<body>
</body>
<script type="text/javascript">
const arr = [1, 2, 3, 2, 4, 3];
const uniqueArr = arr.filter((value, index) => {
return arr.indexOf(value) === index;
});
console.log(uniqueArr); // [1, 2, 3, 4]
</script>
</html>
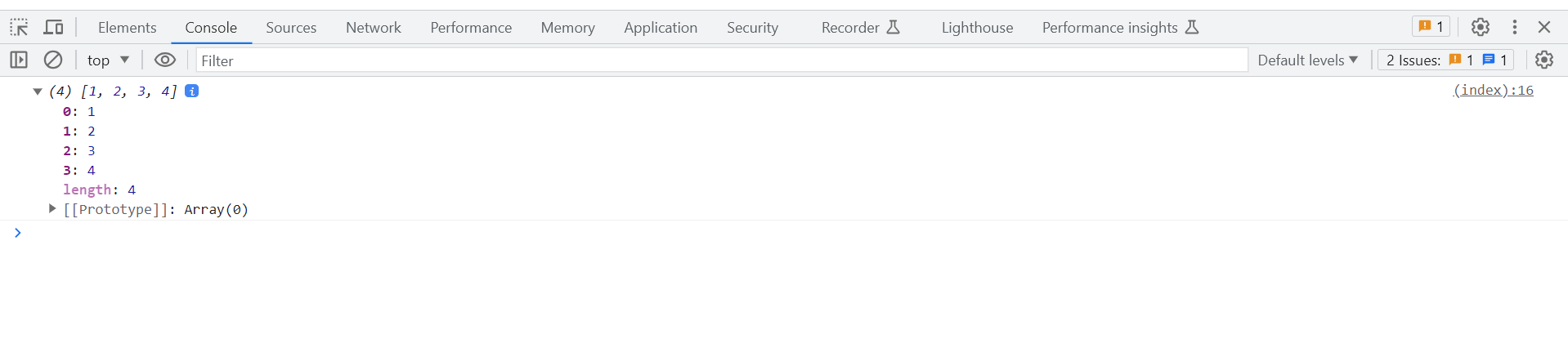
3. Using the reduce() method: You can use the reduce() method to make a new list that contains only distinct values. It works by comparing each value to the ones already in the new list.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to Get Only Unique Values From Array in JavaScript? - shouts.dev</title>
</head>
<body>
</body>
<script type="text/javascript">
const arr = [1, 2, 3, 2, 4, 3];
const uniqueArr = arr.reduce((accumulatorArray , currentValue) => {
if(!accumulatorArray.includes(currentValue)){
accumulatorArray.push(currentValue);
}
return accumulatorArray;
}, []);
console.log(uniqueArr); // [1, 2 ,3 ,4]
</script>
</html>
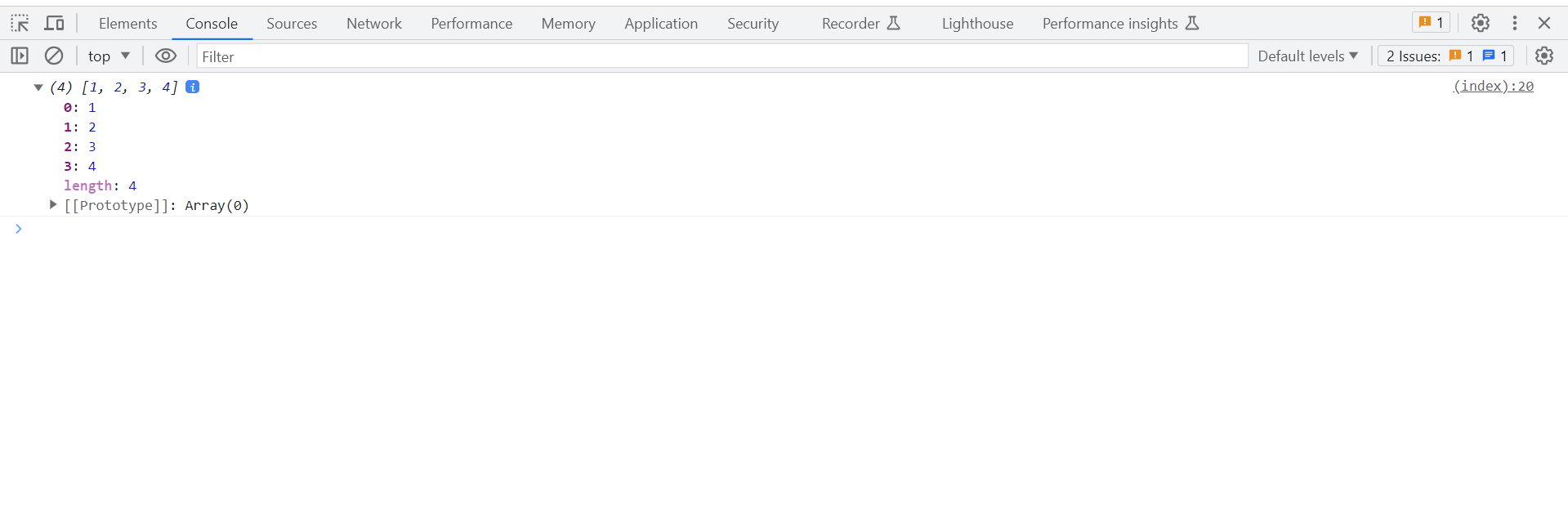
That's it for today. I hope it'll be helpful in upcoming project. Thanks for reading. ๐