Simple Tags Input with Tagify in Tailwind CSS
In this tutorial, we'll create input tags using Tailwind CSS, we can also use it with search dropdown. To create input tags we'll use tagify(tag.js). Let's see how we can easily create input tags using Tailwind CSS and tagify (tag.js). We'll see 3 examples below
Here we'll see the simple input tags
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Tailwind CSS- Input Tags Example</title>
<link
href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css"
rel="stylesheet"
/>
<link
href="https://unpkg.com/@yaireo/tagify/dist/tagify.css"
rel="stylesheet"
type="text/css"
/>
</head>
<body>
<div class="flex items-center justify-center mt-20">
<div class="w-1/2">
<label for="Tags" class="block mb-2 text-sm text-gray-600">Tags</label>
<input
type="text"
class="w-full px-4 py-6 text-sm border border-gray-300 rounded outline-none"
name="tags"
value="VueJs, Tailwind CSS, PHP8.0"
autofocus
/>
</div>
</div>
<script src="https://unpkg.com/@yaireo/tagify"></script>
<script src="https://unpkg.com/@yaireo/tagify/dist/tagify.polyfills.min.js"></script>
<script>
// The DOM element you wish to replace with Tagify
var input = document.querySelector('input[name=tags]');
// initialize Tagify on the above input node reference
new Tagify(input);
</script>
</body>
</html>
Which will produce the below result

Here we'll see input tags with dropdown and suggestions.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Tailwind CSS - Input Tags Example</title>
<link
href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css"
rel="stylesheet"
/>
<link
href="https://unpkg.com/@yaireo/tagify/dist/tagify.css"
rel="stylesheet"
type="text/css"
/>
</head>
<body>
<div class="flex items-center justify-center mt-20">
<div class="w-1/2">
<label for="Tags" class="block mb-2 text-sm text-gray-600">Tags</label>
<input
type="text"
class="w-full px-4 py-6 text-sm border border-gray-300 rounded outline-none "
name="tags"
value="Tailwind CSS, VueJs, PHP8"
autofocus
/>
</div>
</div>
<script src="https://unpkg.com/@yaireo/tagify"></script>
<script src="https://unpkg.com/@yaireo/tagify/dist/tagify.polyfills.min.js"></script>
<script>
// The DOM element you wish to replace with Tagify
var input = document.querySelector('input[name=tags]');
var tagify = new Tagify(input, {
addTagOnBlur: false,
dropdown: {
enabled: 0,
closeOnSelect: false,
},
whitelist: [
'PHP',
'NodeJs',
'Laravel',
'VueJs'
],
templates: {
dropdownItem(item) {
return `<div ${this.getAttributes(item)}
class='tagify__dropdown__item ${item.class ? item.class : ''}'
tabindex="0"
role="option">
${item.value}
<button tabindex="0" data-value="${
item.value
}" class="ml-4 text-red-800">Remove</button>
</div>`;
},
},
hooks: {
suggestionClick(e) {
var isAction = e.target.classList.contains('removeBtn'),
suggestionElm = e.target.closest('.tagify__dropdown__item'),
value = suggestionElm.getAttribute('value');
return new Promise(function (resolve, reject) {
if (isAction) {
removeWhitelistItem(value);
tagify.dropdown.refilter.call(tagify);
reject();
}
resolve();
});
},
},
});
function removeWhitelistItem(value) {
var index = tagify.settings.whitelist.indexOf(value);
if (value && index > -1) tagify.settings.whitelist.splice(index, 1);
}
</script>
</body>
</html>
Which will produce the below result

Here we'll see the input tags with suggestions in dropdown as well as when a user wants to remove any tag, It'll ask the user for confirmation.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Tailwind CSS - Input Tags Example</title>
<link
href="https://unpkg.com/tailwindcss@^2/dist/tailwind.min.css"
rel="stylesheet"
/>
<link
href="https://unpkg.com/@yaireo/tagify/dist/tagify.css"
rel="stylesheet"
type="text/css"
/>
</head>
<body>
<div class="flex items-center justify-center mt-20">
<div class="w-1/2">
<label for="Tags" class="block mb-2 text-sm text-gray-600">Tags</label>
<input
type="text"
class="w-full px-4 py-6 text-sm border border-gray-300 rounded outline-none "
name="tags"
value="Tailwind CSS, VueJs"
autofocus
/>
</div>
</div>
<script src="https://unpkg.com/@yaireo/tagify"></script>
<script src="https://unpkg.com/@yaireo/tagify/dist/tagify.polyfills.min.js"></script>
<script>
// The DOM element you wish to replace with Tagify
var input = document.querySelector('input[name=tags]');
var tagify = new Tagify(input, {
addTagOnBlur: false,
dropdown: {
enabled: 0,
closeOnSelect: false,
},
whitelist: [
'VueJs',
'PHP',
'NodeJs',
'Laravel',
],
templates: {
dropdownItem(item) {
return `<div ${this.getAttributes(item)}
class='tagify__dropdown__item ${item.class ? item.class : ''}'
tabindex="0"
role="option">
${item.value}
<button tabindex="0" data-value="${
item.value
}" class="ml-4 text-red-800">Remove</button>
</div>`;
},
},
hooks: {
suggestionClick(e) {
var isAction = e.target.classList.contains('removeBtn'),
suggestionElm = e.target.closest('.tagify__dropdown__item'),
value = suggestionElm.getAttribute('value');
return new Promise(function (resolve, reject) {
if (isAction) {
removeWhitelistItem(value);
tagify.dropdown.refilter.call(tagify);
reject();
}
resolve();
});
},
beforeRemoveTag: (tags) => {
tags[0].node.classList.add('tagify__tag');
return new Promise((resolve, reject) => {
confirm(`Are you sure to delete ${tags[0].data.value} tag ?`)
? resolve()
: reject();
});
},
},
});
function removeWhitelistItem(value) {
var index = tagify.settings.whitelist.indexOf(value);
if (value && index > -1) tagify.settings.whitelist.splice(index, 1);
}
</script>
</body>
</html>
And here is our outcome for the above code.
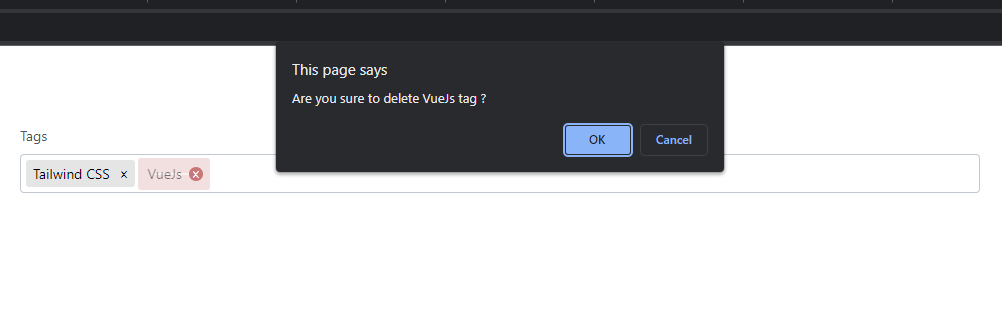
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐