JavaScript Build an Image Gallery with CSS & JavaScript
Hello dev's, today I'll show you how to create an image gallery with CSS & JavaScript, without using any framework/library like bootstrap or any other jQuery plugin. So, let's see how we can easily create an image gallery.
Here we we'll write some CSS code and JS code to manipulate a html content. So, look at the below source code
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Building a Image Gallery with CSS & JavaScript - shouts.dev</title>
<style>
.gallery {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
padding: 10px;
}
.gallery img {
width: calc(25% - 10px);
margin-bottom: 10px;
}
.modal {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.9);
}
.modal img {
display: block;
max-width: 90%;
max-height: 90%;
margin: auto;
margin-top: 50px;
}
</style>
</head>
<body>
<div class="gallery">
<img src="img1.jpg" alt="Image 1">
<img src="img2.jpg" alt="Image 2">
<img src="img3.jpg" alt="Image 3">
<img src="img4.jpg" alt="Image 4">
</div>
<div class="modal">
<img src="" alt="">
</div>
<script>
const gallery = document.querySelector('.gallery');
const modal = document.querySelector('.modal');
const modalImage = document.querySelector('.modal img');
gallery.addEventListener('click', function(event) {
if (event.target.tagName === 'IMG') {
modalImage.src = event.target.src;
modal.style.display = 'block';
}
});
modal.addEventListener('click', function() {
modal.style.display = 'none';
});
</script>
</body>
</html>
Here, we have created a div element with the class "gallery" to serve as the container for our image gallery. Within this container, we have added four “img” elements to serve as our gallery images.
We have also added CSS styles to our gallery container and images to make them responsive. The gallery class has display set to "flex" and flex-wrap set to "wrap", allowing our images to wrap to a new row when they reach the end of the container. We have also set the width of our images to 25% of the container width minus 10 pixels to create a margin between the images.
Finally, we have added event listeners to our gallery images and modal using JavaScript. When an image is clicked, the event listener checks if the target element is an “img” element, sets the “src” attribute of the modal Image to the “src” of the clicked image, and displays the image in a full window like we can see a image in gallery by taping/clicking.
So if we open our html file in our browser then we can find the below output.
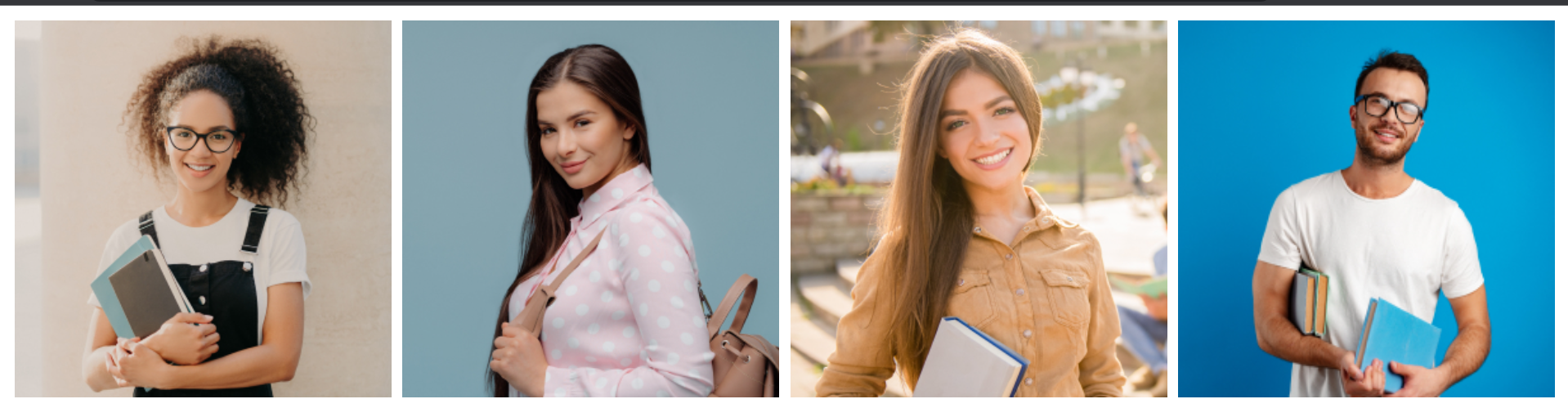
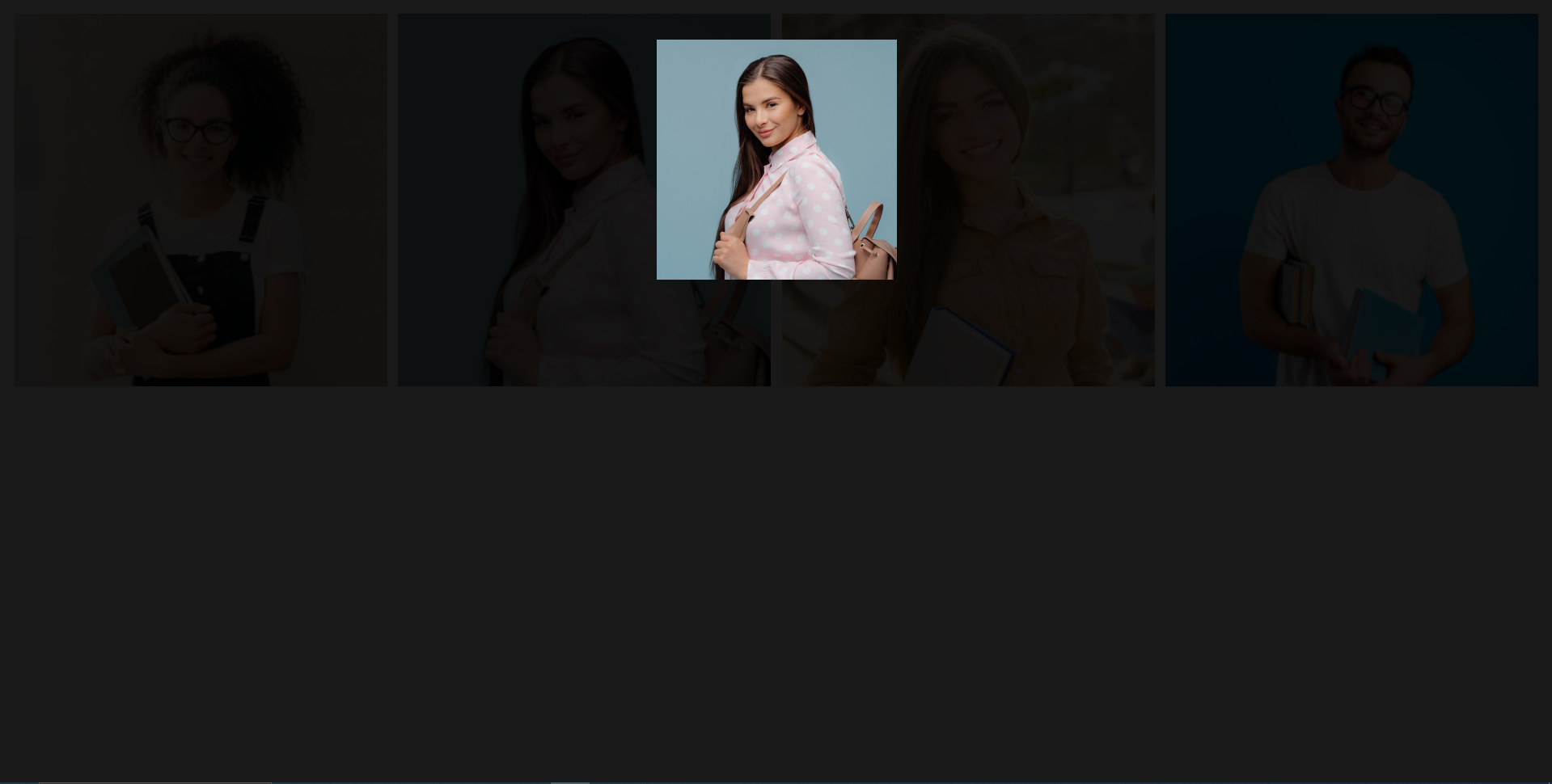
This image height and width is depend on your image ratio.
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. 🙂