Laravel to Set Dynamic Page Title for Each Page
Hello Artisans, today I'll show you how to set dynamic page title in each and every page which user visits. It's quite helpful to show the title of the page to the user for the user friendly behavior and also user can see in which page currently they are. So, let's see how we can easily set the page title in which page user visits.
Note: Tested on Laravel 9.19.
Table of Contents
Create and Setup Blade Files
First of all we'll create 4 blade files
- master.blade.php
- index.blade.php
- create.blade.php
- update.blade.php
Now open the corresponding file and paste the below codes
master.blade.php
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>shouts.dev - @yield('page_title')</title>
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
here @yeild('page_title') is mainly responsible for dynamic page title. Which we later use a @section in every page where we need to set the title of the page.
index.blade.php
@extends('master')
@section('page_title', 'Article List')
@section('content')
<h4 style="text-align: center">Index Page for Article List</h4>
@endsection
create.blade.php
@extends('master')
@section('page_title', 'Create Article')
@section('content')
<h4 style="text-align: center">Create Page for Article List</h4>
@endsection
update.blade.php
@extends('master')
@section('page_title', 'Update Article')
@section('content')
<h4 style="text-align: center">Update Page for Article List</h4>
@endsection
Create Routes
Let's put the below route in web.php
Route::get('articles', function (){
return view('article.index');
});
Route::get('create-article', function (){
return view('article.create');
});
Route::get('edit-article', function (){
return view('article.update');
});
Output
And finally we're ready with our setup. It's time to check our output. Now go to http://localhost:8000/articles, http://localhost:8000/create-article and http://localhost:8000/edit-article, If everything goes well (hope so) you can find a below output.
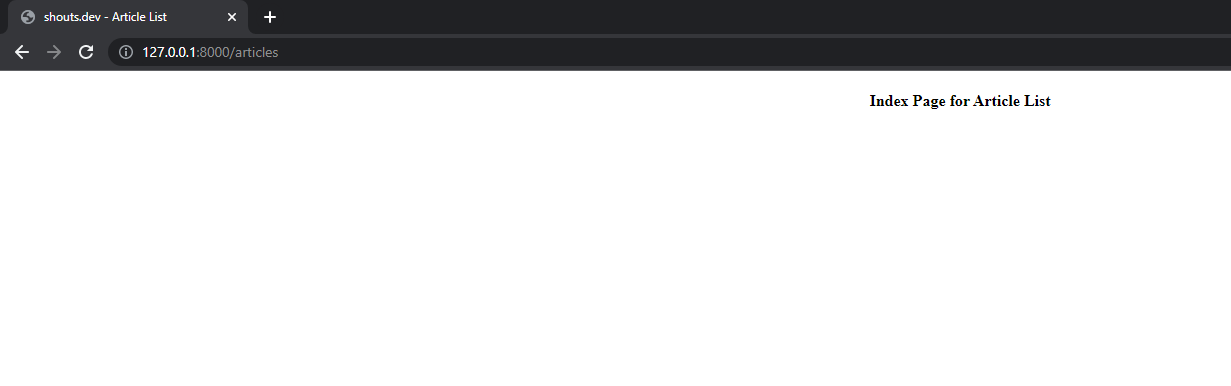
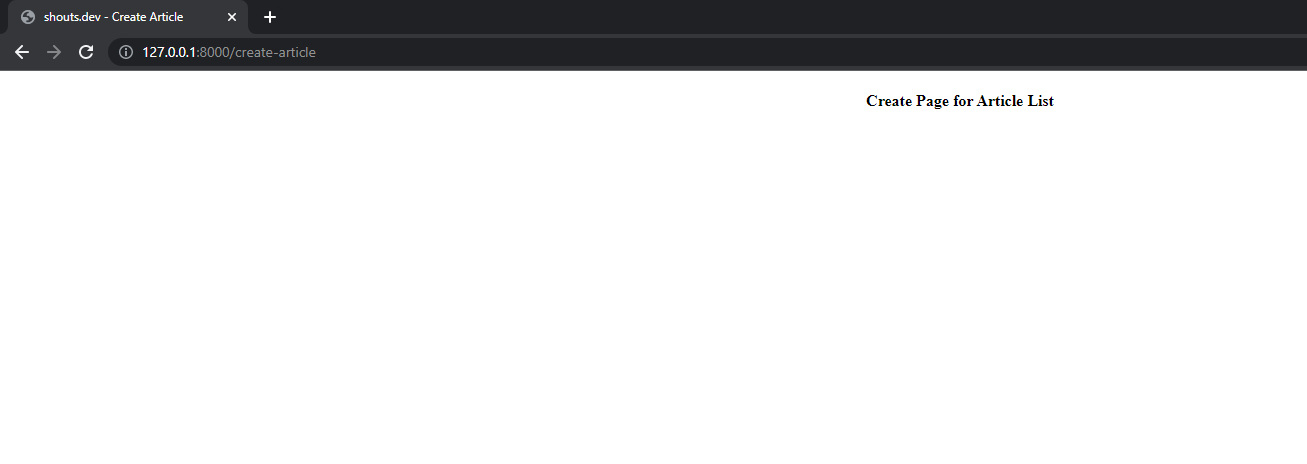
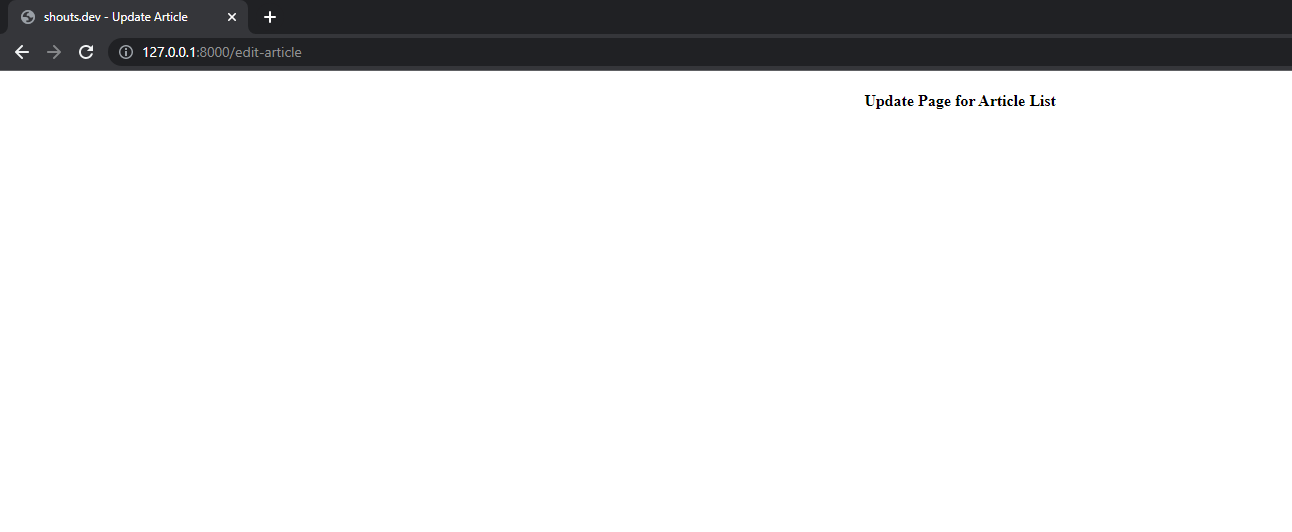
That's it for today. Hope you've enjoyed this tutorial. Catch me in the comment section if anything goes wrong/for more info. You can also download this tutorial from GitHub. Thanks for reading.