Laravel Event and Listener with Example
Hi artisans. In this article, I’m going to share how Laravel event and listener work with example. Let’s start:
Table of Contents
- Create Event
- Create Listener
- Register Event to Providers
- Create Controller and Route
- Create Email Template
- Test
Create Event
Create an event by this artisan command:
php artisan make:event OrderPlaced
The event path is app/Events/OrderPlaced.php. Open the file and paste this code:
<?php
namespace App\Events;
use Illuminate\Broadcasting\Channel;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Broadcasting\PresenceChannel;
use Illuminate\Broadcasting\PrivateChannel;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class OrderPlaced
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $user_id;
/**
* Create a new event instance.
*
* @return void
*/
public function __construct($user_id)
{
$this->user_id = $user_id;
}
/**
* Get the channels the event should broadcast on.
*
* @return \Illuminate\Broadcasting\Channel|array
*/
public function broadcastOn()
{
return new PrivateChannel('channel-name');
}
}
Create Listener
We want to send an email notification to customers if OrderPlaced event fires. Create a listener:
php artisan make:listener SendOrderEmailNotification
The listener path is app/Listeners/SendOrderEmailNotification.php. Open the file and write send email code in the handle()
function like:
<?php
namespace App\Listeners;
use App\Events\OrderPlaced;
use App\Models\User;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Support\Facades\Mail;
class SendOrderEmailNotification
{
/**
* Create the event listener.
*
* @return void
*/
public function __construct()
{
//
}
/**
* Handle the event.
*
* @param object $event
* @return void
*/
public function handle(OrderPlaced $event)
{
$user = User::find($event->user_id)->toArray();
Mail::send('emails.event_order_placed', ['user' => $user], function($message) use ($user) {
$message->to($user['email']);
$message->subject('Thanks for your order');
$message->from('[email protected]', 'Shouts.dev');
});
}
}
Register Event to Providers
Open app/Providers/EventServiceProvider.php file and update the $listen[]
with the following code:
<?php
namespace App\Providers;
use App\Events\OrderPlaced;
use App\Listeners\SendOrderEmailNotification;
use Illuminate\Auth\Events\Registered;
use Illuminate\Auth\Listeners\SendEmailVerificationNotification;
use Illuminate\Foundation\Support\Providers\EventServiceProvider as ServiceProvider;
use Illuminate\Support\Facades\Event;
class EventServiceProvider extends ServiceProvider
{
/**
* The event listener mappings for the application.
*
* @var array
*/
protected $listen = [
Registered::class => [
SendEmailVerificationNotification::class,
],
OrderPlaced::class => [
SendOrderEmailNotification::class,
],
];
/**
* Register any events for your application.
*
* @return void
*/
public function boot()
{
//
}
}
Create Controller and Route
Let’s create a controller:
php artisan make:controller EventController
Open the controller from app/Http/Controllers and pase this code:
<?php
namespace App\Http\Controllers;
use App\Events\OrderPlaced;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Event;
class EventController extends Controller
{
public function index()
{
$user_id = 1;
Event::dispatch(new OrderPlaced($user_id));
dd("Check inbox");
}
}
Now open routes/web.php and register this route:
use App\Http\Controllers\EventController;
Route::get('/event', [EventController::class, 'index']);
Create Email Template
Go to resources/views folder and create a folder named emails. Under emails folder create a file called event_order_placed.blade.php. Then open file and paste this code:
Hi {{ $user['name'] }},<br><br>
Thanks for your order. Your order will be processed soon.<br><br>
Regards,<br>
shouts.dev
Test
Before going to test, check there is at least 1 user in the database. If there is no user, then create 1 user first. You can create the user using Laravel tinker:
php artisan tinker
User::factory()->count(1)->create()
Or using Laravel eloquent:
use App\Models\User;
use Illuminate\Support\Facades\Hash;
// add user
$user = new User();
$user->name = "Md Obydullah";
$user->email = "[email protected]";
$user->password = Hash::make('123456');;
$user->save();
Lastly, to send email, you should set your SMTP credentials in .env file.
Now run this project:
php artisan serve
Now visit http://localhost:8000/event
route. Then check your inbox:
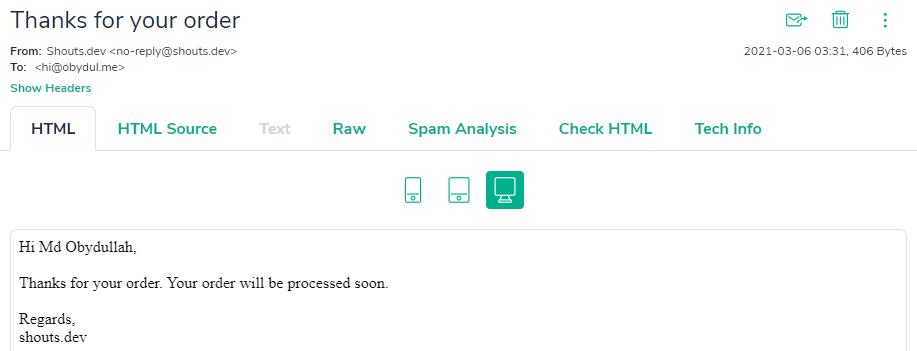
That’s all, folks. Thanks for reading.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.