Laravel 10 How to Add Input Mask using jQuery in Laravel
Hello Artisan, today I'll show you how to mask your input field using jQuery plugin. So, why do we actually need masking an input. Suppose we want an input field where user should only type date format like this dd/mm/YYYY, but you've provided a text field where user can type anything he/she want. So, for handling this situation, we can use input masking which easily can handle this type of situations.
Put the below route in the web.php file.
Route::get('/input_mask', function () {
return view('input_mask');
});
Now we'll setup our view and we'll use the input library in this blade. Where we'll setup our view file with two input field and we'll initiate with this fields with our input masks. So, let's see the below source code.
<!DOCTYPE html>
<html>
<head>
<title>How To Add Input Mask Using Jquery In Laravel 10 - shouts.dev</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.4.0/css/bootstrap.min.css"/>
</head>
<body>
<div class="container">
<h1>How To Add Input Mask Using Jquery In Laravel 10 - shouts.dev</h1>
<div class="form border p-5">
<strong>Phone Number:</strong>
<input type="text" name="phone" class="phone form-control" value="1234567890"><br>
<strong>Mobile Number:</strong>
<input type="text" name="mobile" class="mobile form-control">
</div>
</div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.inputmask/3.3.4/jquery.inputmask.bundle.min.js"></script>
<script>
$(document).ready(function(){
$('.phone').inputmask('(999)-999-9999');
$('.mobile').inputmask('(999)-999-9999');
});
</script>
</body>
</html>
And it's done. In the next step, we'll check out output.
Now it's the time to check the output. Go to localhost:8000/input_mask, and you'll find the below output.
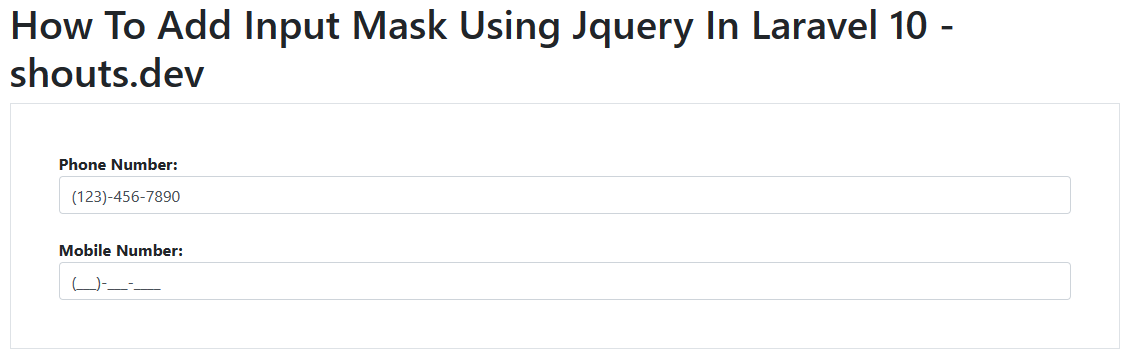
Even if you try to write something else, it'll not let you do.
That's it for today. I hope it'll be helpful in upcoming project. You can also download this source code from GitHub. Thanks for reading. ๐