Laravel Dynamic Chart with Chart.js
Hello Artisans, today we'll discuss about how to integrate dynamic charts using ChartJs. ChartJs is a javascript library which provides various kinds of charts for our website. For more info check here. So, let's see how we can implement our different types of chart in our Laravel Application without using any Laravel package.
Note: Tested on Laravel 9.11
Table of Contents
Create and Setup Controller
First we need to create a controller from where we can write our query so that we can represent our chart according the query result.
Fire the below command to create a controller
php artisan make:controller HighChartController
It'll create a controller under app\Http\Controllers called HighChartController.php. Open the file and replace with below codes.
<?php
namespace App\Http\Controllers;
use App\Models\User;
class HighChartController extends Controller
{
public function index(): \Illuminate\Contracts\View\Factory|\Illuminate\Contracts\View\View|\Illuminate\Contracts\Foundation\Application
{
$users = User::selectRaw('YEAR(created_at) as year,COUNT(*) as count')->groupBy('year')->get();
$data = [
'year' => json_encode($users->pluck('year')),
'user' => json_encode($users->pluck('count')),
];
return view('chart',$data);
}
}
Define Routes
Now we need to put the below routes in our web.php
Route::get('chart', [App\Http\Controllers\HighChartController::class, 'index']);
Create and Setup blade File
Now we need to create a blade file for viewing the chart in our browser. So, create a file under resources\views named chart.blade.php. And open the file and replace with the following codes.
<!DOCTYPE html>
<html>
<head>
<title>Laravel 9 - shouts.dev</title>
</head>
<body>
<h1>ChartJS Chart Example - shouts.dev</h1>
<canvas id="myChart" height="100px"></canvas>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script type="text/javascript">
var labels = {{ $year }};
var users = {{ $user }};
const data = {
labels: labels,
datasets: [{
label: 'My First dataset',
backgroundColor: 'rgb(255, 99, 132)',
borderColor: 'rgb(255, 99, 132)',
data: users,
}]
};
const config = {
type: 'line',
data: data,
options: {}
};
const myChart = new Chart(
document.getElementById('myChart'),
config
);
</script>
</html>
Create Dummy Records
Now we'll create some dummy records using Tinker. If you're not familiar with tinker, then I'll recommend you to follow one of my short tutorial on Tinker. Now, fire the below commands in terminal
php artisan tinker
User::factory()->count(30)->create()
Output
Now we ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000/chart, If everything goes well you'll find a below output.
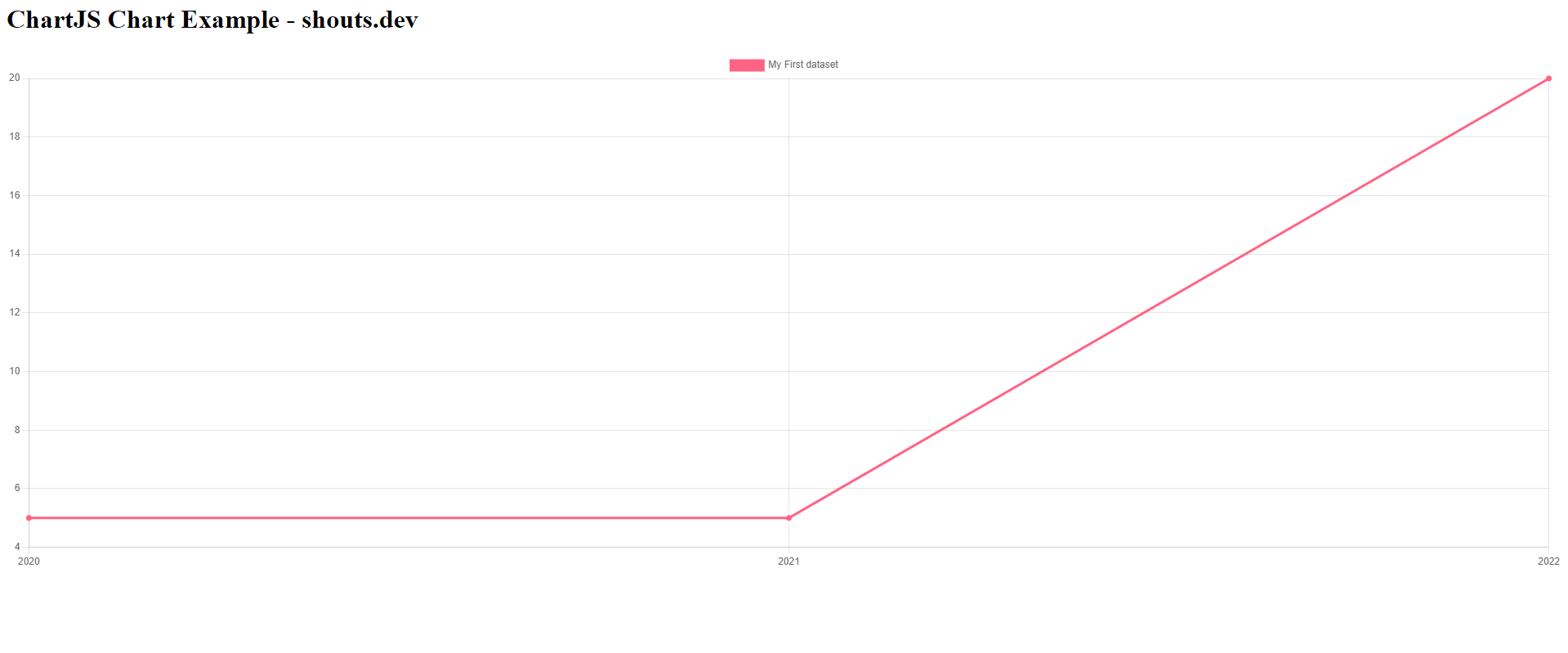
That's it for today. Hope you'll enjoy this tutorial. You can also download this tutorial from GitHub. Thanks for reading. ๐