Laravel Create and use Custom Artisan Command
Hello Artisans, today I'll talk about how you can create your own custom command in your Laravel Application. As we'll know Laravel comes with Artisan command-line interface, which makes the development life cycle very easy. We'll see how we can create some dummy users using our own created Artisan command. So let's see how we can create and use our custom artisan command in our Laravel Application.
Note: Tested on Laravel 9.2.
Table of Contents
Setup .env
Frist of all we need to ensure that our database is connected. So, insert database credentials like database name, username and password in your .env file as below.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=generate_command
DB_USERNAME=root
DB_PASSWORD=
Create and Setup Artisan Command
Now we need to create a command using the below command.
php artisan make:command createDummyUsers
It'll create a file under the app/Console/Commands/createDummyUsers.php, open the file and paste the below code
<?php
namespace App\Console\Commands;
use App\Models\User;
use Illuminate\Console\Command;
class createDummyUsers extends Command
{
protected $signature = 'create:dummy-users {number}';
protected $description = 'Command description';
public function __construct()
{
parent::__construct();
}
public function handle()
{
$usersData = $this->argument('number');
for ($i = 0; $i < $usersData; $i++) {
User::factory()->create();
}
}
}
Output
Now you are all set to go, just fire the below command in your terminal
php artisan create:dummy-users 50
Hola!!! your users are created:
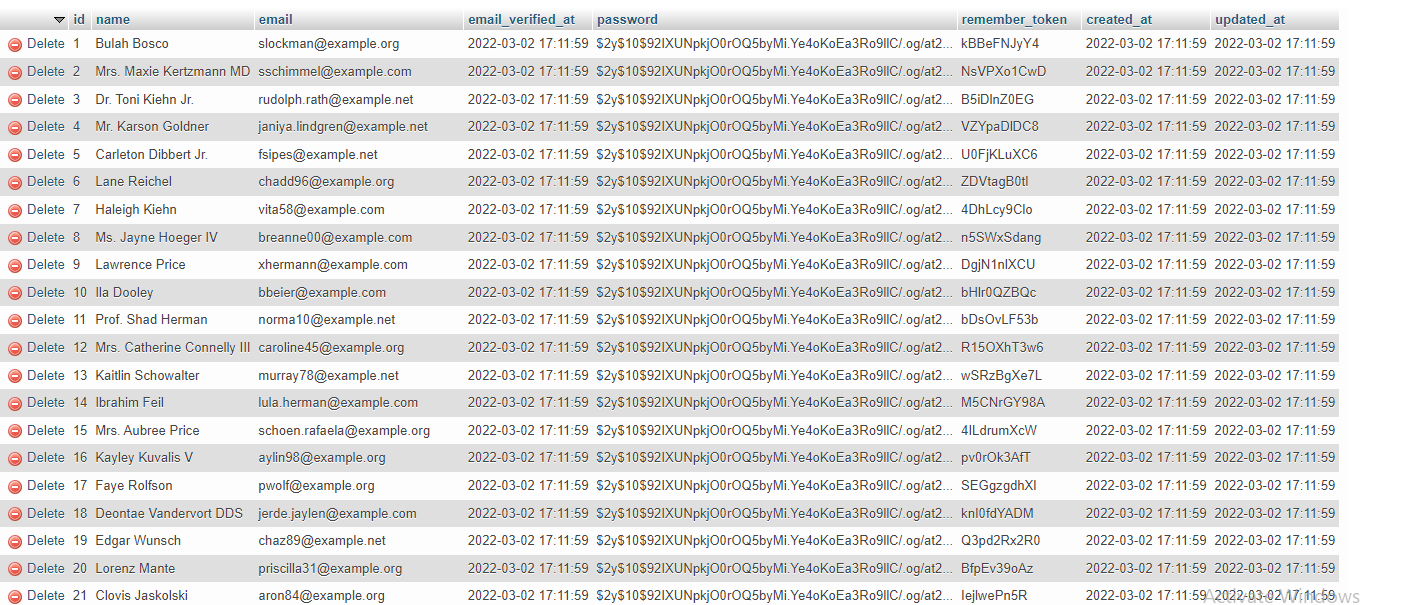
That's it for today. Hope you'll enjoy this tutorial. You can also download this tutorial from GitHub. Thank's for reading :)