How to Store Array in Database in Laravel
Hello Artisans, today I'll show you how to store array in your database column. Sometimes we may need to store array of data or json data in our column. But mysql doesn't support array to be stored. And today's tutorial I want to show you how do to that in a easier and in a Artisan way. So, no more talk let's see how we can easily store such data in our database column.
Note: Tested on Laravel 9.19.
Table of Contents
Create Model, Migration and Controller
For creating a model, migration and controller we just need to fire the below command in our terminal
php artisan make:model Article -mcr
and it'll create a model called Article.php, ArticleController.php and article_migrations. We'll work later with these files.
Setup Migration
Now we'll setup our migration file that we created on step1, here we'll create the table schema for our articles table. Let's see the below code snippet.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('articles', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('details')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('articles');
}
};
Setup Model
Now we'll setup our model file that we created on step1, here we'll add our table columns into fillable property and also cast the details column as array, so that we can store arrays of data in our database. Let's see the below code snippet.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Article extends Model
{
use HasFactory;
protected $fillable = ['title','details'];
protected $casts = ['details' => 'array'];
}
Setup Controller
Now we'll setup our controller that we created on step1, here we'll give the input as array and it'll store on detail's column. And also if we want to retrieve these column, it'll print the result in array. Let's see the below code snippet
<?php
namespace App\Http\Controllers;
use App\Models\Article;
use Illuminate\Http\Request;
class ArticleController extends Controller
{
public function create(Request $request)
{
$data = [
'title' => 'Article',
'details' => [
'category_1' => 'Laravel',
'category_2' => 'VueJs',
'category_3' => 'Mysql',
]
];
$article = Article::create($data);
dd($article->details);
}
}
Create Route
Let's put the below route in web.php
Route::resource('store-array',\App\Http\Controllers\ArticleController::class)->only('create');
Output
And finally we're ready with our setup. It's time to check our output. Now go to http://127.0.0.1:8000/store-array/create, If everything goes well (hope so) you can find a below output on.
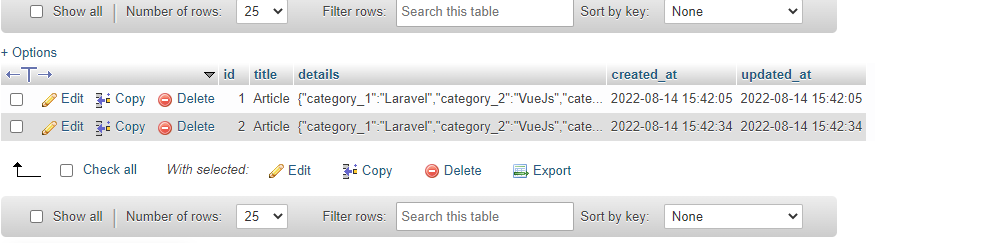
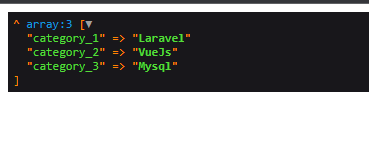
That's it for today. If you have any questions, ask me in the comment section. Hope you've enjoyed this tutorial. You can also download this tutorial from GitHub. Thanks for reading.