JavaScript get Unique/Non-Unique Values from an Array
Hi devs, today I'll show you how to filter your array for getting unique or non-unique values. We'll see two examples of that and use filter() and indexOf() methods. These methods are pretty handy for filtering and getting the index of an item into an array. For more info see the following docs for filter() and indexOf() methods. So, no more talk let's see how we can easily filter our array.
Example 1: Get Unique values from an Array
In the first example we'll see how to get unique values from an array. Let's see the below code snippet.
const arry = [1, 2, 1, 3, 4, 3, 5];
const toFindDuplicates = arry => arry.filter((item, index) => arry.indexOf(item) == index);
const duplicateElements = toFindDuplicates(arry);
console.log(duplicateElements);
What we do is here is, firstly we'll use array filter() method then in the iteration part we give an condition like the index of current iteration and the item of current iteration of the array is same or not. If the condition pass then it'll filter my data according to that. Which will give the output as below
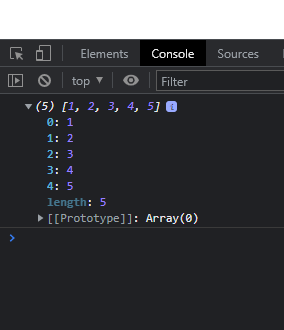
Example 2: Get Non-Unique values from an Array
In the second example we'll see how to get non-unique values from an array. In this example, we'll use same code snippet from step1, but as of our example is about to reverse of step1, so we'll reverse our example as well. Let's see the below code snippet.
const arry = [1, 2, 1, 3, 4, 3, 5];
const toFindDuplicates = arry => arry.filter((item, index) => arry.indexOf(item) !== index);
const duplicateElements = toFindDuplicates(arry);
console.log(duplicateElements);
Here we'll use the same mechanism of step1, but the difference is in this example unlike the step1, we'll check index of current iteration and the item of current iteration of the array is not same. If the condition pass then it'll filter my data according to that. Which will give the output as below
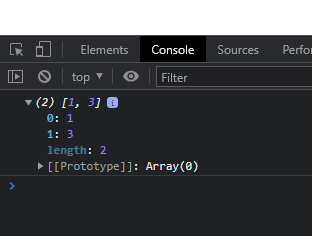
That's it for today. I hope you've enjoyed this tutorial. Thanks for reading. ๐