How to Auto Select Country Using jQuery IP Lookup
Hello Artisan, today I'll show you how to validate your phone numbers using jQuery. We'll use international Telephone Input jQuery library. This package provides blur event which let us easily validate our number. And we'll also use some third party API's so that we can find our IP and by finding our IP we can find the country code. After finding the country code we'll the use that intel-tel-input library to auto select our country. So, let's dive into the topic and how we can easily use this library to get our desired output.
At first, we'll write some HTML codes so that we can include our required stylesheets and CDN files. So, let's put the below HTML code in your HTML file.
<html lang="en">
<head>
<title>How To Validate International Phone Number Using jQuery - shouts.dev</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/build/css/intlTelInput.css">
</head>
<body>
<div class="col-md-6 offset-md-2">
<div class="container mt-5">
<div class="card">
<div class="card-header">
<strong>How To Validate International Phone Number Using jQuery - shouts.dev</strong>
</div>
<div class="card-body">
<h6 class="card-title">Phone Number:</h6>
<input id="phone" type="tel">
<span id="valid-msg" class="hide">โ Valid</span>
<span id="error-msg" class="hide"></span>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/build/js/intlTelInput.min.js"></script>
</body>
</html>
Then we'll put some CSS into it. So, that we can show response in a better way. Let's see below code.
.hide {
display: none;
}
#valid-msg {
color: #00c900;
}
#error-msg {
color: red;
}
Here we'll just telling some rules so that when we get and valid number then we'll show it with #00c900 color and whenever we get an error by entering wrong number, then we'll show it with red color.
Ok now we'll add some jQuery code. See the below source code.
const input = document.querySelector("#phone");
const errorMsg = document.querySelector("#error-msg");
const validMsg = document.querySelector("#valid-msg");
// here, the index maps to the error code returned from getValidationError - see readme
const errorMap = ["Invalid number", "Invalid country code", "Too short", "Too long", "Invalid number"];
// initialise plugin
const iti = window.intlTelInput(input, {
initialCountry: "auto",
geoIpLookup: callback => {
fetch("https://api.iplocation.net/?cmd=get-ip")
.then(res => res.json())
.then(data => fetch("https://api.iplocation.net?ip="+data.ip)
.then(res => res.json())
.then(data => callback(data.country_code2))
)
.catch(() => callback("us"));
},
utilsScript: "https://cdn.jsdelivr.net/npm/[email protected]/build/js/utils.js"
});
const reset = () => {
input.classList.remove("error");
errorMsg.innerHTML = "";
errorMsg.classList.add("hide");
validMsg.classList.add("hide");
};
// on blur: validate
input.addEventListener('blur', () => {
reset();
if (input.value.trim()) {
if (iti.isValidNumber()) {
validMsg.classList.remove("hide");
} else {
input.classList.add("error");
const errorCode = iti.getValidationError();
errorMsg.innerHTML = errorMap[errorCode];
errorMsg.classList.remove("hide");
}
}
});
// on keyup / change flag: reset
input.addEventListener('change', reset);
input.addEventListener('keyup', reset);
- First of all, we'll find the IP and after finding the IP we'll search our Country code. After finding the country code we'll set the default country with callback() method.
- It selects the necessary elements from the HTML using the document.querySelector method and assigns them to variables: input, errorMsg, and validMsg. These variables represent the input field, error message element, and valid message element, respectively.
- An array named errorMap is defined, which maps error codes returned from the getValidationError function to corresponding error messages.
- The intlTelInput library is initialized by calling window.intlTelInput with the input element as the first argument and an options object as the second argument. The options object includes a utilsScript property that specifies the URL of the utils.js script required by the library.
- The reset function is defined, which removes error classes from the input field, clears the error message, and hides the error and valid messages.
- An event listener is added to the blur event of the input element. When the input field loses focus, the reset function is called, and if the input value is not empty, the phone number is validated using the isValidNumber function provided by the intlTelInput library.
- If the phone number is valid, the valid message is displayed by removing the "hide" class from the validMsg element. Otherwise, the input field is marked with an error class, the error code is obtained using the getValidationError function, and the corresponding error message is displayed in the errorMsg element.
- Two additional event listeners are added to the change and keyup events of the input element. These event listeners call the reset function whenever the input value or the selected country flag changes.
Overall, this code sets up an input field for phone numbers and provides real-time validation using the intlTelInput library. It displays error messages for invalid phone numbers and a success message for valid phone numbers.
So, finally our whole code looks like below.
<html lang="en">
<head>
<title>Auto Select Country Using jQuery - shouts.dev</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/build/css/intlTelInput.css">
<style>
.hide {
display: none;
}
#valid-msg {
color: #00c900;
}
</style>
</head>
<body>
<div class="col-md-6 offset-md-2">
<div class="container mt-5">
<div class="card">
<div class="card-header">
<strong>Auto Select Country Using jQuery - shouts.dev</strong>
</div>
<div class="card-body">
<h6 class="card-title">Phone Number:</h6>
<input id="phone" type="tel">
<span id="valid-msg" class="hide">โ Valid</span>
<span id="error-msg" class="hide"></span>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/build/js/intlTelInput.min.js"></script>
<script>
const input = document.querySelector("#phone");
const errorMsg = document.querySelector("#error-msg");
const validMsg = document.querySelector("#valid-msg");
// here, the index maps to the error code returned from getValidationError - see readme
const errorMap = ["Invalid number", "Invalid country code", "Too short", "Too long", "Invalid number"];
// initialise plugin
const iti = window.intlTelInput(input, {
initialCountry: "auto",
geoIpLookup: callback => {
fetch("https://api.iplocation.net/?cmd=get-ip")
.then(res => res.json())
.then(data => fetch("https://api.iplocation.net?ip="+data.ip)
.then(res => res.json())
.then(data => callback(data.country_code2))
)
.catch(() => callback("us"));
},
utilsScript: "https://cdn.jsdelivr.net/npm/[email protected]/build/js/utils.js"
});
const reset = () => {
input.classList.remove("error");
errorMsg.innerHTML = "";
errorMsg.classList.add("hide");
validMsg.classList.add("hide");
};
// on blur: validate
input.addEventListener('blur', () => {
reset();
if (input.value.trim()) {
if (iti.isValidNumber()) {
validMsg.classList.remove("hide");
} else {
input.classList.add("error");
const errorCode = iti.getValidationError();
errorMsg.innerHTML = errorMap[errorCode];
errorMsg.classList.remove("hide");
}
}
});
// on keyup / change flag: reset
input.addEventListener('change', reset);
input.addEventListener('keyup', reset);
</script>
</html>
So, if we open our HTML file in our browser then we'll show the below output.
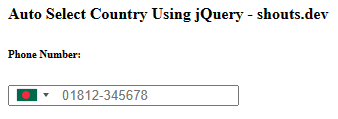
That's it for today. I hope it'll be helpful in upcoming project. Thanks for reading. ๐