How to Map a Nested Array in React
A nested array is an array that contains other arrays within it, similar to a table or 2D grid. There are two ways to map a nested array: using a combination of the flat and map array functions, or using a map within a map. The choice of which method to use depends on the specific situation, and I will provide an example for each. If the data is only a 1D array, then a regular map can be used, which is explained in this article.
Let's see how can we map a nested array:
By using the flat function, we can transform our 2-dimensional array into a 1-dimensional array, which we can then map over to generate our components. To illustrate this, consider the example of a basic students list which is sorted by First Character in a group.
const students = [
['Ahmad', 'Amin', 'Amir'],
['Barira', 'Basir','Burhan'],
['Cemal', 'Caleb'],
];
And here’s the code for our component:
import React from 'react';
function StudentList({ student }) {
return <li className="bg-neutral-800 p-2 font-normal shadow">{student}</li>;
}
export function App() {
const students = [
['Ahmad', 'Amin', 'Amir'],
['Barira', 'Basir','Burhan'],
['Cemal', 'Caleb'],
];
return (
<div className='App'>
<h1>Student's List</h1>
{students.flat().map((student, index) => (
<StudentList key={index} student={student} />
))}
</div>
);
}
And here is our rendered output:
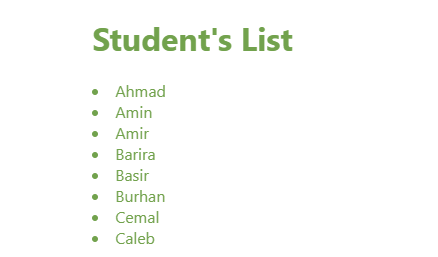
When you need to display a list of items, sometimes it makes sense to group related items together. However, to simply display the items, you can just list them all together without any grouping. This method is useful when the grouping of data is not important. Although this approach leads to shorter code, it also results in a simpler data structure. An example of this can be seen in an Instagram feed where posts from different users are combined and displayed in a single feed.
In many situations, it may be desirable to preserve the structure of nested data. For instance, you may want to maintain the grouping of students by their respective characters in different list. In such cases, it is important to refactor the data while retaining its structure.
import React from 'react';
function StudentList({ student }) {
return <li className="bg-neutral-800 p-2 font-normal shadow">{student}</li>;
}
function StudentGroup({students}) {
return (
<ul>
{students.map((student, idx) => (
<StudentList key={idx} student={student} />
))}
</ul>
)
}
export function App() {
const students = [
['Ahmad', 'Amin', 'Amir'],
['Barira', 'Basir','Burhan'],
['Cemal', 'Caleb'],
];
return (
<div className='App'>
<h1>Grouped Student's List</h1>
{students.map((studentGroup, index) => (
<StudentGroup key={index} students={studentGroup} />
))}
</div>
);
}
To maintain a tidy code, I have divided it into three components: one for a single students group, one for a row in the list, and one for the entire list. I believe this approach is effective for situations like this, where you construct a component by assembling simpler, less intricate building blocks. This method simplifies the problem from having to map within a map to just mapping over an array in two scenarios.
And here is our rendered output:
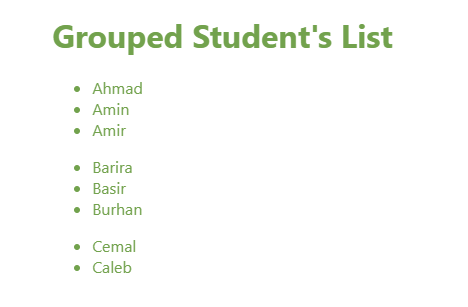
Instead of being in one big list, the rows are now kept in separate lists.
If you were able to successfully map through a nested array using one of these examples, or encountered any difficulties, please feel free to leave a comment below!
Thanks for reading.