Concurrency in Computer Science with Example
Concurrency in computer science refers to the concept of executing multiple tasks or processes simultaneously, allowing for better resource utilization and improved program performance. It's a fundamental concept in modern computing, especially in multi-core processors and distributed systems.
Here's a simple example to illustrate concurrency using a common scenario: a restaurant with a chef and a waiter.
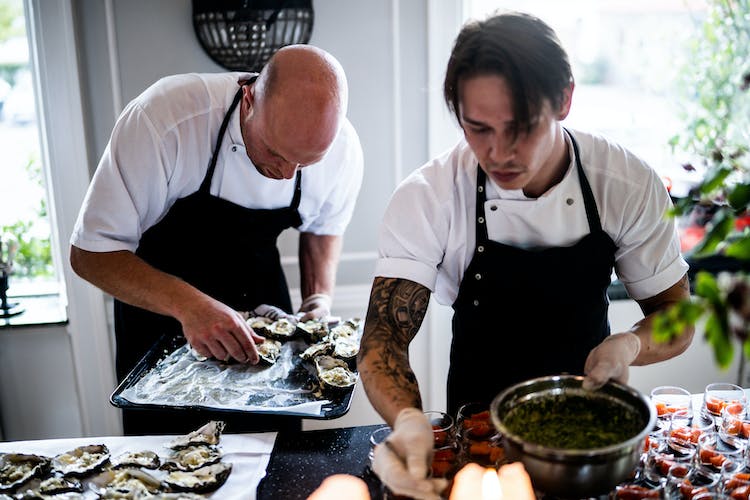
Imagine you're running a restaurant, and you have a chef who prepares dishes and a waiter who serves them to customers. In a sequential (non-concurrent) scenario, the process might look like this:
- Chef starts cooking a meal.
- Chef finishes cooking and hands the meal to the waiter.
- Waiter serves the meal to the customer.
- Waiter waits for the customer to finish eating.
- Waiter returns to the chef to request another meal
In this sequential process, the waiter has to wait for the chef to finish cooking before serving the meal, and the chef has to wait for the waiter to request another meal before starting to cook again. This can lead to inefficiency and wasted time.
Now, let's introduce concurrency to make the process more efficient:
- Chef starts cooking a meal.
- Waiter takes another customer's order and brings it to the chef.
- Chef continues cooking the first meal while starting to prepare the second.
- Chef finishes the first meal and hands it to the waiter.
- Waiter serves the first meal to the customer.
- Waiter waits for the first customer to finish eating while the chef finishes the second meal.
- When the first customer finishes, the waiter takes their plate and brings it to the kitchen.
- The waiter serves the second meal to the second customer.
- This process repeats, allowing the chef and waiter to work concurrently.
In this concurrent process, the chef and waiter can work on multiple orders simultaneously, which makes better use of their time and reduces wait times for customers. This is similar to how concurrency works in computer programs. Multiple tasks or processes run simultaneously, and they can overlap or run in parallel, improving overall system efficiency and performance.
Concurrency in Laravel can be achieved using various techniques and tools. One common approach is to use Laravel's built-in support for queues and jobs. Queues allow you to handle time-consuming tasks concurrently, which can greatly improve the performance and responsiveness of our application.
Here's an example of how to implement concurrency in Laravel using queues and jobs:
Create a new job by running the following Artisan command:
php artisan make:job ProcessTask
In the app/Jobs/ProcessTask.php
file, define the logic that we want to run concurrently. For example:
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
class ProcessTask implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $data;
public function __construct($data)
{
$this->data = $data;
}
public function handle()
{
// Simulate a time-consuming task
sleep(5);
// Process $this->data
// You can log or store the result here
}
}
In this example, the ProcessTask
job simulates a time-consuming task using sleep(5)
.
In our Laravel application code, we can dispatch this job whenever we want to execute it concurrently. For example, in a controller method:
use App\Jobs\ProcessTask;
public function index()
{
// Data you want to process concurrently
$data = ['item1', 'item2', 'item3'];
foreach ($data as $item) {
ProcessTask::dispatch($item);
}
return "Tasks dispatched for processing.";
}
This code will dispatch the ProcessTask
job for each item in the $data
array. Laravel will handle the concurrency for you by pushing these jobs into a queue.
With this setup, Laravel will handle the concurrency of processing the tasks in the background using the queue worker. This can greatly improve the performance and responsiveness of Laravel application when dealing with time-consuming tasks.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.