How to Merge Multiple PDF Files in Single File
Hello Artisan, today I'll show you how to merge multiple pdf file in a single pdf file. We'll use a package which will easier our job. Let's see how we can use it in our application.
Note: Tested on Laravel 8.75.
Table of Contents
Install Package
To install the package we just need to fire the below command
composer require setasign/fpdf
composer require setasign/fpdi
And that's it, we've successfully install the package.
Create and Setup Controller
Now, we need to do some work in our controller which will process the pdf's file merge it into one. So, first create a controller using below command
Now, we need to do some work in our controller which will process the pdf's file merge it into one. So, first create a controller using below command
php artisan make:controller PdfController
It'll create controller called PdfController.php. Open the file and paste the following code
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use setasign\Fpdi\Fpdi;
class PdfController extends Controller
{
public function index()
{
return view('index');
}
public function store(Request $request)
{
$request->validate([
'filenames' => 'required',
'filenames.*' => 'mimes:pdf'
]);
$files = [];
foreach ($request->file('filenames') as $key => $value) {
$files[] =$value->getPathName();
}
$fpdi = new FPDI;
foreach ($files as $file) {
$filename = $file;
$count = $fpdi->setSourceFile($filename);
for ($i = 1; $i <= $count; $i++) {
$template = $fpdi->importPage($i);
$size = $fpdi->getTemplateSize($template);
$fpdi->AddPage($size['orientation'], array($size['width'], $size['height']));
$fpdi->useTemplate($template);
}
}
$fpdi->Output(public_path('/merged-pdf.pdf'), 'F');
return back()->with('message' , 'File Merged Successfully');
}
}
Create Route
Put the below route in your web.php
Route::get('file',[\App\Http\Controllers\PdfController::class,'index'])->name('file');
Route::post('file',[\App\Http\Controllers\PdfController::class,'store'])->name('file');
Setup Blade Template
Now we need setup our blade file where we can upload pdf files and download the merged one. So create index.blade.php under resources/views and paste the following code.
<html>
<head>
<title></title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<!-- Popper JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<!-- Latest compiled JavaScript -->
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">
<h2>Laravel 8 Merge Multiple Pdf into One</h2>
</div>
<div class="card-body">
@if (session()->has('message'))
<div class="alert alert-success">
{{ session('message') }}
</div>
@endif
<form method="post" action="{{route('file')}}" enctype="multipart/form-data">
@csrf
<input type="file" name="filenames[]" class="myfrm form-control" multiple>
<div class="text-center">
<button type="submit" class="btn btn-success" style="margin-top:10px">Submit</button>
</div>
</form>
</div>
</div>
@if(file_exists(public_path('merged-pdf.pdf')))
<table class="table table-bordered">
<thead>
<tr>
<th>#</th>
<th>File</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr>
<th>1</th>
<th>merged-pdf.pdf</th>
<th><a href="{{ asset('merged-pdf.pdf') }}" class="btn btn-primary" download="merged_pdf.pdf">Download</a></th>
</tr>
</tbody>
</table>
@endif
</div>
</div>
</div>
</body>
</html>
Output
Now we've completed our tutorial. It's time to show the result. Go to http://127.0.0.1:8000/file and you'll find the below output
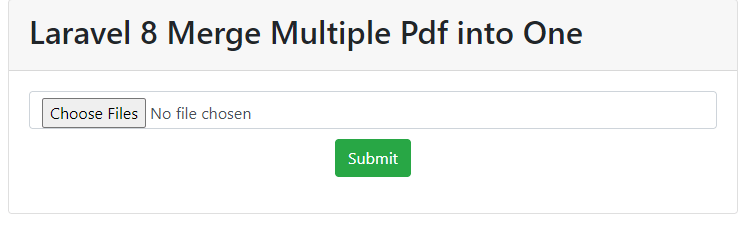
Now upload 2/3 pdf files then you can see the below output with download option of your merged pdf file.
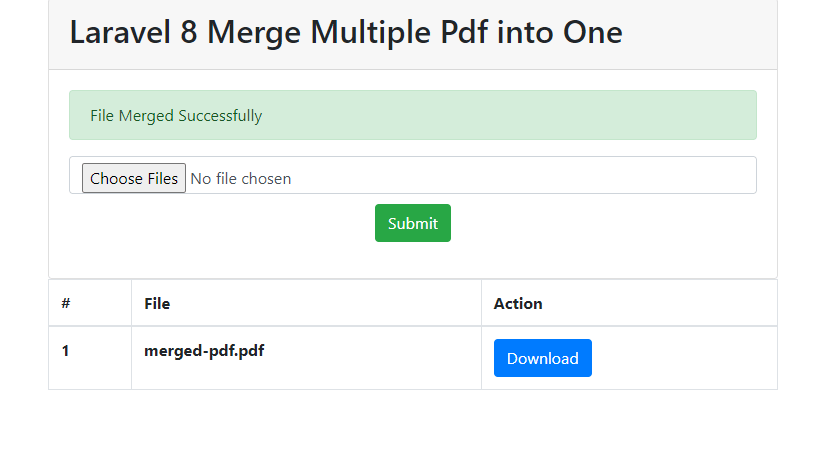
That's it for today. Hope you'll enjoy through this tutorial. You can also also download this from GitHub. Thank's for reading :)