How to Get Online Users in Laravel
Hello Artisans, today, I would like to show you how to get online users in laravel. We will look at example of laravel get all logged in users. We will learn in laravel how to check if a user is online or not. Let's get started with to check if user is online or not.
In this tutorial, I will show you simplest way of how to get all online users with last seen time in laravel.
Here, we will add new column "last_seen" in users table and create new middleware for web that will check if user login then it will update last_seen time and add key for online in Cache, so we can check user online or not using that. So, no more talk, let's see how we can get our online users in our Laravel Application.
Note: Tested on Laravel 9.18
Table of Contents
- Add New Column to users table
- Create Middleware
- Create and Setup Controller
- Define Route
- Create and Setup Blade File
- Output
Add new column to users migration file
Here, we will create new migration for adding "last_seen" column:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->timestamp('last_seen')->nullable();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('user', function (Blueprint $table) {
$table->dropColumn('last_seen');
});
}
};
Now, we will migrate our migration file:
php artisan migrate
Create Middleware
now, we will create OnlineUser for update last seen time and add online status to cache, let's run below command:
php artisan make:middleware OnlineUser
Now, update middleware code as bellow:
<?php
namespace App\Http\Middleware;
use App\Models\User;
use Closure;
use Illuminate\Http\Request;
use Auth;
use Cache;
class OnlineUser
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure(\Illuminate\Http\Request): (\Illuminate\Http\Response|\Illuminate\Http\RedirectResponse) $next
* @return \Illuminate\Http\Response|\Illuminate\Http\RedirectResponse
*/
public function handle(Request $request, Closure $next)
{
if (Auth::check()) {
$expiresAt = now()->addMinutes(2); /* keep online status for 1 min */
Cache::put('user-is-online-' . Auth::user()->id, true, $expiresAt);
/* last seen */
User::where('id', Auth::user()->id)->update(['last_seen' => now()]);
}
return $next($request);
}
}
Now register, this middleware to kernel file:
<?php
namespace App\Http;
use App\Http\Middleware\OnlineUser;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel
{
............
protected $middlewareGroups = [
'web' => [
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
// \Illuminate\Session\Middleware\AuthenticateSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
\Illuminate\Routing\Middleware\SubstituteBindings::class,
OnlineUser::class,
],
'api' => [
// \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
];
..........
}
Create and Setup Controller
Now create UserController and update controller like this:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index(): \Illuminate\Contracts\View\Factory|\Illuminate\Contracts\View\View|\Illuminate\Contracts\Foundation\Application
{
$users = User::whereNotNull('last_seen')
->orderBy('last_seen', 'DESC')
->paginate(5);
return view('users', compact('users'));
}
}
Define route
Now, we will define our route
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('online-user', [App\Http\Controllers\UserController::class, 'index']);
Create and Setup Blade File
<!DOCTYPE html>
<html>
<head>
<title>Laravel Online User Showing Example - shouts.dev</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" integrity="sha512-SfTiTlX6kk+qitfevl/7LibUOeJWlt9rbyDn92a1DqWOw9vWG2MFoays0sgObmWazO5BQPiFucnnEAjpAB+/Sw==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<style type="text/css" media="screen">
body{
background-color: #f7fcff;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 offset-2">
<div class="card mt-5">
<div class="card-header">
<div class="row">
<div class="col-md-11">
<h4>Laravel Online User Showing Example - shouts.dev</h4>
</div>
</div>
</div>
<div class="card-body">
<table class="table table-bordered">
<thead>
<tr>
<th>No</th>
<th>Name</th>
<th>Email</th>
<th>Last Seen</th>
<th>Status</th>
</tr>
</thead>
<tbody>
@foreach($users as $key => $user)
<tr>
<td>{{ ++$key }}</td>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
<td>
{{ Carbon\Carbon::parse($user->last_seen)->diffForHumans() }}
</td>
<td>
@if(Cache::has('user-is-online-' . $user->id))
<span class="text-success">Online</span>
@else
<span class="text-secondary">Offline</span>
@endif
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Output
Now we are ready to run our example and login with user. so run below command so quick run:
php artisan serve
Now, login to your system and check on http://127.0.0.1:8000/online-user and see the result:
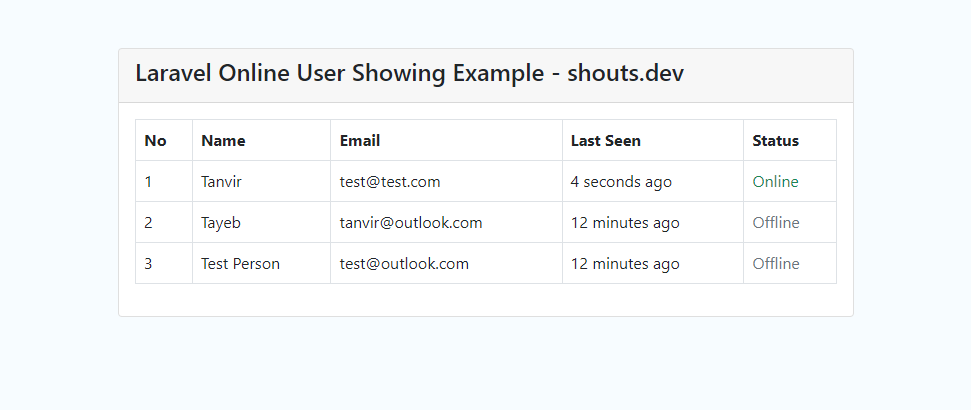
That's it for today. Hope you'll enjoy this tutorial. Thanks for reading.๐