How to Generate Multi Unique Slug in Laravel
Hello Artisans, today I'll talk about about how you can generate the the multiple unique slug from the product name /title. A slug is the URL or most human readable unique identifier which helps us to quickly identify the web page or web resource. So, let's see how we can implement slug generator in our Laravel application.
Note: Tested on Laravel 9.2.
Table of Contents
- Generate Model, Migration and Controller
- Setup Model and Migration
- Setup Controller
- Define Routes
- Output
Generate Model, Migration and Controller
Fire the below command to create Product Model, ProductController and products migration
php artisan make:model Product -mcr
Setup Model and Migration
Now open the products migration which we create at #step1 and paste the following code
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('slug');
$table->string('description')->nullable();
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('products');
}
};
Now run the below command to migrate your products table
php artisan migrate
Now open the Product.php, which we create on #step1 and paste the following code
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Str;
class Product extends Model
{
use HasFactory;
protected $fillable = ['name', 'slug', 'description'];
}
Setup Controller
Now open the ProductController.php, which we create on #step1 and paste the following code. Here we just create a new product and to test if our unique slug is generated or not.
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use Illuminate\Http\Request;
use Illuminate\Support\Str;
class ProductController extends Controller
{
public function store(Request $request)
{
$slug = Str::slug("Iphone 13 Max Pro");
$max = Product::whereName("Iphone 13 Max Pro")->latest('id')->first();
if ($max) {
$slug = $slug . '-' . $max->id + 1;
}
$product = Product::create([
"name" => "Iphone 13 Max Pro",
"slug" => $slug,
"description" => "This is just for laravel slug example"
]);
dd($product);
}
}
Define Routes
Now put the below route in your web.php
Route::get('product/store',[\App\Http\Controllers\ProductController::class,'store'])->name('product.store');
Output
Now we're done with our coding, now we need to check the output.
If we visit this URL http://127.0.0.1:8000/product/store multiple time, we can see a below output
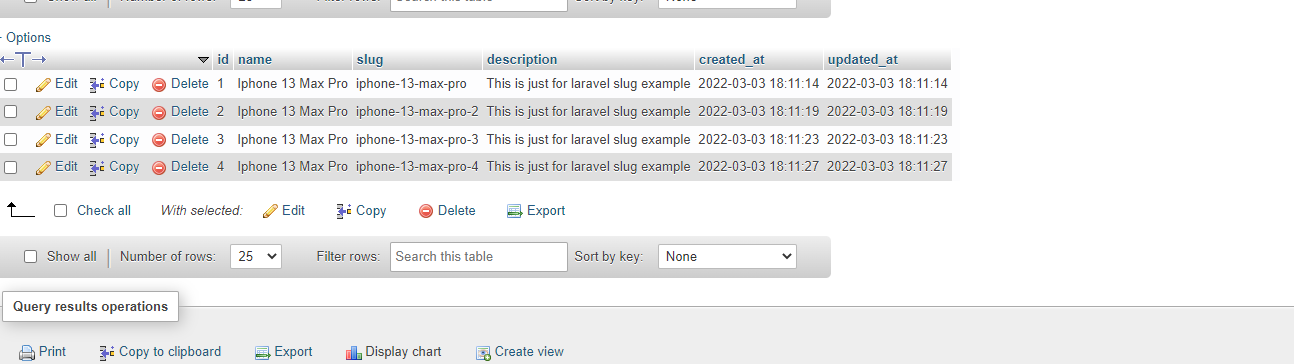
That's it for today. Hope you'll enjoy through this tutorial. You can also also download this tutorial from GitHub. Thanks for reading :)