Generate QR Code in Laravel
We know that QR Code is the trademark for a type of matrix barcode (or two-dimensional barcode). QR Code can hold 3248 bits or 406 bytes. In this tutorial, we are going to generate the QR Code.
Table of Contents
We need to follow these steps:
- Install Laravel
- Install Package and Configure
- Basic Usage
- Create Route
- Generate in Blade File
- Advanced Usage
Step 1 : Install Laravel
Go to your project folder (for xampp, go to htdocs folder) and write this command in your terminal to create a project.
composer create-project --prefer-dist laravel/laravel laravelproject
Step 2 : Install Package and Configure
We will use a package named ‘simplesoftwareio/simple-qrcode‘. Let’s use composer to install this package:
composer require simplesoftwareio/simple-qrcode
Now we have to add a service provider and aliase to the configuration file (optional for Laravel 5.5 and upper). Open config/app.php file and put the code like below:
'providers' => [
....
SimpleSoftwareIO\QrCode\QrCodeServiceProvider::class,
],
'aliases' => [
....
'QrCode' => SimpleSoftwareIO\QrCode\Facades\QrCode::class,
],
Step 3 : Basic Usage
We have successfully installed QR Code in our Laravel application. Let’s see some basic usage. The basic syntax is:
QrCode::size(100)->generate('MyNotePaper');
Size: We can set the size of the QR code image.
QrCode::size(300)->generate('MyNotePaper');
Color: We can also set background color.
QrCode::size(250)->backgroundColor(255,255,204)->generate('MyNotePaper');
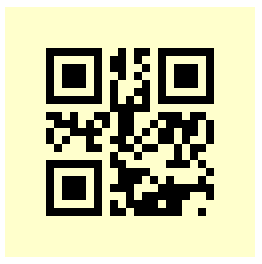
Step 4 : Create Route
In this step, we will create a ‘qrcode’ route. Copy and paste this code in routes/web.php file:
<?php
Route::get('qrcode', function () {
return QrCode::size(250)
->backgroundColor(255, 255, 204)
->generate('MyNotePaper');
});
Now run your application and visit the URL to see the QR Code. ?
// run application
php artisan serve
// visit the route
http://localhost:8000/qrcode
Step 5 : Generate in Blade File
We can easily generate QR Code in the blade file. The format is:
{!! QrCode::generate('MyNotePaper'); !!}
Let’s see an example:
Create a route:
Route::get('qrcode_blade', function () {
return view('qr_code');
});
Now create a blade file in resources folder named ‘qr_code_blade.php’.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<title>Laravel QR Code Example</title>
</head>
<body>
<div class="text-center" style="margin-top: 50px;">
<h3>Laravel QR Code Example</h3>
{!! QrCode::size(300)->generate('MyNotePaper'); !!}
<p>MyNotePaper</p>
</div>
</body>
</html>
Let’s see the output by visiting the route from browser:
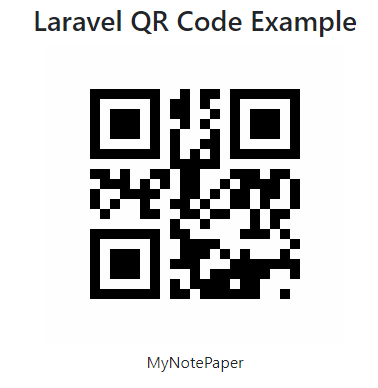
Step 6 : Advanced Usage
View QR Code without Saving: You can display a PNG image without saving the file by providing a raw string and encoding with base64_encode
.
<img src="data:image/png;base64, {!! base64_encode(QrCode::format('png')->size(100)->generate('Make me into an QrCode!')) !!} ">
Format: Normally generate(); function return svg image. There are some formats too.
QrCode::format('png'); //Returns a PNG image
QrCode::format('eps'); //Returns a EPS image
QrCode::format('svg'); //Returns a SVG image
Encoding: We can set encoding of the character too:
QrCode::encoding('UTF-8')->generate('QR code with special symbols ♠♥!!');
Margin: Set margin by this way:
QrCode::margin(10)->generate('MyNotePaper');
Bitcoin: With the help of this helper function we can send payment when scanned.
QrCode::BTC($address, $amount);
//Sends a 0.334BTC payment to the address
QrCode::BTC('bitcoin address', 0.334);
//Sends a 0.334BTC payment to the address with some optional arguments
QrCode::size(500)->BTC('address', 0.0034, [
'label' => 'my label',
'message' => 'my message',
'returnAddress' => 'https://www.returnaddress.com'
]);
Text Message: We can write sms in QR code.
QrCode::SMS($phoneNumber, $message);
//Creates a text message with the number filled in.
QrCode::SMS('555-555-5555');
//Creates a text message with the number and message filled in.
QrCode::SMS('555-555-5555', 'Body of the message');
Mobile Number: Dial mobile number from scanned QR code.
QrCode::phoneNumber($phoneNumber);
QrCode::phoneNumber('555-555-5555');
QrCode::phoneNumber('1-800-Laravel');
Email: We can also automatically fill email
, subject
and body
when scanned QR Code:
QrCode::email($to, $subject, $body);
//Fills in the to address
QrCode::email('[email protected]');
//Fills in the to address, subject, and body of an e-mail.
QrCode::email('[email protected]', 'This is the subject.', 'This is the message body.');
//Fills in just the subject and body of an e-mail.
QrCode::email(null, 'This is the subject.', 'This is the message body.');
Geo Location: Pass longitude and latitude through the QR code:
QrCode::geo($latitude, $longitude);
QrCode::geo(37.822214, -122.481769);
Connect to WiFi: Easily connect to WiFi from scanned QR code.
QrCode::wiFi([
'encryption' => 'WPA/WEP',
'ssid' => 'SSID of the network',
'password' => 'Password of the network',
'hidden' => 'Whether the network is a hidden SSID or not.'
]);
//Connects to an open WiFi network.
QrCode::wiFi([
'ssid' => 'Network Name',
]);
//Connects to an open, hidden WiFi network.
QrCode::wiFi([
'ssid' => 'Network Name',
'hidden' => 'true'
]);
//Connects to an secured, WiFi network.
QrCode::wiFi([
'ssid' => 'Network Name',
'encryption' => 'WPA',
'password' => 'myPassword'
]);
Image Placement: We can set an image inside the QR code.
$image = QrCode::format('png')
->merge('folder/image.png', 0.5, true)
->size(500)->errorCorrection('H')
->generate('MyNotePaper');
return response($image)->header('Content-type','image/png');
With the help of this package, it’s very easy to generate QR Code. I hope this article will help you.
Md Obydullah
Software Engineer | Ethical Hacker & Cybersecurity...
Md Obydullah is a software engineer and full stack developer specialist at Laravel, Django, Vue.js, Node.js, Android, Linux Server, and Ethichal Hacking.